3 Fast Ways to Remove Duplicates from Array

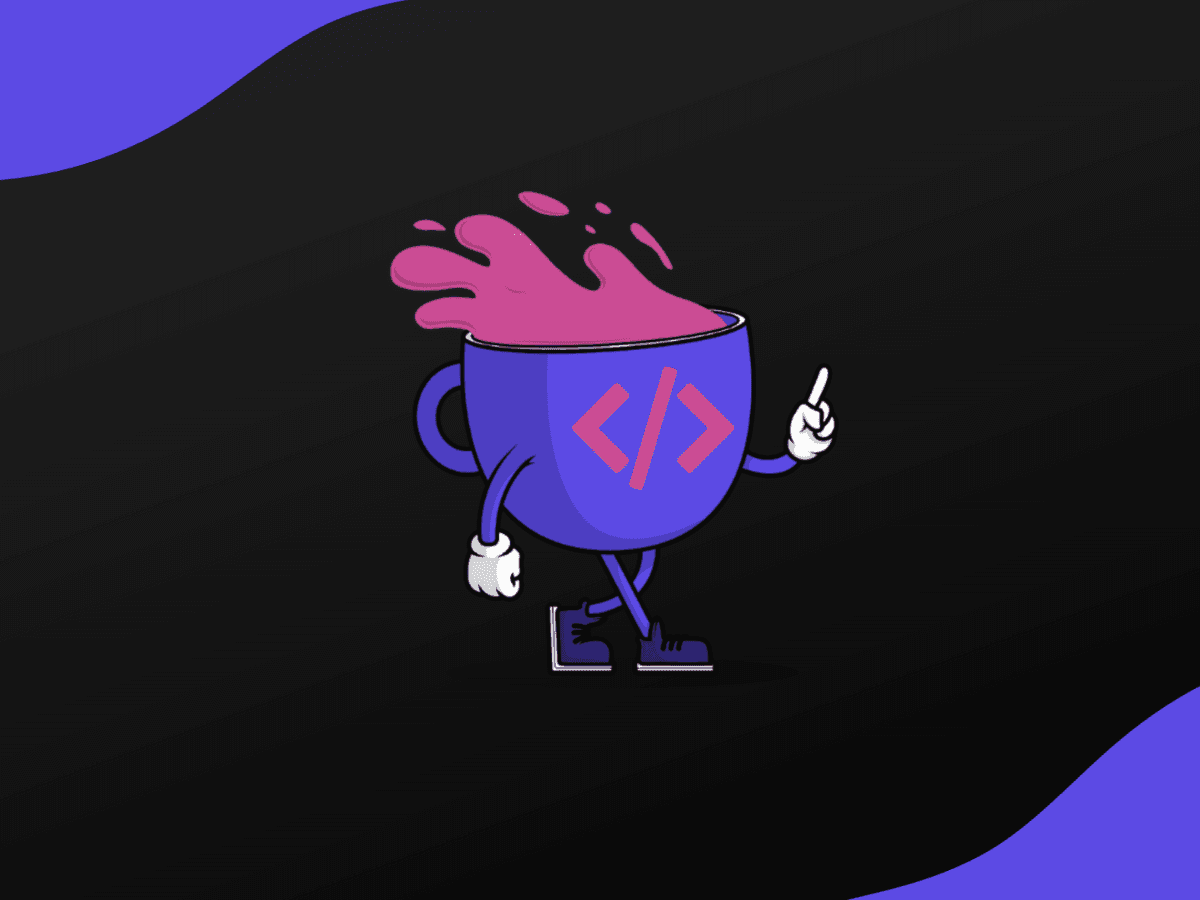
- Set Object
- filter() Method
- reduce() Method
- Comparison
- Browser Compatibility
In JavaScript, arrays are a versatile data structure that can hold any type of data and can be manipulated in various ways. One common task is to remove duplicates from an array. This tutorial will explore different methods for achieving this task.
Set
Object
The Set
object lets you store unique values. When an array is passed, it removes duplicate values.
filter()
Method
The filter()
method creates a new array with elements that pass the test implemented by the provided function.
1let unique = arr.filter((value, index) => arr.indexOf(value) === index); // returns [1, 2, 3, 4, 5]
reduce()
Method
The reduce()
method applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single output value.
1let unique = arr.reduce((accumulator, current) => {
2 return accumulator.includes(current) ? accumulator : [...accumulator, current];
3}, []); // returns [1, 2, 3, 4, 5]
Comparison
The quickest way to remove duplicates is by using the Set
object due to its internal optimization. However, if you need to support older browsers like IE, you might want to consider using the filter()
or reduce()
methods.
You can learn more about these methods in our JavaScript course. If you're new to web development, start with our Introduction to Web Development course.
To learn more about JavaScript arrays, refer to Mozilla Developer Network's guide on arrays.
Browser Compatibility
All three methods work in all modern browsers. The Set
object is not supported in IE. If you need to support IE, use the filter()
or reduce()
methods.
I hope this tutorial has been helpful in understanding how to remove duplicates from arrays in JavaScript.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
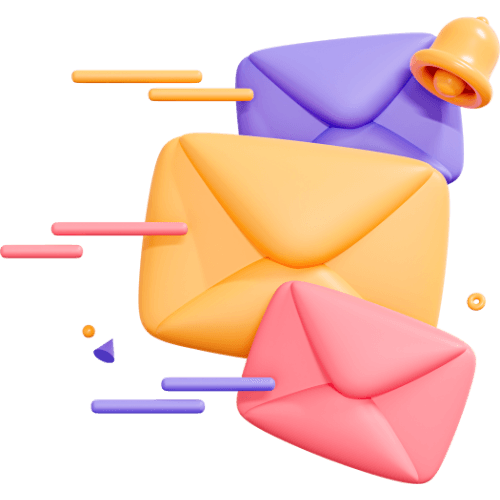
Related articles
9 Articles
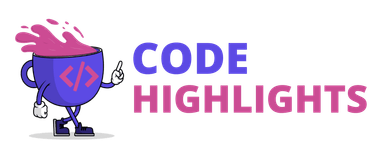
Copyright © Code Highlights 2025.