How to JavaScript Read CSV for Easy Data Handling

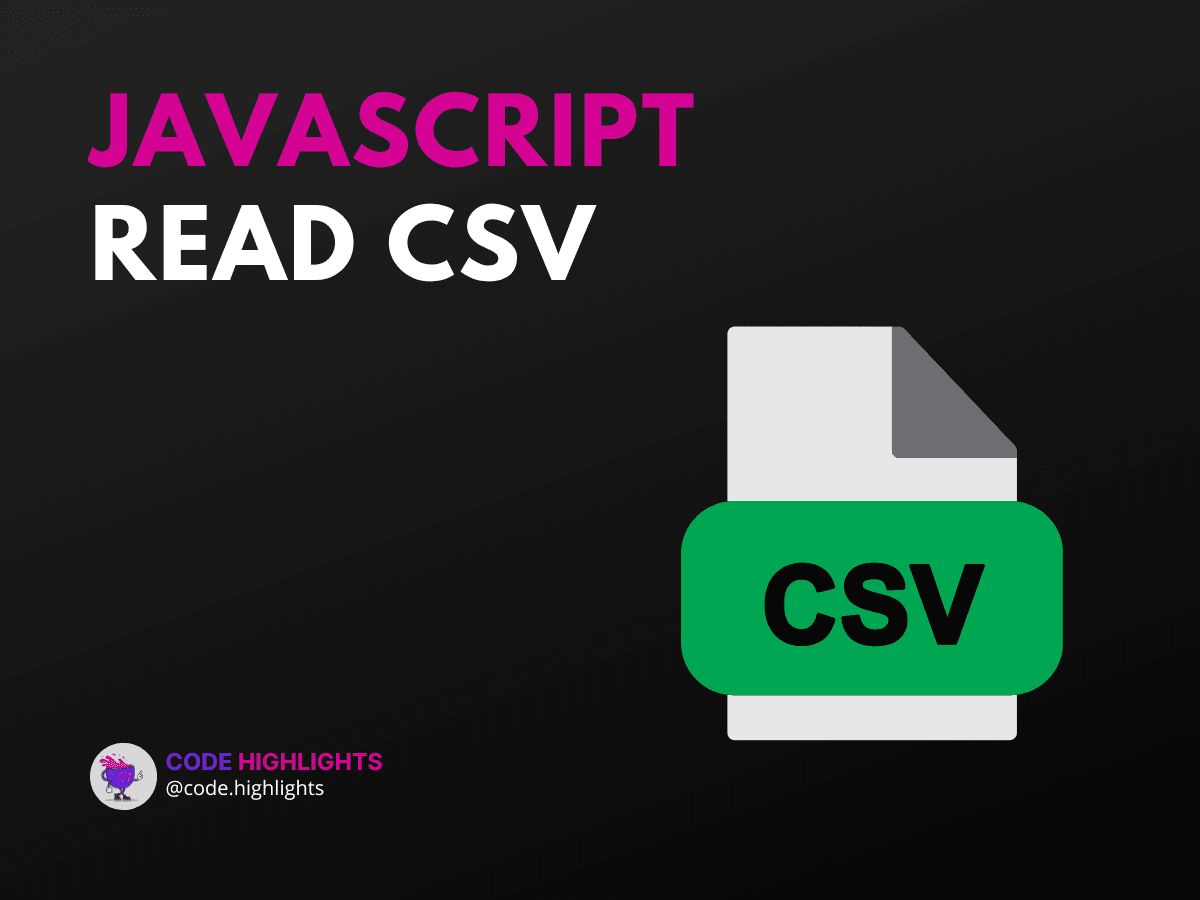
- Introduction to Reading CSV Files in JavaScript
- Understanding the Syntax
- Parameters and Return Values
- Parsing the CSV Data
- Practical Examples
- Example 1: Reading a CSV File from a URL
- Example 2: Handling Local CSV Files
- Example 3: Converting CSV Data to JSON
- Compatibility with Major Web Browsers
- Summary
Reading CSV files in JavaScript can greatly simplify data manipulation tasks. This tutorial will walk you through the process of reading CSV files using JavaScript, making it easy to handle and process data from these files.
