How to Implement a JavaScript Queue for Better Performance

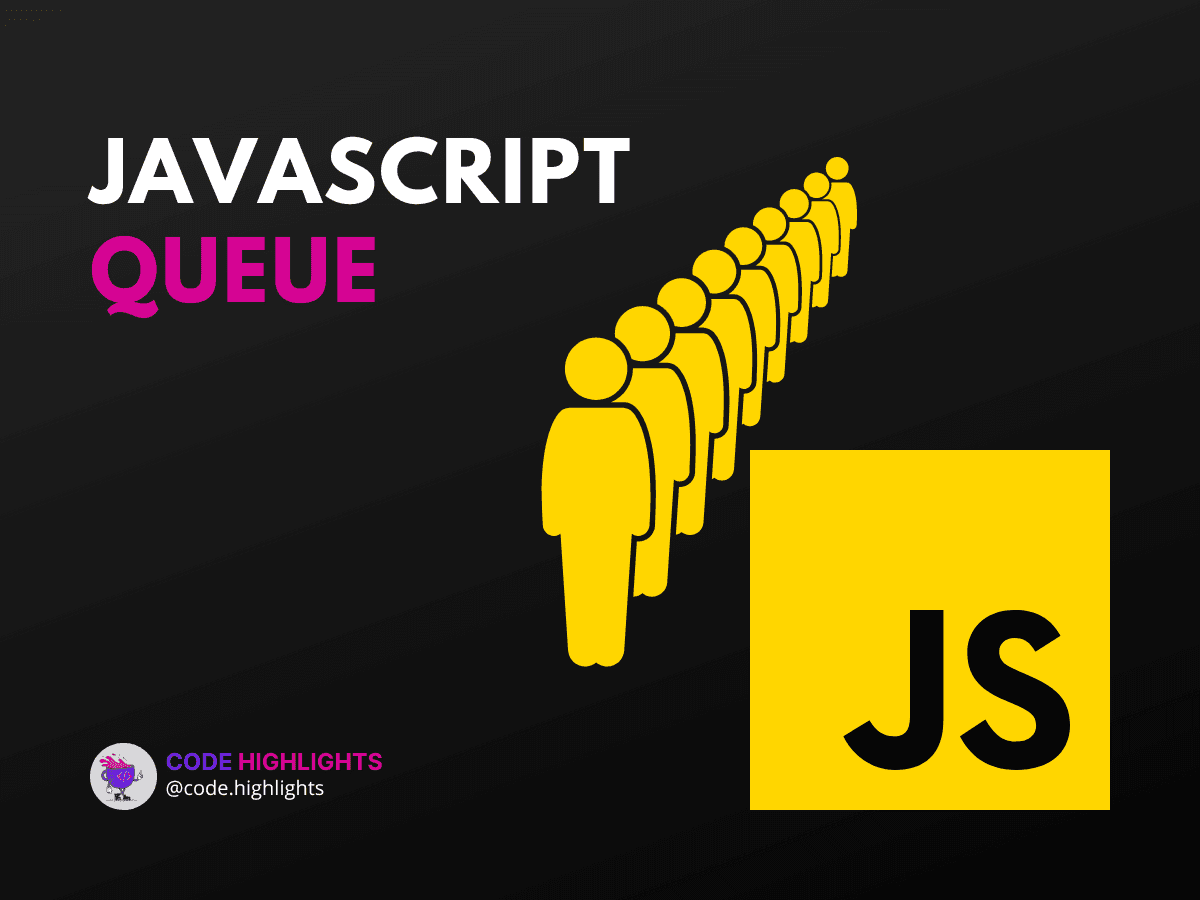
- Understanding Queues
- Step-by-Step Queue Implementation
- Step 1: Setting Up the Queue Class
- Step 2: Adding Elements with enqueue
- Step 3: Removing Elements with dequeue
- Step 4: Checking if the Queue is Empty
- Step 5: Putting It All Together
- Why Use a Queue?
