The Secret to Mastering JavaScript Regex with Variable

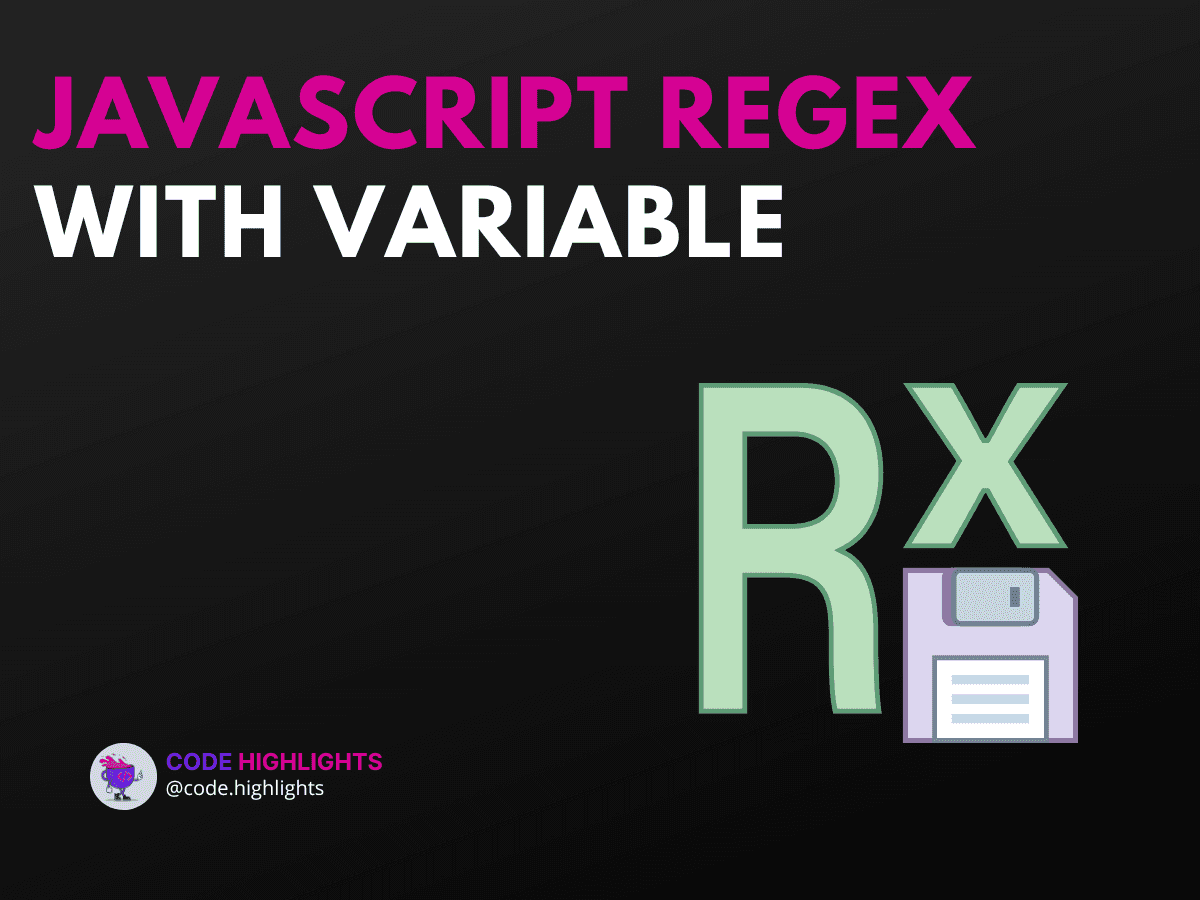
- Quick Code Example
- Understanding Regex Syntax
- Parameters and Return Values
- Example 1: Dynamic Search with Variables
- Using Variables for User Input
- Example 2: Validating Email Addresses
- Regex for Email Validation
- Example 3: Finding All Matches
- Extracting Data from Text
- Browser Compatibility
- Conclusion
JavaScript Regular Expressions (Regex) can seem complex at first, but they are powerful tools for pattern matching in strings. Understanding how to use Regex with variables allows you to create dynamic patterns that can adapt to different inputs. This tutorial will guide you through the essentials of using JavaScript Regex with variables, providing clear examples and explanations to help you master this skill.