Introduction to Reading CSV Files in JavaScript
CSV (Comma-Separated Values) files are commonly used to store tabular data. Each line in a CSV file represents a row, and each value is separated by a comma. Let's get started with a simple example:
1const csvData = `name,age,city
2John,30,New York
3Jane,25,Los Angeles
4Sam,35,Chicago`;
5
6console.log(csvData);
This snippet creates a string that mimics the content of a CSV file. Now, let's dive into how we can read and parse this data in JavaScript.
Understanding the Syntax
To read a CSV file in JavaScript, we typically use the fetch
API to retrieve the file and then parse its contents. Here’s a basic function to fetch and read a CSV file:
1async function readCSV(url) {
2 const response = await fetch(url);
3 const data = await response.text();
4 return data;
5}
Parameters and Return Values
- Parameters: The
url
parameter specifies the location of the CSV file. - Return Values: The function returns the content of the CSV file as a string.
Parsing the CSV Data
Once we have the CSV data as a string, we need to parse it into a more usable format, such as an array of objects. Here’s a function to do that:
1function parseCSV(data) {
2 const lines = data.split('\n');
3 const headers = lines[0].split(',');
4
5 return lines.slice(1).map(line => {
6 const values = line.split(',');
7 return headers.reduce((obj, header, index) => {
8 obj[header] = values[index];
9 return obj;
10 }, {});
11 });
12}
Practical Examples
Example 1: Reading a CSV File from a URL
This example demonstrates how to read a CSV file from a remote URL and parse it into an array of objects.
1async function fetchAndParseCSV(url) {
2 const csvData = await readCSV(url);
3 const parsedData = parseCSV(csvData);
4 console.log(parsedData);
5}
6
7fetchAndParseCSV('https://example.com/data.csv');
Example 2: Handling Local CSV Files
If you have a local CSV file, you can read it using the FileReader API.
1document.getElementById('fileInput').addEventListener('change', function(event) {
2 const file = event.target.files[0];
3 const reader = new FileReader();
4
5 reader.onload = function(e) {
6 const csvData = e.target.result;
7 const parsedData = parseCSV(csvData);
8 console.log(parsedData);
9 };
10
11 reader.readAsText(file);
12});
Example 3: Converting CSV Data to JSON
This example shows how to convert CSV data into a JSON format, which is often required for further processing.
1async function csvToJson(url) {
2 const csvData = await readCSV(url);
3 const jsonData = parseCSV(csvData);
4 return JSON.stringify(jsonData, null, 2);
5}
6
7csvToJson('https://example.com/data.csv').then(json => console.log(json));
Compatibility with Major Web Browsers
Reading CSV files using JavaScript is compatible with all major web browsers, including Chrome, Firefox, Safari, Edge, and Opera. The fetch
API and FileReader API are widely supported, ensuring that your code will work seamlessly across different platforms.
Summary
In this tutorial, we covered how to read and parse CSV files in JavaScript. We started with a simple example, explored the syntax, and provided multiple practical examples to illustrate different use cases. By following these steps, you can easily handle and manipulate CSV data in your JavaScript applications.
For more detailed learning, consider exploring our JavaScript course, HTML fundamentals course, and CSS introduction course.
For additional information on handling CSV files in JavaScript, you can refer to this guide on reading CSV files and this tutorial on parsing CSV data.
Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
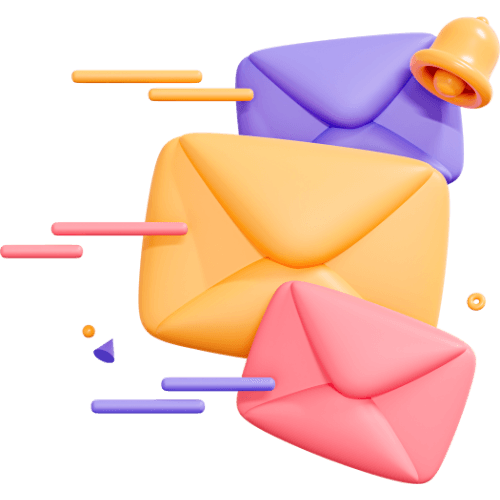
Related articles
9 Articles
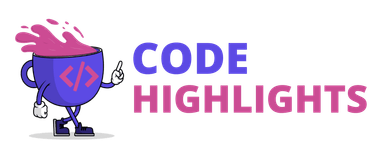
Copyright © Code Highlights 2025.