5 Powerful JavaScript Pop Techniques for 2024

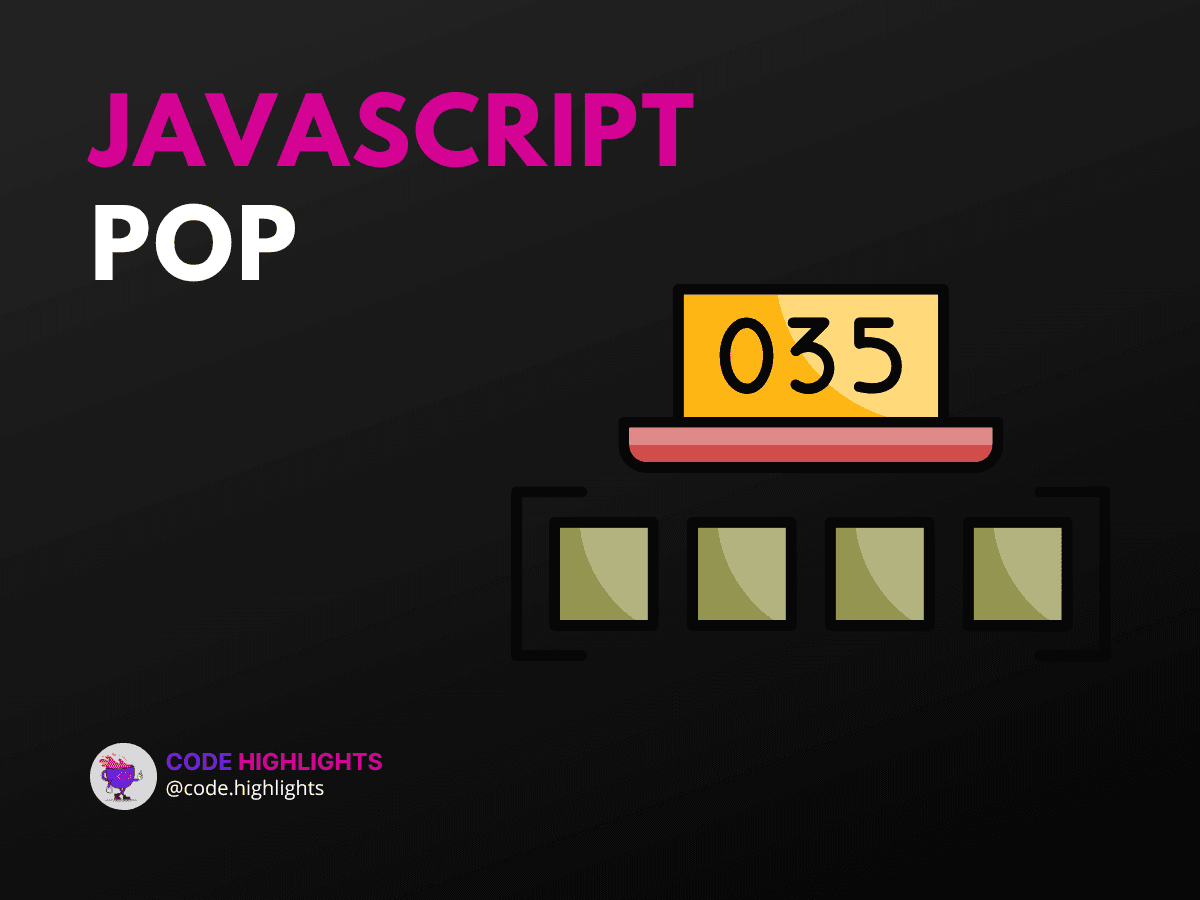
- Introduction to pop()
- Syntax of pop()
- Technique 1: Basic Array Manipulation
- Technique 2: Using pop() in Stack Operations
- Technique 3: Combining pop() with push()
- Technique 4: Using pop() in Real-time Applications
- Technique 5: Handling Empty Arrays
- Compatibility with Major Web Browsers
- Summary
JavaScript is a versatile language, and one of its most useful methods is pop()
. This method is essential for anyone looking to manipulate arrays effectively. In this tutorial, we'll dive into five powerful techniques for using pop()
in JavaScript, providing practical examples and detailed explanations.
