7 Surprising Facts About JavaScript Pass by Reference

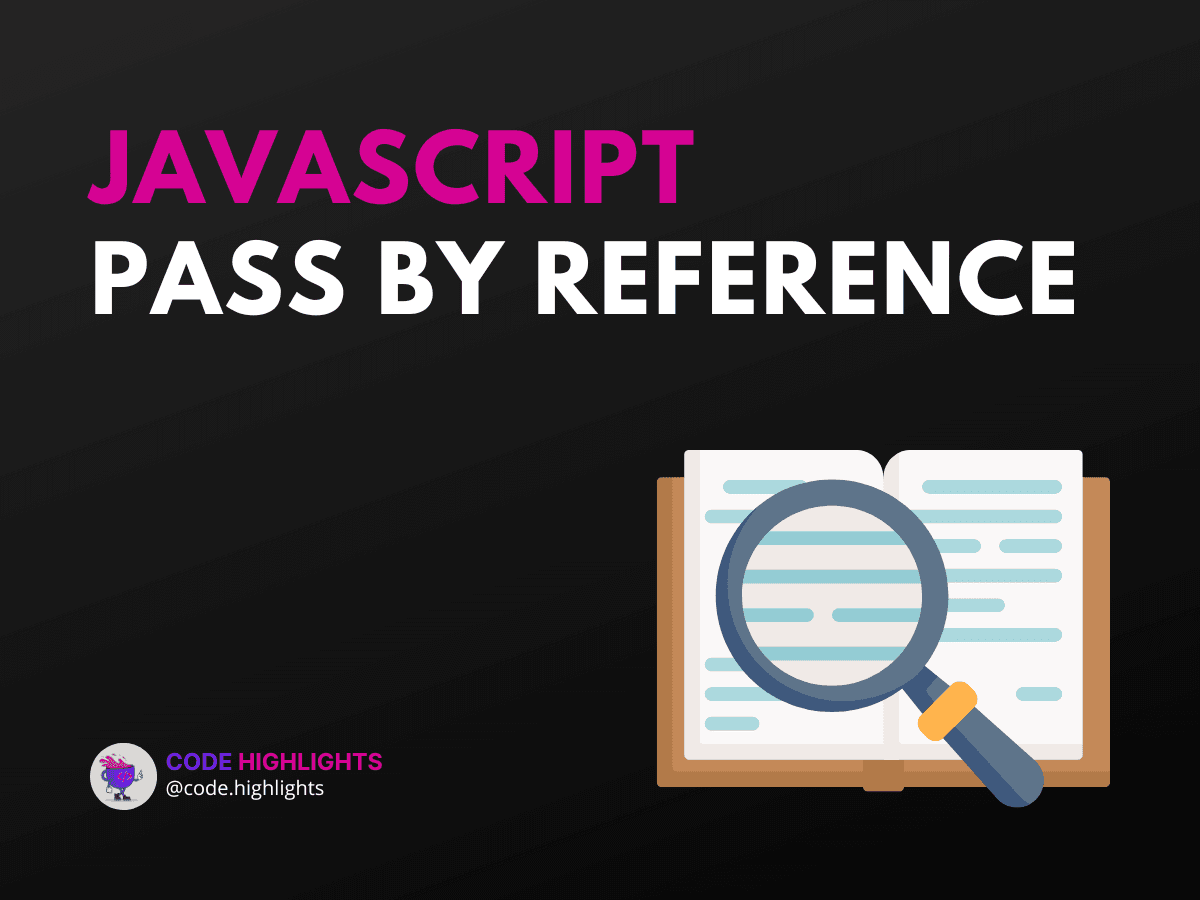
- Introduction to Pass by Reference
- Understanding the Syntax
- Code Snippet for Syntax Variation
- Fact 1: Pass by Value vs. Pass by Reference
- Example of Pass by Value
- Fact 2: Does JavaScript Always Pass by Value?
- Fact 3: What Does It Mean to Pass by Reference?
- Fact 4: Can You Pass a Function by Reference?
- Example of Passing a Function by Reference
- Fact 5: Mutability of Objects
- Example of Object Mutation
- Fact 6: Scope and Reference
- Example of Scope
- Fact 7: Compatibility with Browsers
- Summary
JavaScript is a powerful programming language that allows developers to create dynamic web applications. One of its intriguing features is how it handles data, particularly in terms of passing variables. Understanding JavaScript pass by reference can greatly enhance your coding skills and help you write more efficient code. In this tutorial, we will explore seven surprising facts about passing by reference in JavaScript, including code examples and practical applications.
