7 Javascript Regex B Patterns for Powerful Text Manipulation

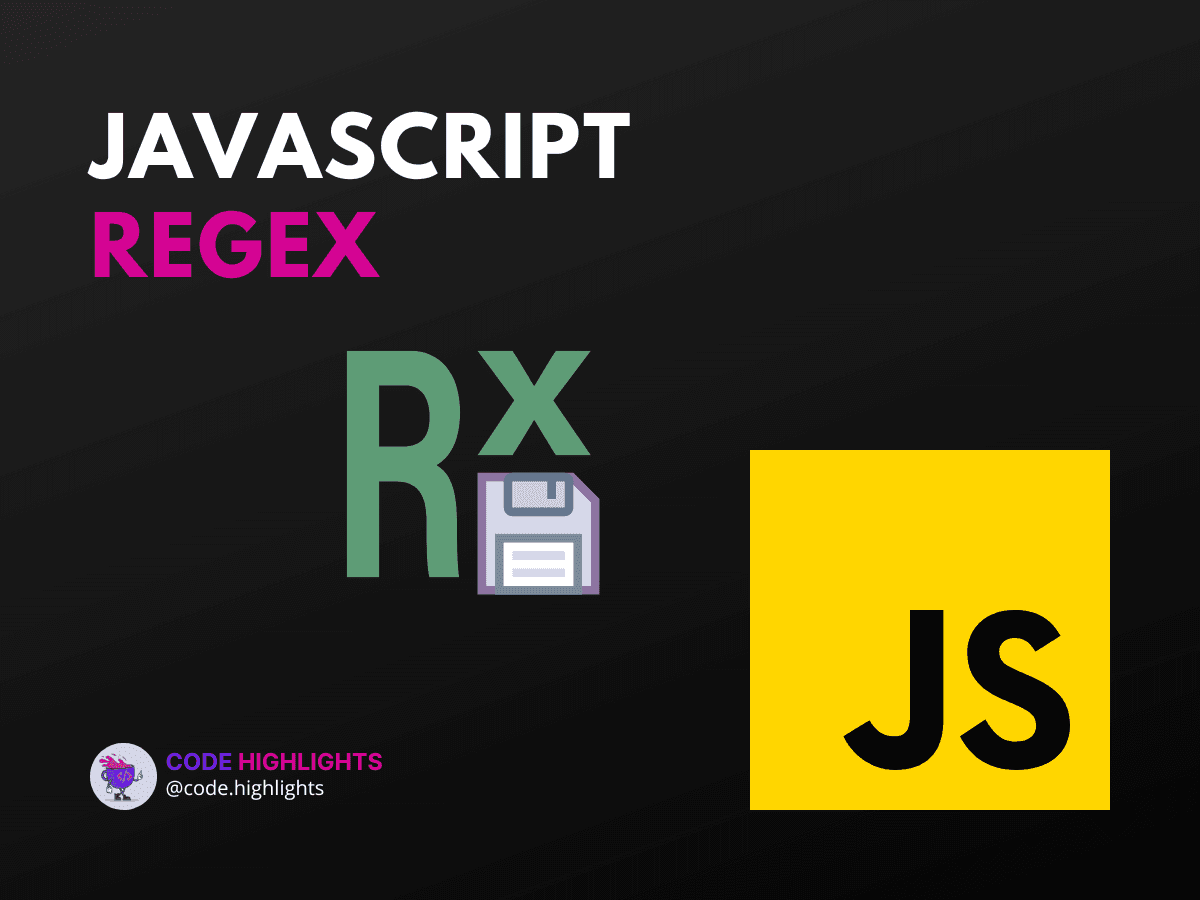
- Pattern 1: Matching Whole Words
- Pattern 2: Word Boundary at the Start of a String
- Pattern 3: Word Boundary at the End of a String
- Pattern 4: Using Word Boundaries with Quantifiers
- Pattern 5: Excluding Specific Words
- Pattern 6: Capturing Words Before or After a Specific Word
- Pattern 7: Validating Password Strength
Regular expressions, or regex, are a powerful tool in any programmer's arsenal, especially when dealing with text manipulation and pattern matching. In JavaScript, regex patterns offer a concise and efficient way to search, replace, and validate strings. Today, we're going to explore seven practical Javascript regex patterns that focus on the use of the \b
metacharacter, which represents a word boundary.
This tutorial will enhance your understanding of how to harness the power of \b
in regex to make your JavaScript code more robust and your text processing more precise. Let's dive right in with a simple example:
1let sampleText = "The quick brown fox.";
2let regexPattern = /\bquick\b/;
3console.log(sampleText.match(regexPattern)); // Outputs: ["quick"]
In the example above, the regex pattern matches the word "quick" only when it appears as a whole word, not as part of another word, thanks to the \b
metacharacter.
