How to Print JavaScript Objects for Clear Debugging

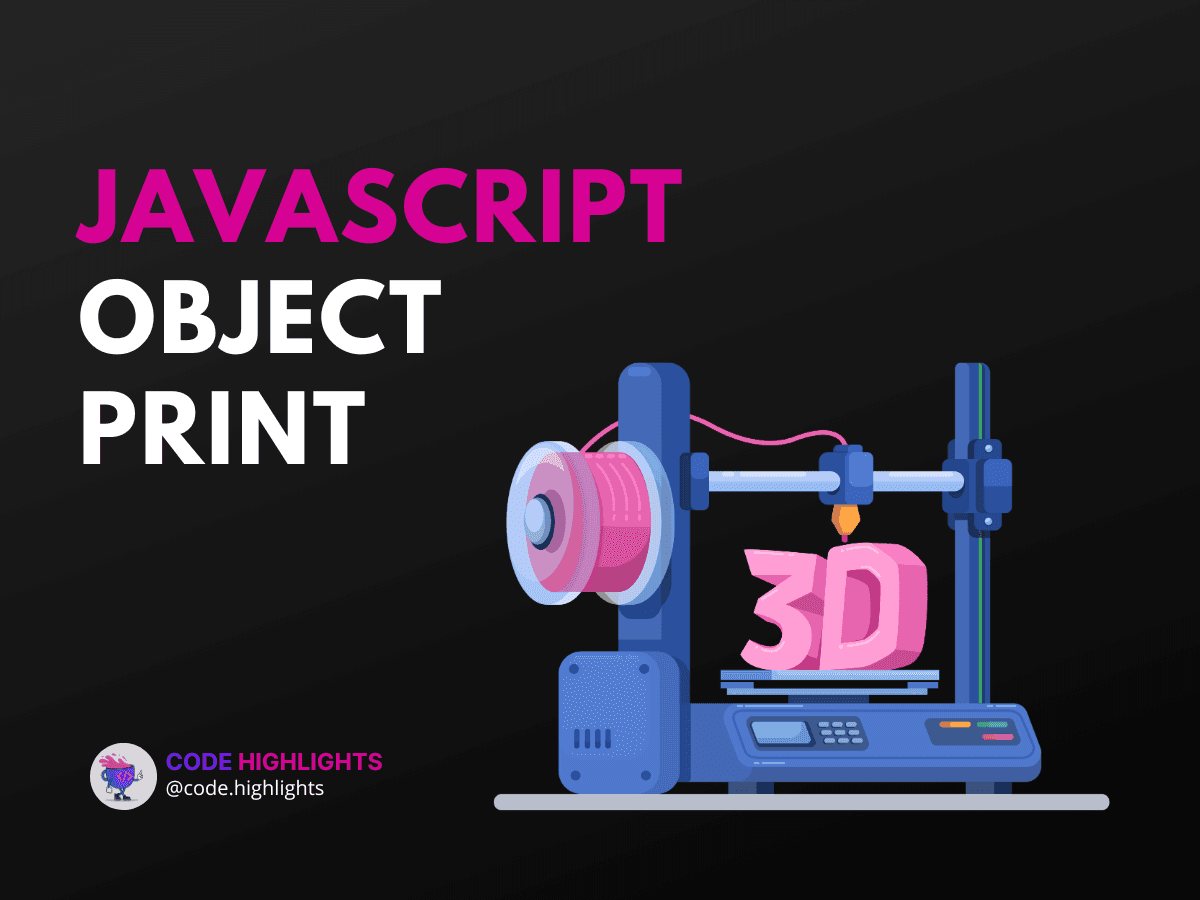
- Understanding JavaScript Object Printing
- Syntax
- Variations
- Example 1: Basic Object Printing
- Using console.log()
- Example 2: Printing as a String
- Using JSON.stringify()
- Example 3: Pretty Print JSON
- Formatting Output
- Example 4: Inspecting Nested Objects
- Accessing Inner Properties
- Example 5: Looping Through Object Properties
- Using for...in Loop
- Browser Compatibility
- Key Takeaways
Debugging is a crucial part of programming. When working with JavaScript, understanding the contents of an object can help you identify issues in your code. In this tutorial, we will explore various methods to print JavaScript objects, making it easier to see what’s inside them. This knowledge is vital for developers who want to debug efficiently and improve their coding skills.