Introduction to Pass by Reference
Before diving into the facts, let’s take a look at a simple code example that demonstrates passing by reference. Here’s a quick snippet:
1let obj = { name: "Alice" };
2let reference = obj;
3
4reference.name = "Bob";
5console.log(obj.name); // Outputs: Bob
In this example, when we change the name
property of reference
, it also changes obj
because both variables point to the same object in memory.
Understanding the Syntax
In JavaScript, when you pass an object or an array to a function, you are passing a reference to that object or array, not the actual value. Here's a breakdown of how this works:
- Parameters: The variables that are passed into the function.
- Return Values: The value that the function sends back after execution.
- Variations: This behavior applies to objects and arrays but not to primitive types like numbers or strings.
Code Snippet for Syntax Variation
1function modifyArray(arr) {
2 arr.push(4);
3}
4
5let numbers = [1, 2, 3];
6modifyArray(numbers);
7console.log(numbers); // Outputs: [1, 2, 3, 4]
In this case, the array numbers
is modified because it was passed by reference.
Fact 1: Pass by Value vs. Pass by Reference
A common question is, “What is the difference between pass by value and pass by reference in JavaScript?” When you pass a primitive type (like a number), it is passed by value. This means that changes to the parameter do not affect the original variable.
Example of Pass by Value
1function changeValue(num) {
2 num = 10;
3}
4
5let value = 5;
6changeValue(value);
7console.log(value); // Outputs: 5
Here, value
remains unchanged because numbers are passed by value.
Fact 2: Does JavaScript Always Pass by Value?
You might wonder, “Does JavaScript always pass by value?” The answer is yes, but it behaves differently for objects and arrays. While primitives are passed by value, objects and arrays are passed by reference.
Fact 3: What Does It Mean to Pass by Reference?
To “pass by reference” means that you are giving a function access to the original object or array rather than a copy. This allows the function to modify the original data directly.
Fact 4: Can You Pass a Function by Reference?
Yes, functions in JavaScript are first-class citizens, meaning you can pass them as arguments. When you pass a function to another function, you’re passing a reference to that function.
Example of Passing a Function by Reference
1function greet() {
2 console.log("Hello!");
3}
4
5function callFunction(fn) {
6 fn();
7}
8
9callFunction(greet); // Outputs: Hello!
In this example, greet
is passed by reference to callFunction
.
Fact 5: Mutability of Objects
When you pass an object to a function, it can be mutated. This means you can change its properties without creating a new object.
Example of Object Mutation
1function updatePerson(person) {
2 person.age++;
3}
4
5let person = { name: "John", age: 30 };
6updatePerson(person);
7console.log(person.age); // Outputs: 31
This illustrates how objects can be modified through references.
Fact 6: Scope and Reference
Understanding scope is crucial when dealing with references. If you declare a variable inside a function, it will not affect the outer variable, even if they share the same name.
Example of Scope
1let x = 10;
2
3function testScope() {
4 let x = 20; // This x is different
5 console.log(x);
6}
7
8testScope(); // Outputs: 20
9console.log(x); // Outputs: 10
Fact 7: Compatibility with Browsers
The concept of passing by reference is well-supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. This makes it a reliable feature for web development.
Summary
In this tutorial, we explored JavaScript pass by reference and uncovered seven surprising facts. We learned about the differences between passing by value and passing by reference, how objects and arrays behave, and the implications of mutability and scope. Understanding these concepts is essential for effective programming in JavaScript.
For those looking to deepen their knowledge, consider checking out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. Additionally, you can explore the broader field of web development.
By mastering these principles, you’ll be better equipped to handle complex data structures and enhance your coding skills in JavaScript.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
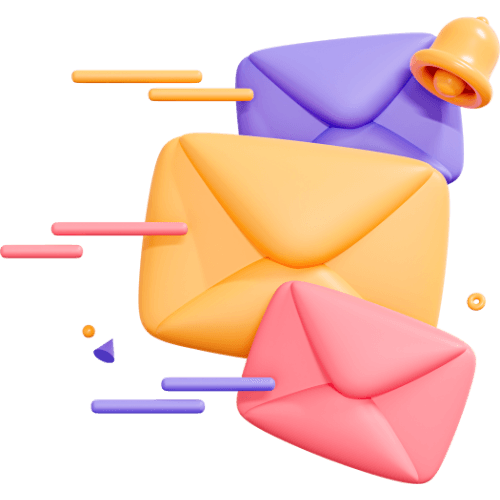
Related articles
9 Articles
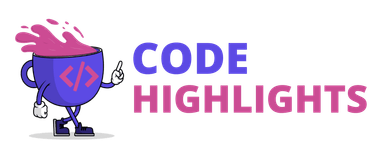
Copyright © Code Highlights 2025.