7 Essential JavaScript Print Object Properties Techniques

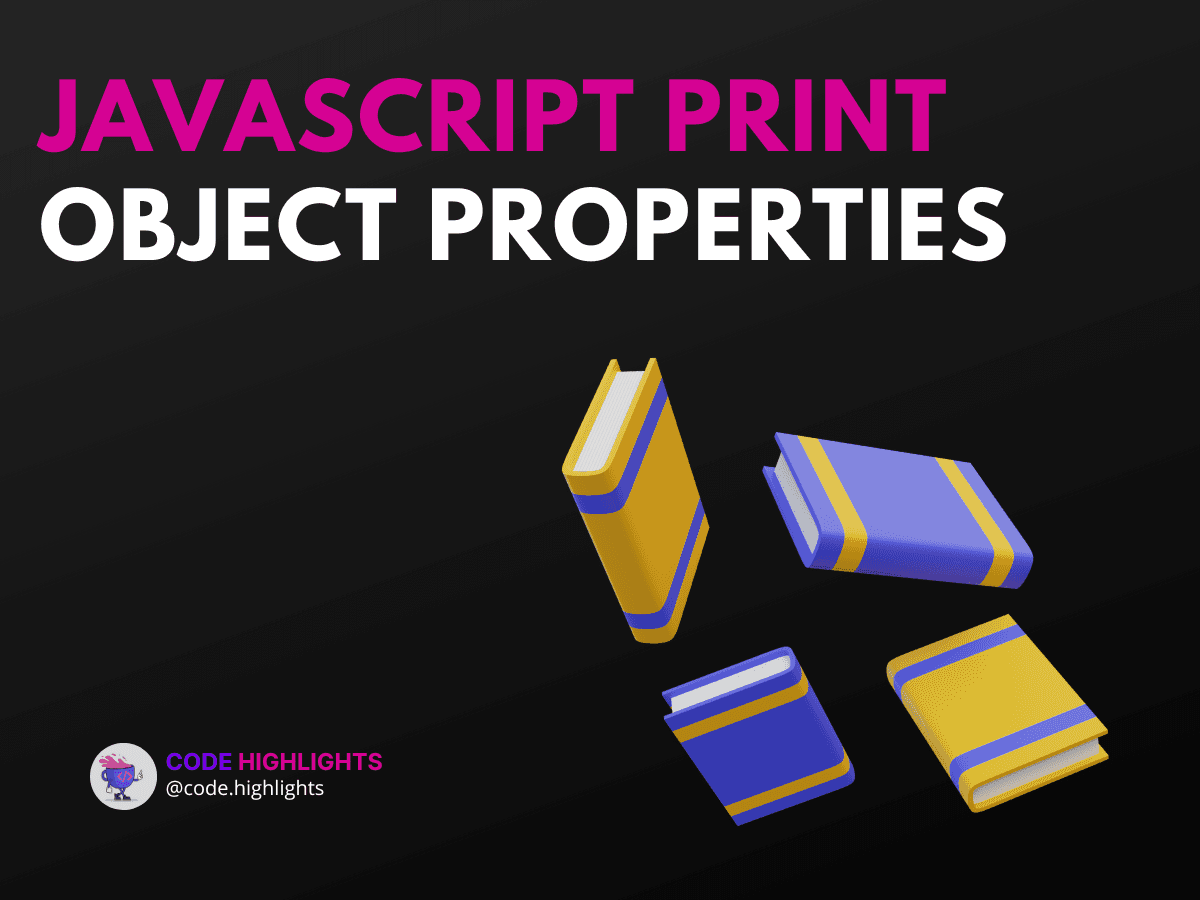
- 1. Using console.log()
- Key Points:
- 2. Looping Through Properties with for...in
- Key Points:
- 3. Using Object.keys()
- Key Points:
- 4. Displaying Properties with JSON.stringify()
- Key Points:
- 5. Printing Property Names with Object.entries()
- Key Points:
- 6. Logging Properties with console.table()
- Key Points:
- 7. Using Reflect.ownKeys()
- Key Points:
- Browser Compatibility
- Summary
Understanding how to javascript print object properties is essential for any developer. Objects are a fundamental part of JavaScript, allowing you to store collections of data and more complex entities. By learning how to print object properties, you can better debug your code and understand the structure of your data.