Quick Code Example
Before diving into the details, here’s a quick example to illustrate how to use a variable with a Regex pattern:
1let searchTerm = "cat";
2let regex = new RegExp(searchTerm, 'i');
3console.log(regex.test("I have a cat.")); // true
In this example, we create a Regex pattern using a variable, making our search dynamic.
Understanding Regex Syntax
Regular expressions have a specific syntax. Here are some key components:
/pattern/flags
: The basic structure.g
: Global search.i
: Case-insensitive search.m
: Multiline search.
When using a variable, you can create a Regex object dynamically:
Parameters and Return Values
When you create a Regex with new RegExp()
, you can pass two parameters:
- Pattern: The string pattern you want to match.
- Flags: Optional flags to modify the search behavior.
The return value is a Regex object that you can use with methods like .test()
or .exec()
.
Example 1: Dynamic Search with Variables
Using Variables for User Input
You can use variables to capture user input and create a Regex pattern:
1let userInput = prompt("Enter a word to search:");
2let regex = new RegExp(userInput, 'i');
3
4if (regex.test("This is a test sentence.")) {
5 console.log("Match found!");
6} else {
7 console.log("No match.");
8}
In this example, the user can enter any word, and the program checks if it exists in the string.
Example 2: Validating Email Addresses
Regex for Email Validation
You can also use Regex with variables for validating formats, like email addresses:
1let emailPattern = "^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}$";
2let emailRegex = new RegExp(emailPattern);
3
4let emailToTest = "example@test.com";
5console.log(emailRegex.test(emailToTest)); // true
This Regex checks if the entered string is a valid email format.
Example 3: Finding All Matches
Extracting Data from Text
Using the global flag, you can find all instances of a pattern:
1let text = "Cats are great. I love my cat.";
2let searchWord = "cat";
3let findRegex = new RegExp(searchWord, 'gi');
4let matches = text.match(findRegex);
5
6console.log(matches); // [ 'cat', 'cat' ]
In this case, the Regex finds all occurrences of the word "cat," regardless of case.
Browser Compatibility
JavaScript Regex is well-supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. This means you can confidently use Regex in your web applications without worrying about compatibility issues.
Conclusion
Mastering JavaScript Regex with variables opens up a world of possibilities for string manipulation and validation. Whether you are searching for patterns, validating user input, or extracting data, understanding how to leverage Regex effectively can greatly enhance your programming skills. For more in-depth learning, consider exploring our courses on JavaScript, HTML Fundamentals, and CSS Introduction.
By practicing these examples and concepts, you'll be well on your way to becoming proficient in using JavaScript Regex with variables. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
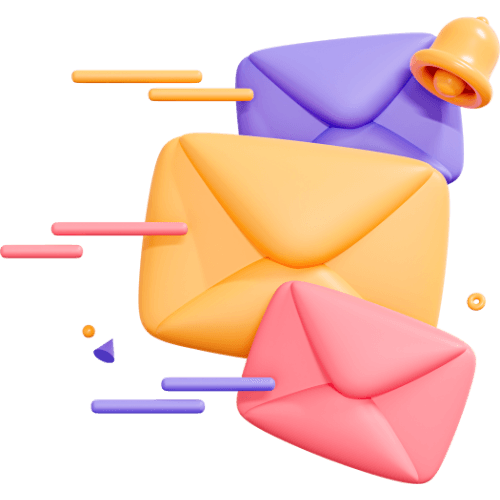
Related articles
9 Articles
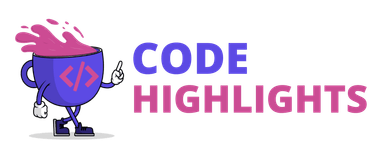
Copyright © Code Highlights 2025.