Converting a NodeList to an array with vanilla JavaScript

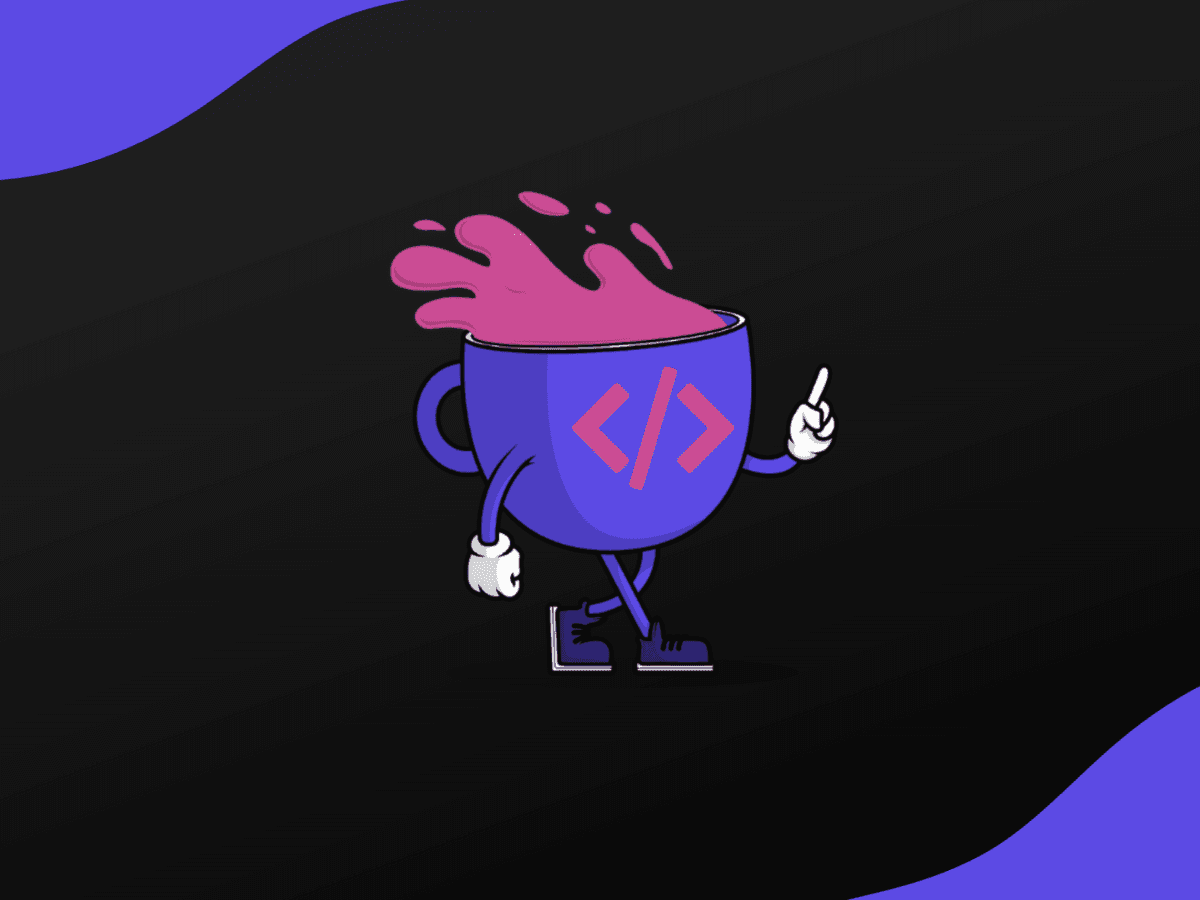
- Array.from()
- Spread Operator
- Array.prototype.slice.call()
In JavaScript, NodeList and Array are two different types of objects. A NodeList is a collection of nodes extracted from a document. An Array, on the other hand, is a global object that is used in the construction of arrays.
1var nodeList = document.querySelectorAll("div");
2console.log(Array.isArray(nodeList)); // Will log `false`
Today, we will explore different ways to convert a NodeList into an Array.
Array.from()
The Array.from()
method creates a new Array instance from an iterable object. In our case, the iterable is the NodeList.
1var nodeList = document.querySelectorAll("div");
2var array = Array.from(nodeList);
3console.log(Array.isArray(array)); // returns true
This method is part of the ES6 specification and is widely supported in modern browsers.
Spread Operator
The spread operator (...
) allows iterables such as NodeList to be expanded into places where zero or more arguments or elements are expected.
1var nodeList = document.querySelectorAll("div");
2var array = [...nodeList];
3console.log(Array.isArray(array)); // returns true
This is also an ES6 feature.
Array.prototype.slice.call()
Before ES6, the common way to convert a NodeList to an array was by using Array.prototype.slice.call()
.
1var nodeList = document.querySelectorAll("div");
2var array = Array.prototype.slice.call(nodeList);
3console.log(Array.isArray(array)); // returns true
This method works in all browsers including IE6.
To loop through a NodeList, you can use the forEach
method after converting it to an array.
1var nodeList = document.querySelectorAll("div");
2var array = Array.from(nodeList);
3array.forEach(function (element) {
4 console.log(element);
5});
Remember, a NodeList is not an array. They are different types of objects. But with these methods, you can easily convert a NodeList to an array in JavaScript.
You can learn more about JavaScript and its features in our JavaScript course.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
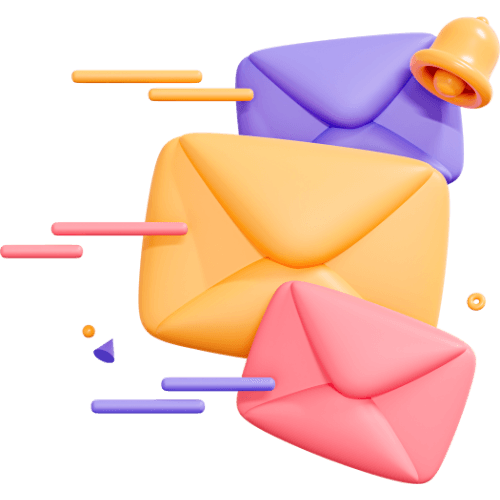
Related articles
9 Articles
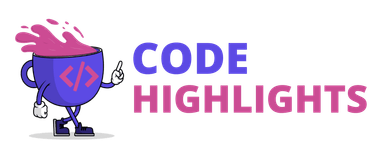
Copyright © Code Highlights 2025.