7 Powerful Tips for JavaScript Reverse For Loop Mastery

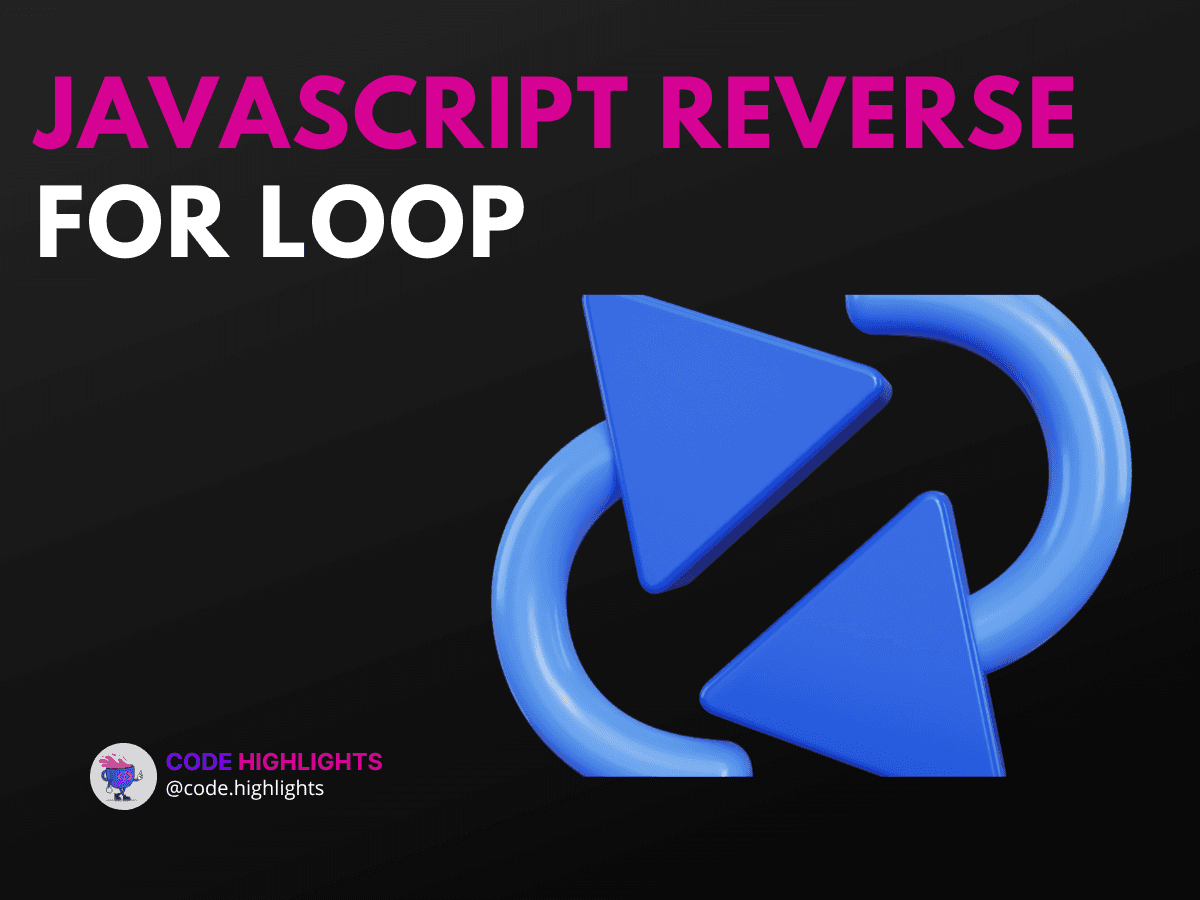
- Quick Code Example
- Understanding the Syntax
- Parameters:
- Return Values:
- Example 1: Simple Reverse Loop
- Title: Basic Reverse Loop
- Example 2: Reverse Loop with Conditions
- Title: Conditional Reverse Loop
- Example 3: Reverse Loop with Array Modification
- Title: Modifying Array Elements
- Example 4: Reversing a String
- Title: Reverse String with Loop
- Example 5: Iterating Over an Object in Reverse
- Title: Reverse Iteration Over Object Properties
- Compatibility with Browsers
- Summary
- Questions Answered:
When working with arrays in JavaScript, sometimes you may need to loop through them in reverse. Using a reverse for loop can be an efficient way to achieve this. This tutorial will explore the JavaScript reverse for loop, offering practical examples and tips to master this technique.