Introduction to pop()
The pop()
method is used to remove the last element from an array and returns that element. This method changes the length of the array. Here's a quick example to get you started:
1let fruits = ["Apple", "Banana", "Cherry"];
2let lastFruit = fruits.pop();
3console.log(lastFruit); // Outputs: Cherry
4console.log(fruits); // Outputs: ["Apple", "Banana"]
Syntax of pop()
The syntax for pop()
is straightforward:
- Parameters: The
pop()
method does not take any parameters. - Return Value: It returns the removed element from the array. If the array is empty, it returns
undefined
.
Technique 1: Basic Array Manipulation
Using pop()
for basic array manipulation is common. It helps in removing the last item from an array, which can be useful in various scenarios.
Technique 2: Using pop()
in Stack Operations
In computer science, stacks are a common data structure. The pop()
method is often used to implement the "Last In, First Out" (LIFO) principle in stacks.
1let stack = [];
2stack.push(10);
3stack.push(20);
4stack.push(30);
5console.log(stack.pop()); // Outputs: 30
6console.log(stack); // Outputs: [10, 20]
For a deeper understanding of stack operations, you might want to check out our Introduction to Web Development.
Technique 3: Combining pop()
with push()
The push()
method adds one or more elements to the end of an array. Combining pop()
with push()
allows for dynamic array modifications.
1let items = ["Pen", "Pencil", "Eraser"];
2items.push("Sharpener");
3console.log(items.pop()); // Outputs: Sharpener
4console.log(items); // Outputs: ["Pen", "Pencil", "Eraser"]
You can learn more about array manipulation in our Learn JavaScript course.
Technique 4: Using pop()
in Real-time Applications
In real-time applications, such as undo functionalities, pop()
can be very useful. For instance, in a text editor, you might use pop()
to remove the last action performed by the user.
1let actions = ["type", "bold", "italic"];
2actions.pop(); // Undoing the last action
3console.log(actions); // Outputs: ["type", "bold"]
Technique 5: Handling Empty Arrays
It's important to handle cases where the array might be empty when using pop()
. This prevents errors in your code.
1let emptyArray = [];
2let result = emptyArray.pop();
3console.log(result); // Outputs: undefined
4console.log(emptyArray); // Outputs: []
Compatibility with Major Web Browsers
The pop()
method is widely supported across all major web browsers, including Chrome, Firefox, Safari, Edge, and Internet Explorer. This makes it a reliable choice for web development projects.
Summary
In this tutorial, we've explored five powerful techniques for using the pop()
method in JavaScript. From basic array manipulation to real-time application scenarios, pop()
is a versatile tool in your JavaScript toolkit. Understanding its syntax, return values, and practical applications will enhance your coding skills.
For more in-depth learning, consider exploring our HTML Fundamentals Course and Learn CSS Introduction. Additionally, you can read more about JavaScript array methods on MDN Web Docs.
By mastering these techniques, you'll be well-equipped to handle complex array operations in your future projects. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
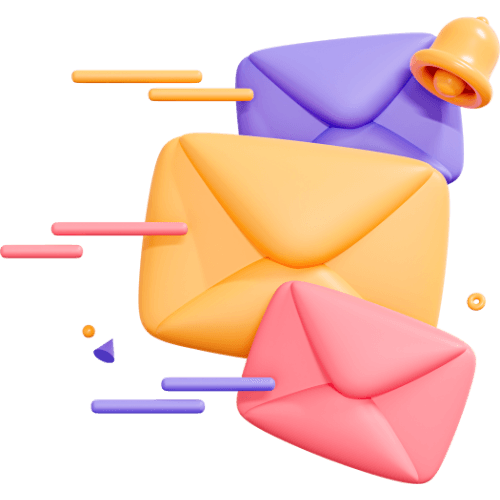
Related articles
9 Articles
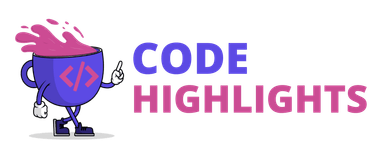
Copyright © Code Highlights 2025.