Pattern 1: Matching Whole Words
To start, let's use the \b
metacharacter to match whole words within a string.
1let text = "Learning at code school is fun.";
2let wordRegex = /\bcode\b/;
3console.log(text.match(wordRegex)); // Outputs: ["code"]
This pattern ensures that "code" is matched as an independent word, not as a substring of another word like "decode" or "encode."
Pattern 2: Word Boundary at the Start of a String
You can use \b
at the beginning of your regex pattern to ensure the match starts at the beginning of a word.
1let greeting = "Hello world!";
2let startWordRegex = /\bHello/;
3console.log(greeting.match(startWordRegex)); // Outputs: ["Hello"]
This pattern is useful when you want to find words that start with a specific sequence of characters.
Pattern 3: Word Boundary at the End of a String
Similarly, placing \b
at the end of your pattern ensures the match is at the end of a word.
1let farewell = "Goodbye cruel world";
2let endWordRegex = /world\b/;
3console.log(farewell.match(endWordRegex)); // Outputs: ["world"]
This can be particularly helpful when you're looking to replace or remove specific words from a string.
Pattern 4: Using Word Boundaries with Quantifiers
Combine \b
with quantifiers to match words of a certain length or pattern.
1let animalSounds = "Meow, moo, baa, oink, woof";
2let threeLetterAnimalSoundRegex = /\b\w{3}\b/g;
3console.log(animalSounds.match(threeLetterAnimalSoundRegex)); // Outputs: ["moo", "baa", "oink", "woof"]
This pattern matches all three-letter words representing animal sounds.
Pattern 5: Excluding Specific Words
Use \b
with a negative lookahead to exclude specific words from your match.
1let fruits = "Apples and oranges are delicious.";
2let noOrangesRegex = /\b(?!oranges\b)\w+/g;
3console.log(fruits.match(noOrangesRegex)); // Outputs: ["Apples", "and", "are", "delicious."]
This pattern will match every word except "oranges."
Pattern 6: Capturing Words Before or After a Specific Word
\b
can help you capture words that appear right before or after a specific keyword.
1let bookQuote = "It was the best of times, it was the worst of times.";
2let beforeAfterRegex = /\b(\w+)\s+of\s+(\w+)\b/g;
3console.log(bookQuote.match(beforeAfterRegex)); // Outputs: ["best of times", "worst of times"]
This pattern captures phrases in the format "X of Y."
Pattern 7: Validating Password Strength
Finally, use \b
to create a regex pattern that validates password strength by ensuring the presence of certain character types.
1let password = "StrongP@ssw0rd";
2let strongPasswordRegex = /^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}\b/;
3console.log(strongPasswordRegex.test(password)); // Outputs: true
This pattern checks for a mix of uppercase, lowercase, digits, and special characters, ensuring a strong password.
To further your JavaScript journey, consider exploring courses on JavaScript fundamentals, HTML basics, an introduction to CSS, or a full introduction to web development.
For additional reading on regex patterns and their applications in JavaScript, check out resources from MDN Web Docs, W3Schools, or JavaScript.info.
By mastering these seven Javascript regex \b
patterns, you'll be able to perform more sophisticated text manipulations and elevate your coding skills. Remember, practice makes perfect, so try incorporating these patterns into your next project!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
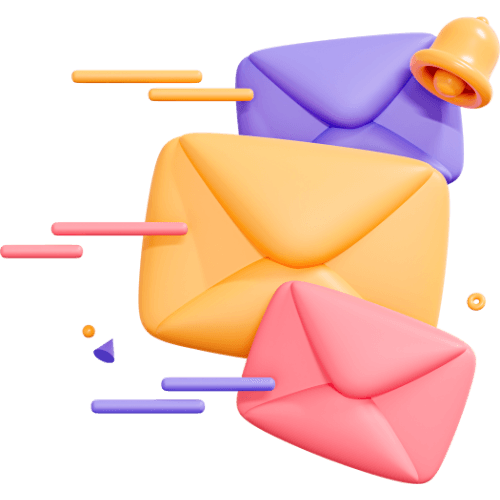
Related articles
114 Articles
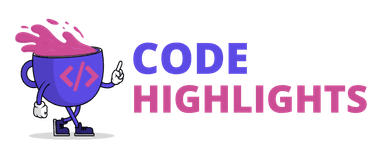
Copyright © Code Highlights 2024.