How do I replace all occurrences of a string in JavaScript?

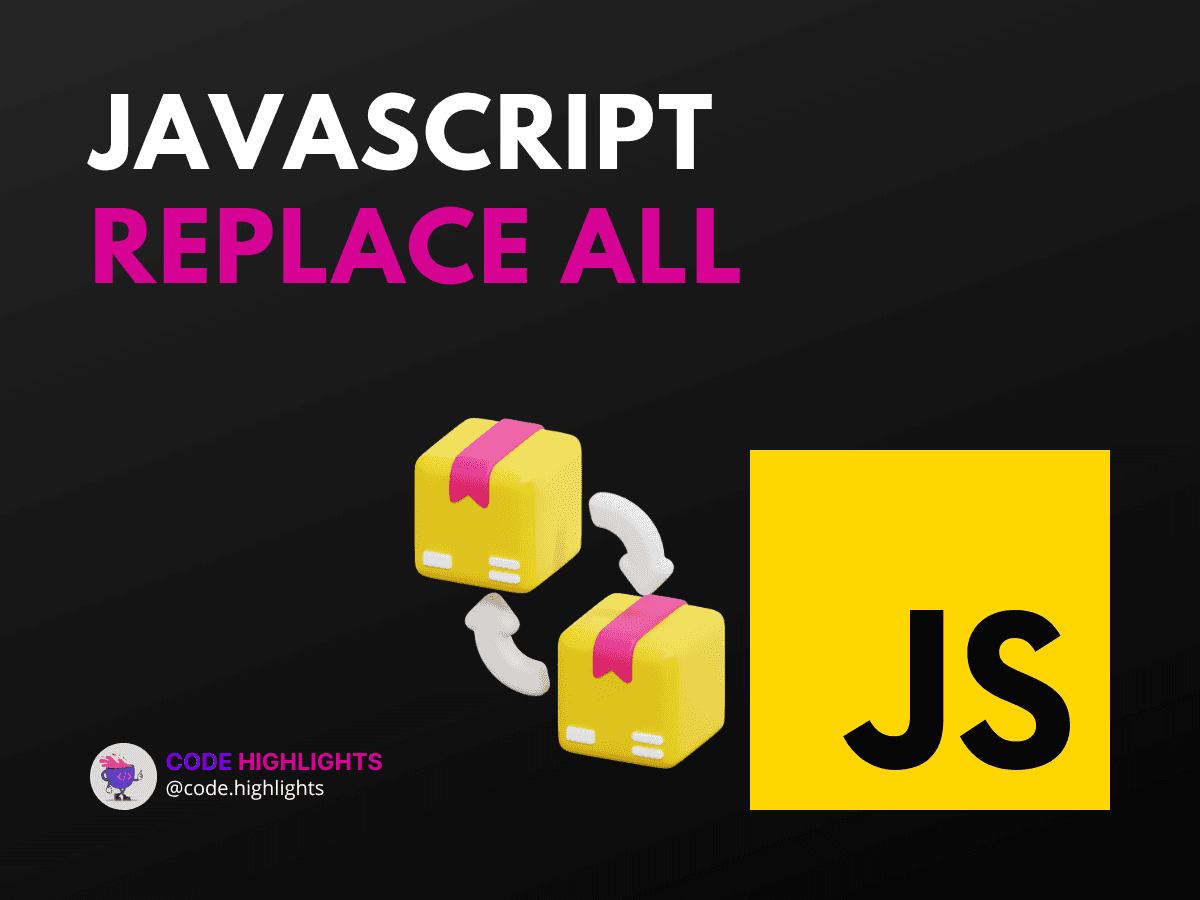
- Step-by-Step Guide to Replacing All String Occurrences
- Understanding the String.prototype.replace() Method
- Using String Literals
- Using Regular Expressions for Global Replacement
- Handling Case Sensitivity
- Escaping Special Characters
- Incorporating Function Replacements
- Conclusion
JavaScript is a powerful language that enables you to manipulate strings in various ways. One common task is replacing occurrences of a substring within a string. In this tutorial, we'll explore how to use JavaScript to replace all instances of a specific substring with another string, a technique often searched for as "javascript replace all."