In the bustling world of web development, performance is key. One way to keep your applications running smoothly is by managing data efficiently. Enter the JavaScript queue, a fundamental structure in computer science that can streamline your data processing. Let's dive into how you can implement a javascript queue
to enhance your application's performance.
1// Simple JavaScript Queue Implementation
2function Queue() {
3 this.elements = [];
4}
5
6Queue.prototype.enqueue = function (e) {
7 this.elements.push(e);
8};
9
10Queue.prototype.dequeue = function () {
11 return this.elements.shift();
12};
13
14Queue.prototype.isEmpty = function () {
15 return this.elements.length === 0;
16};
17
18// Usage
19var queue = new Queue();
20queue.enqueue(1);
21queue.enqueue(2);
22console.log(queue.dequeue()); // Outputs 1
This introductory code snippet provides a sneak peek at the syntax we'll be using. Now, let's break down what a queue is and how you can implement one that boosts your code's efficiency.
Understanding Queues
Imagine you're waiting in line for your favorite coffee. The first person in line gets served and leaves, then the next, and so on. This is how a queue operates: it follows a first-in, first-out (FIFO) principle. In JavaScript, a queue can help manage tasks, operations, or any data collection where the order of processing is crucial.
Step-by-Step Queue Implementation
Step 1: Setting Up the Queue Class
Firstly, you need a container for your queue elements:
Here, we're using a simple array to store the items in our queue.
Step 2: Adding Elements with enqueue
To add an element to the back of the queue:
The push
method comes in handy to insert items at the end of our array.
Step 3: Removing Elements with dequeue
Removing an item from the front is just as easy:
The shift
method pops off the first item, maintaining our FIFO structure.
Step 4: Checking if the Queue is Empty
To prevent errors, always check if the queue is empty before dequeuing:
This function returns true
when there are no more items to process.
Step 5: Putting It All Together
Now, let's see our queue in action:
1var queue = new Queue();
2queue.enqueue('Learn JavaScript');
3queue.enqueue('Practice queues');
4console.log(queue.dequeue()); // Outputs 'Learn JavaScript'
You've just processed your first queue item!
Why Use a Queue?
Queues are perfect for scenarios where order matters, like managing user inputs, handling asynchronous tasks, or processing streams of data. By ensuring a well-organized flow of operations, queues can significantly boost your application's performance.
For those looking to deepen their understanding of JavaScript, consider enrolling in this JavaScript course. And if you're starting out, brushing up on HTML fundamentals and CSS introduction will solidify your web development foundation, which you can build upon with an introduction to web development.
To further enrich your knowledge, explore these reputable sources:
- Mozilla Developer Network (MDN) on JavaScript Arrays
- W3Schools JavaScript Queue Tutorial
- GeeksforGeeks on Queue Data Structure
Implementing a javascript queue
is just the beginning. Remember, practice makes perfect. Try extending the queue with more functionalities like peeking at the next item or tracking the queue's size. The more you play with the code, the better you'll understand how it can serve your performance goals. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
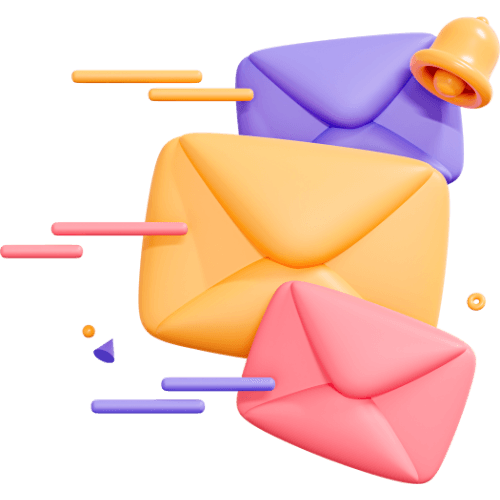
Related articles
9 Articles
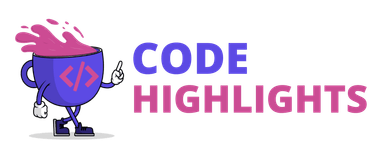
Copyright © Code Highlights 2025.