Quick Code Example
Here’s a simple example to get started:
1const numbers = [1, 2, 3, 4, 5];
2for (let i = numbers.length - 1; i >= 0; i--) {
3 console.log(numbers[i]);
4}
In this code, we start from the last element and move to the first. Let’s dive deeper into the syntax!
Understanding the Syntax
The basic syntax for a reverse for loop is:
Parameters:
- Initialization: Typically, you set your counter variable, starting at the last index.
- Condition: The loop continues while the counter is greater than or equal to zero.
- Decrement: You decrease the counter by one each time, moving backwards through the array.
Return Values:
A reverse for loop doesn't return a value but allows you to perform actions on each element.
Example 1: Simple Reverse Loop
Title: Basic Reverse Loop
1const fruits = ['apple', 'banana', 'cherry'];
2for (let i = fruits.length - 1; i >= 0; i--) {
3 console.log(fruits[i]);
4}
This example prints the fruits in reverse order: cherry, banana, apple.
Example 2: Reverse Loop with Conditions
Title: Conditional Reverse Loop
1const scores = [85, 92, 76, 88, 90];
2for (let i = scores.length - 1; i >= 0; i--) {
3 if (scores[i] > 80) {
4 console.log(scores[i]);
5 }
6}
Here, only scores higher than 80 are printed in reverse. This demonstrates how to use conditions effectively.
Example 3: Reverse Loop with Array Modification
Title: Modifying Array Elements
1const items = [1, 2, 3, 4, 5];
2for (let i = items.length - 1; i >= 0; i--) {
3 items[i] *= 2; // Double each item
4}
5console.log(items);
This example shows how to modify elements of an array while looping in reverse. The output will be [10, 8, 6, 4, 2]
.
Example 4: Reversing a String
Title: Reverse String with Loop
1const str = "Hello";
2let reversedStr = '';
3for (let i = str.length - 1; i >= 0; i--) {
4 reversedStr += str[i];
5}
6console.log(reversedStr); // Output: "olleH"
In this case, we build a new string by adding characters in reverse order.
Example 5: Iterating Over an Object in Reverse
Title: Reverse Iteration Over Object Properties
1const obj = { a: 1, b: 2, c: 3 };
2const keys = Object.keys(obj);
3for (let i = keys.length - 1; i >= 0; i--) {
4 console.log(`${keys[i]}: ${obj[keys[i]]}`);
5}
This example shows how to iterate over object properties in reverse order.
Compatibility with Browsers
Most modern web browsers support the JavaScript reverse for loop. Whether you're using Chrome, Firefox, Safari, or Edge, you can confidently implement this technique in your projects.
Summary
In this tutorial, we explored the JavaScript reverse for loop and its various applications. From basic loops to more complex scenarios, mastering this technique can enhance your coding skills. Remember, you can learn more about JavaScript through our JavaScript courses.
If you want to expand your knowledge further, check out our resources on HTML Fundamentals and CSS Introduction. Understanding these topics is essential for web development, which you can start with our Introduction to Web Development.
Questions Answered:
- How to reverse a for loop in JS? Use the length of the array and decrement the index.
- How to reverse using for loop? Start from the last index and loop until the first index.
- Is there a reverse method in JavaScript? Yes, the
reverse()
method is available for arrays. - How to iterate object in reverse JavaScript? Convert the object keys to an array and iterate in reverse.
By incorporating these techniques, you can efficiently handle data in reverse order, making your JavaScript skills even more powerful!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
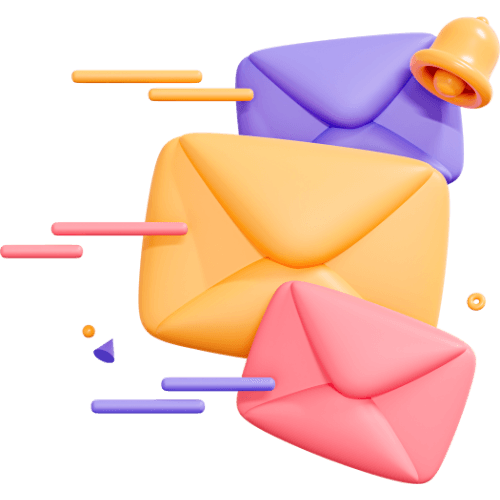
Related articles
9 Articles
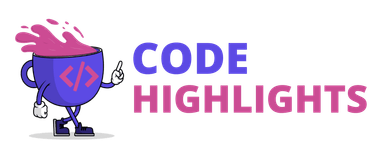
Copyright © Code Highlights 2025.