Imagine you have a string: "The quick brown fox jumps over the lazy dog." Now, let's say you want to replace every occurrence of the word "the" with "a". Here's a simple code example that demonstrates how to do this:
1let text = "The quick brown fox jumps over the lazy dog.";
2let newText = text.replace(/the/gi, "a");
3console.log(newText); // Output: "a quick brown fox jumps over a lazy dog."
In the above example, we used the replace
method with a regular expression. Notice the gi
modifier; g
stands for global, and i
stands for case-insensitive, ensuring all instances are replaced regardless of their case.
Step-by-Step Guide to Replacing All String Occurrences
Understanding the String.prototype.replace()
Method
The replace
method is a part of the String object's prototype. It takes two parameters: the substring to be replaced (or a regular expression) and the replacement string.
Using String Literals
If you're only replacing a single occurrence or a substring that doesn't repeat, you can use a simple string literal:
1let sentence = "Hello World!";
2let newSentence = sentence.replace("World", "Universe");
3console.log(newSentence); // Output: "Hello Universe!"
Using Regular Expressions for Global Replacement
For global replacement, you'll need to use a regular expression with the g
flag:
1let phrase = "Apples and bananas.";
2let newPhrase = phrase.replace(/a/g, "o");
3console.log(newPhrase); // Output: "Opples ond bononos."
Handling Case Sensitivity
To make your replacement case-insensitive, add the i
flag to your regular expression:
1let text = "Summer is here and summer is fun.";
2let newText = text.replace(/summer/gi, "winter");
3console.log(newText); // Output: "Winter is here and winter is fun."
Escaping Special Characters
If the string you're replacing contains special characters (like .
, *
, or ?
), you'll need to escape them with a backslash (\
):
1let info = "Visit example.com today!";
2let newInfo = info.replace(/example\.com/g, "example.net");
3console.log(newInfo); // Output: "Visit example.net today!"
Incorporating Function Replacements
For more complex replacements, you can pass a function as the second parameter:
1let story = "There were 3 pigs and 4 wolves.";
2let newStory = story.replace(/\d+/g, (match) => match * 2);
3console.log(newStory); // Output: "There were 6 pigs and 8 wolves."
Conclusion
Replacing all occurrences of a string in JavaScript is a straightforward process when you understand how to properly use the replace
method with regular expressions. This skill is essential for manipulating text and is a fundamental part of JavaScript programming.
If you're looking to expand your web development skills further, consider exploring our courses on HTML fundamentals, CSS, and a comprehensive introduction to web development.
For more detailed information about JavaScript's string manipulation capabilities, check out resources from Mozilla Developer Network, W3Schools, and Stack Overflow.
Remember, practice makes perfect. Try out these examples in your projects, and soon, replacing strings in JavaScript will become second nature!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
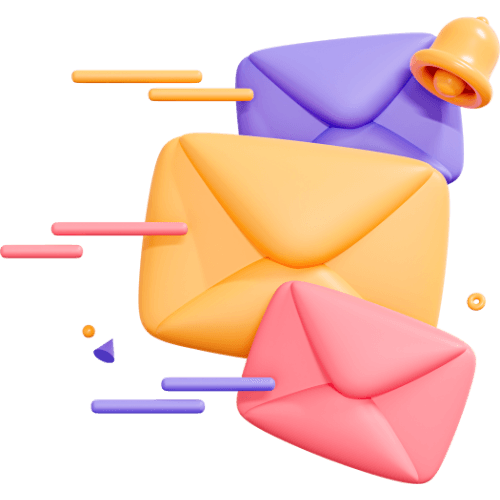
Related articles
9 Articles
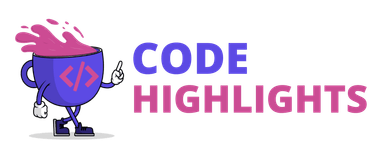
Copyright © Code Highlights 2025.