JavaScript not equal and Comparison Operators Explained

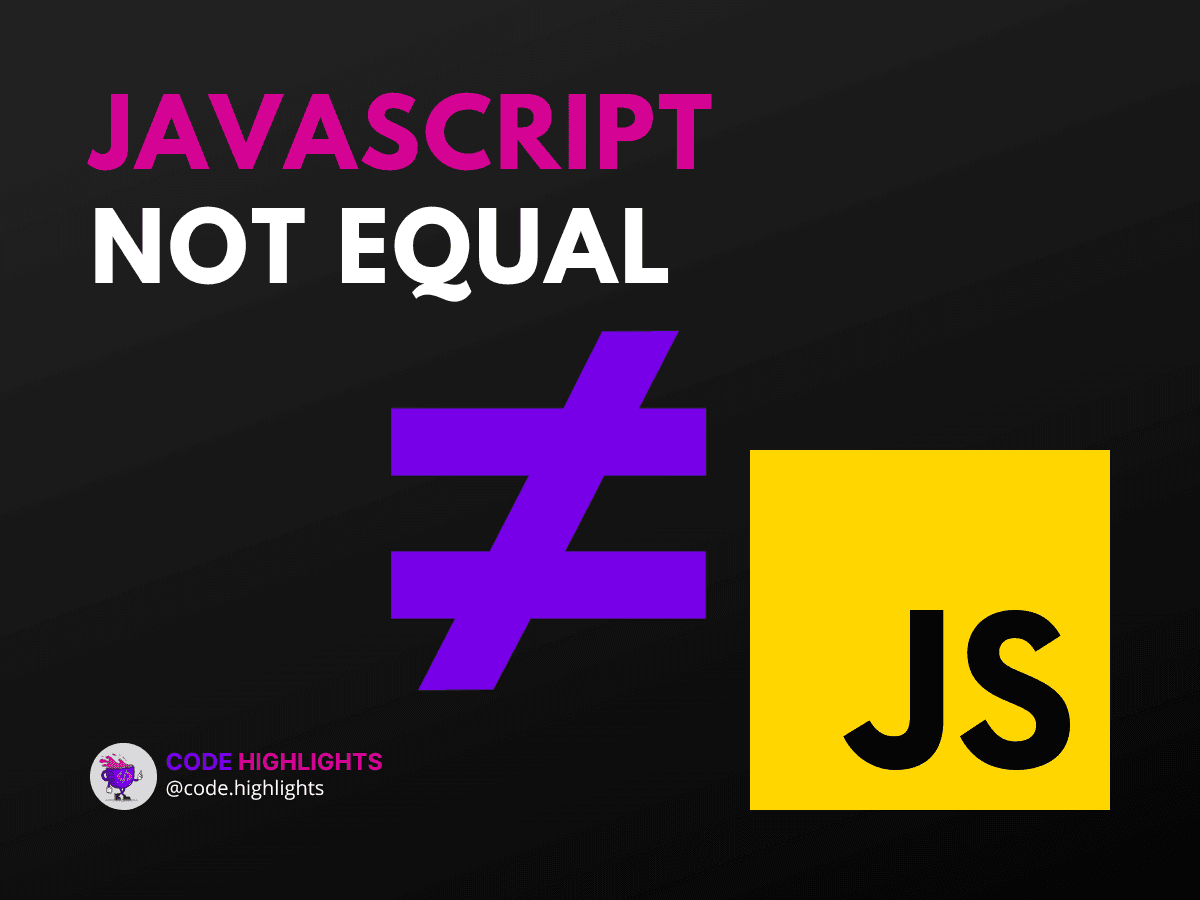
- Understanding != and !== in JavaScript
- The Pitfalls of Using == in JavaScript
- What Does != Mean in Code?
- Choosing Between == and === in JavaScript
When diving into the world of JavaScript, understanding comparison operators is like learning the rules of the road before you start driving. It's essential! In this tutorial, we're going to focus on the javascript not equal
operator and its siblings in the comparison family. We'll explore the differences between !=
and !==
, why ==
might not always be your best choice, and when it's appropriate to use ==
and ===
. Let's buckle up and get ready for a ride through the logic lanes of JavaScript!
Understanding !=
and !==
in JavaScript
In JavaScript, !=
is known as the "not equal" or "inequality" operator. It checks whether two values are not equal and returns true
if they are indeed not equal. Here's a simple example:
But wait, there's more! JavaScript also offers a stricter version called the "strict not equal" operator, !==
. This operator not only checks if the values are not equal but also if they are of different types:
Even though apples
and oranges
have the same value numerically, they are of different types (string vs. number), hence not strictly equal.
The Pitfalls of Using ==
in JavaScript
You may wonder, why not to use ==
in JavaScript? The ==
operator is the "equal" operator that checks if two values are equal after performing type coercion if necessary. This can lead to unexpected results:
Here, '3'
(a string) is considered equal to 3
(a number) because JavaScript converts them to a common type before comparison. However, this can cause confusion and bugs in your code.
What Does !=
Mean in Code?
Simply put, !=
means "not equal to" in JavaScript. It checks if the operands on either side are different after converting them to a common type. For example:
Since '3' is not equal to 4, the output is true
.
Choosing Between ==
and ===
in JavaScript
When to use ==
and ===
in JavaScript is a crucial decision. Use ==
if you need type coercion and are certain it won't lead to unintended consequences. However, for a safer and more predictable comparison, ===
, the strict equality operator, is generally recommended. It ensures both value and type are the same:
The first comparison returns true
because both operands are of the same type and value. The second comparison returns false
because, although their values are similar, their types are not.
In conclusion, understanding and using the correct comparison operators like !=
and !==
can help you write more reliable and bug-free JavaScript code. For those looking to deepen their JavaScript knowledge, consider exploring further with Learn JavaScript or broadening your web development skills at Introduction to Web Development.
Remember, practice makes perfect. So, try out these operators in your code, and soon you'll be adept at navigating the nuances of JavaScript comparisons. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
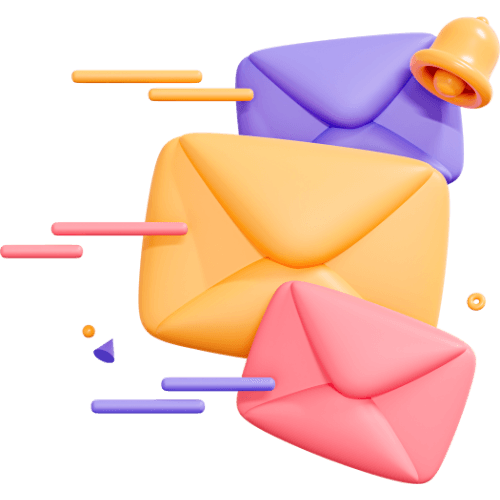
Related articles
9 Articles
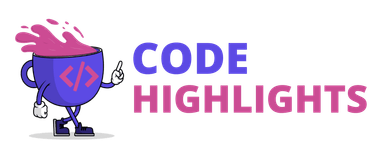
Copyright © Code Highlights 2025.