JavaScript Optional Parameters: What You Must Know

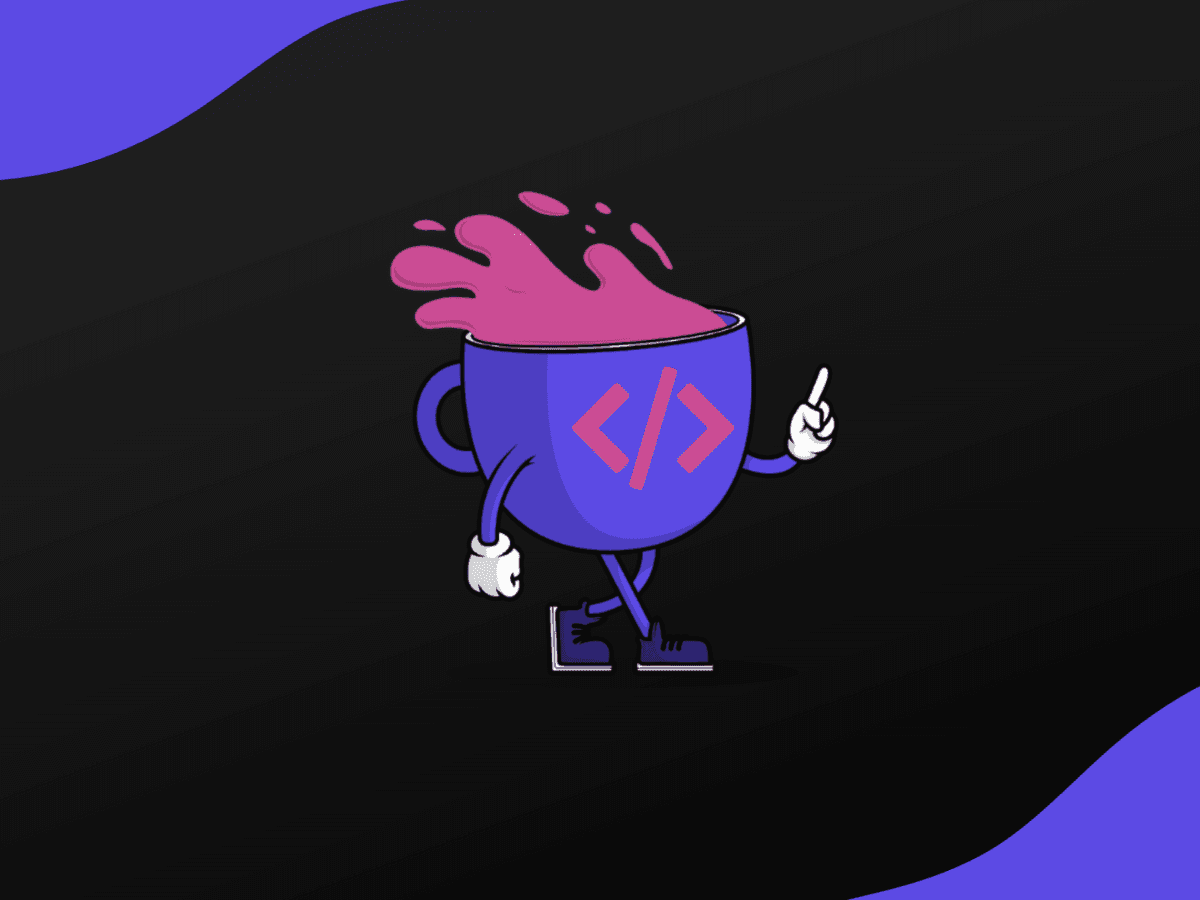
- Using undefined to Skip Optional Parameters
- Making Parameters Mandatory
- Further Learning
In JavaScript, a function parameter is defined as optional if it is not necessary to provide a value for it when the function is called. This feature can be quite useful when you want to make your function more flexible and easier to use.
The name
parameter in the greet()
function is optional. If no argument is passed when the function is called, it will default to "User". This is known as specifying an optional parameter in JavaScript.
Using undefined
to Skip Optional Parameters
It's possible to skip optional parameters in JavaScript by passing undefined
in their place. This way, you can provide values for later parameters without specifying earlier optional ones.
1function displayInfo(name = "John", age = 25, country = "USA") {
2 console.log(name + " is " + age + " years old and lives in " + country);
3}
4displayInfo(undefined, 30); // Outputs: John is 30 years old and lives in USA
In this example, we skipped the name
parameter by passing undefined
, and specified a new value for the age
parameter.
Making Parameters Mandatory
JavaScript doesn't have a built-in way to make parameters mandatory. However, you can achieve this by throwing an error if no argument is provided.
1function mandatoryParam() {
2 throw new Error('Missing parameter');
3}
4function add(a = mandatoryParam(), b = mandatoryParam()) {
5 return a + b;
6}
7add(1, 2); // returns 3
8add(1); // throws an error
In this example, if either a
or b
is not provided when add()
is called, the mandatoryParam()
function will be invoked, throwing an error.
Further Learning
To learn more about JavaScript functions and parameters, check out our JavaScript course. If you're new to coding, our Introduction to Web Development course is a great place to start.
For additional resources, Mozilla's JavaScript Guide is an excellent reference. You might also find JavaScript.info helpful for understanding more complex JavaScript concepts.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
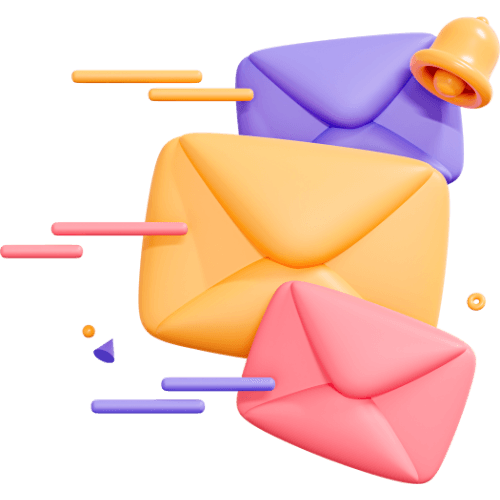
Related articles
9 Articles
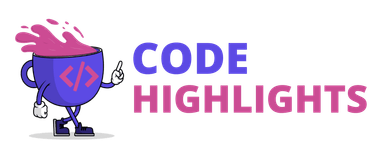
Copyright © Code Highlights 2025.