Here’s a quick example to get us started:
This simple code snippet prints the person
object to the console, showing its properties. Let’s dive deeper into the syntax and methods available for printing JavaScript objects.
Understanding JavaScript Object Printing
To print JavaScript objects, we usually use the console.log()
function. This method displays the object in the console, which is helpful for debugging. Here’s how it works:
Syntax
- Parameters: The parameter is the object you want to print.
- Return Value: This function does not return a value; it simply outputs the object to the console.
Variations
You can also use JSON.stringify()
to convert an object into a string format before printing it. Here’s how:
This method is useful when you want to see the object as a string.
Example 1: Basic Object Printing
Using console.log()
This code prints the car
object directly to the console. It shows all properties and their values.
Example 2: Printing as a String
Using JSON.stringify()
In this case, the book
object is converted to a string before printing. This method is particularly helpful when you want a clean output.
Example 3: Pretty Print JSON
Formatting Output
1const user = { username: "john_doe", email: "john@example.com" };
2console.log(JSON.stringify(user, null, 2));
By adding null
and 2
as parameters, we format the output to be more readable. This prints the user
object in a structured way, making it easier to read.
Example 4: Inspecting Nested Objects
Accessing Inner Properties
1const student = {
2 name: "Emma",
3 grades: { math: 90, science: 85 }
4};
5console.log(student.grades);
Here, we print only the grades
property of the student
object. This approach helps focus on specific parts of an object.
Example 5: Looping Through Object Properties
Using for...in
Loop
1const animal = { type: "Dog", breed: "Beagle", age: 3 };
2
3for (let key in animal) {
4 console.log(key + ": " + animal[key]);
5}
This example loops through each property of the animal
object and prints them one by one. It’s useful for displaying all properties dynamically.
Browser Compatibility
Most modern browsers, including Chrome, Firefox, and Edge, support the console.log()
function and JSON.stringify()
. However, always check compatibility if you're working with older browsers.
Key Takeaways
In this tutorial, we explored how to print JavaScript objects effectively. We covered various methods like console.log()
and JSON.stringify()
, along with examples demonstrating their usage. Understanding how to print objects is essential for debugging and improving your coding skills. For more information on JavaScript fundamentals, consider checking out our JavaScript courses.
If you're interested in web development, you might also want to explore our HTML Fundamentals Course and CSS Introduction. These resources can provide a solid foundation for your programming journey.
For additional reading on debugging techniques, you can find helpful insights from MDN Web Docs and JavaScript.info.
By mastering these techniques, you’ll make your debugging process smoother and more effective!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
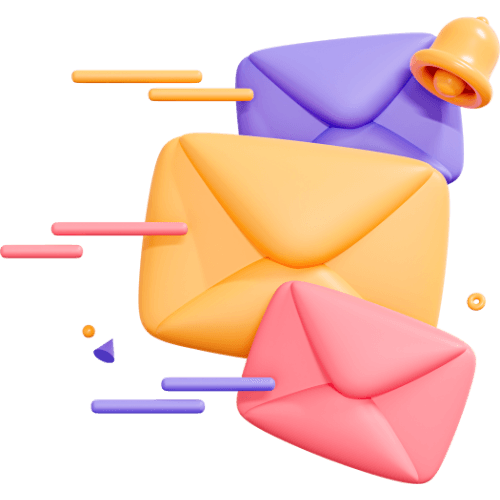
Related articles
9 Articles
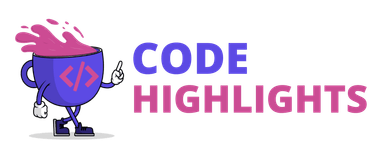
Copyright © Code Highlights 2025.