Let's dive right in with a simple example to get started:
This snippet creates an object called person
and prints it to the console. Now, let's explore various techniques to print properties from this object.
1. Using console.log()
The simplest way to display properties of an object in JavaScript is by using console.log()
. This method prints the entire object or specific properties.
Key Points:
- Use dot notation to access properties.
- You can also use bracket notation:
person["city"]
.
2. Looping Through Properties with for...in
Another technique is using a for...in
loop. This allows you to iterate over all properties of an object.
Key Points:
- This will print each property name and its value.
- Useful for dynamically accessing properties.
3. Using Object.keys()
You can use Object.keys()
to get an array of the object's keys and then print them.
Key Points:
- This method is useful if you only need the keys.
- It provides a cleaner approach when working with arrays.
4. Displaying Properties with JSON.stringify()
If you want a quick way to print all properties in a readable format, use JSON.stringify()
.
Key Points:
- The second parameter is a replacer, and the third is for indentation.
- Great for logging complex objects.
5. Printing Property Names with Object.entries()
To print both property names and values, Object.entries()
returns an array of key-value pairs.
Key Points:
- This method is similar to
Object.keys()
but includes values. - Useful for destructuring in loops.
6. Logging Properties with console.table()
For a more organized view, you can use console.table()
to display properties in a table format.
Key Points:
- This method is particularly helpful for debugging.
- It provides a clear representation of object properties.
7. Using Reflect.ownKeys()
If you want to include non-enumerable properties, use Reflect.ownKeys()
.
1const obj = Object.create({}, {
2 hidden: { value: 'secret', enumerable: false },
3 visible: { value: 'shown', enumerable: true }
4});
5
6console.log(Reflect.ownKeys(obj));
Key Points:
- This will return all property keys, including hidden ones.
- Useful for advanced object manipulation.
Browser Compatibility
Most of these methods work across major web browsers like Chrome, Firefox, Safari, and Edge. However, always check compatibility for newer features like Reflect.ownKeys()
to ensure they work in your target environment.
Summary
In this tutorial, we explored seven essential techniques for javascript print object properties. We covered using console.log()
, looping with for...in
, and employing methods like Object.keys()
, JSON.stringify()
, and console.table()
. Each technique has its unique advantages, making it easier to display and understand your objects.
If you're looking to deepen your JavaScript knowledge, consider checking out our JavaScript Course or explore HTML Fundamentals and CSS Introduction.
For further reading on JavaScript objects and their properties, you can refer to resources from MDN Web Docs and W3Schools.
Now that you have these techniques, you can confidently print object properties and enhance your JavaScript skills!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
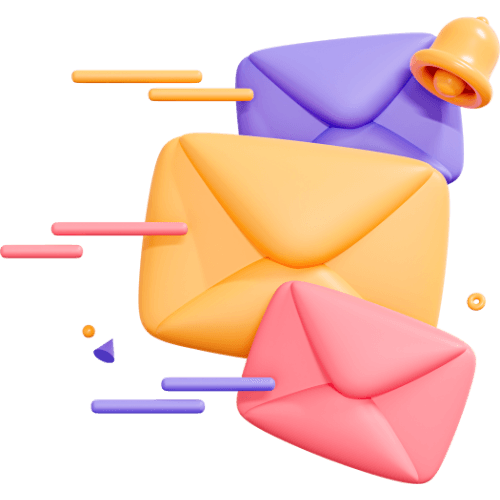
Related articles
9 Articles
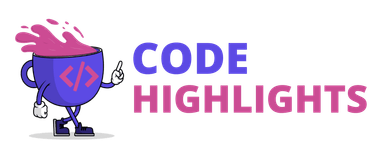
Copyright © Code Highlights 2025.