Master Javascript Replace at Index in 3 Easy Steps

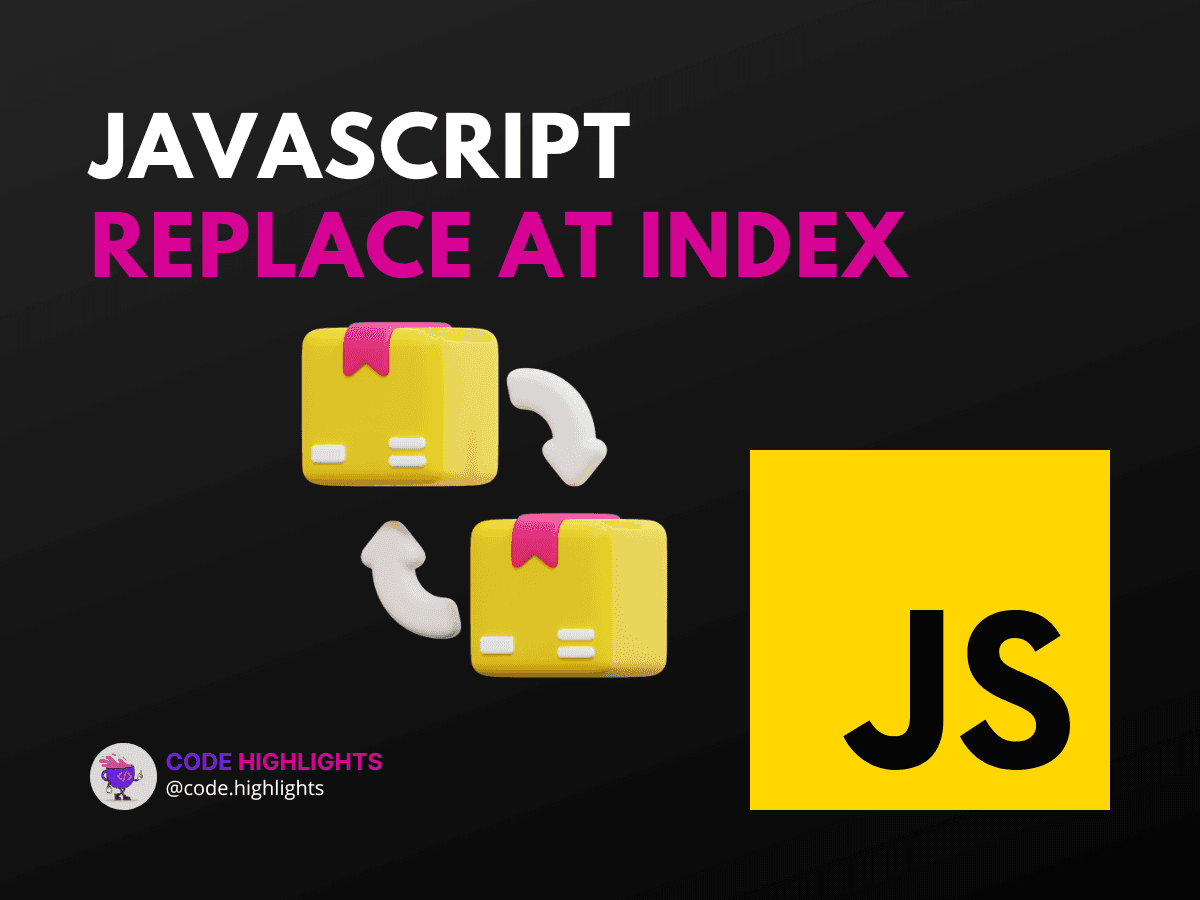
- Step 1: Understand the Problem
- Step 2: Crafting the Solution
- Step 3: Implement and Test
- Wrapping Up and Additional Resources
Have you ever been stuck on a coding project, wondering how to swap out a specific character or substring in JavaScript? Manipulating strings is a common task, and understanding how to javascript replace at index
is an essential skill for any aspiring developer. In this easy-to-follow tutorial, we'll uncover the simplicity behind this powerful string manipulation technique.
Before diving into the nitty-gritty, let's take a quick look at JavaScript's string syntax with a basic example:
Now, imagine you want to change the exclamation mark to a period. How would you do it? Keep reading to find out!
Step 1: Understand the Problem
Strings in JavaScript are immutable, meaning once a string is created, its characters cannot be changed directly. So, how do you replace at a particular index in JavaScript? You need to create a new string with the desired changes.
For instance, if you wish to change a value at an index in a string in JavaScript, you'll need to slice and concatenate parts of your original string around the index where the replacement is needed.
Step 2: Crafting the Solution
Here's a simple function that answers the question: How do I replace a string with an index?
1function replaceAtIndex(str, index, replacement) {
2 if (index >= str.length) {
3 return str;
4 }
5 return str.substring(0, index) + replacement + str.substring(index + 1);
6}
This function takes three parameters: the original string (str
), the index at which to replace (index
), and the new value (replacement
). It checks if the index is valid and then creates a new string with the replacement.
Step 3: Implement and Test
Let's see how to replace a string at a position in JavaScript with our new function:
1let originalString = "Hello, World!";
2let newString = replaceAtIndex(originalString, 12, '.');
3console.log(newString); // Outputs: Hello, World.
Voila! The exclamation mark has been replaced with a period.
Wrapping Up and Additional Resources
Now that you've mastered the javascript replace at index
technique, you're well-equipped to handle string manipulations in your future projects. If you're looking to expand your JavaScript knowledge, consider taking a JavaScript course. For those just starting out, understanding the basics through an HTML fundamentals course or a CSS introduction can also be beneficial. And if you're completely new to web development, an introduction to web development might be the perfect starting point.
As you continue your coding journey, remember to refer to reputable sources like MDN Web Docs or W3Schools for more in-depth explanations and examples. Happy coding!
Remember, practice makes perfect. Keep experimenting with different strings and indexes to solidify your understanding of this concept. With these three easy steps, you're now able to confidently tackle string replacements in JavaScript!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
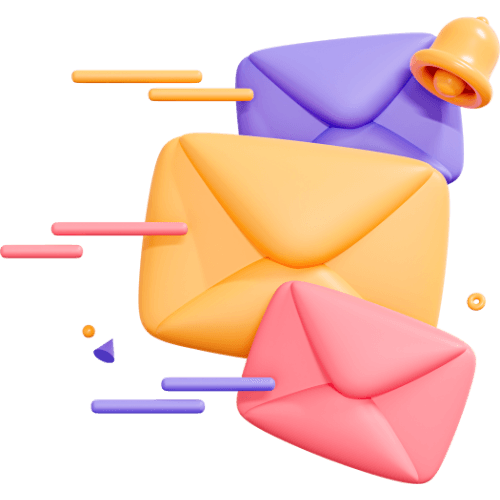
Related articles
9 Articles
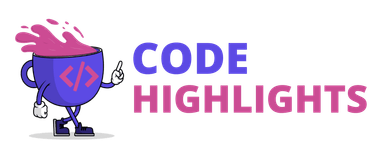
Copyright © Code Highlights 2025.