The Secret to Javascript Safe Programming

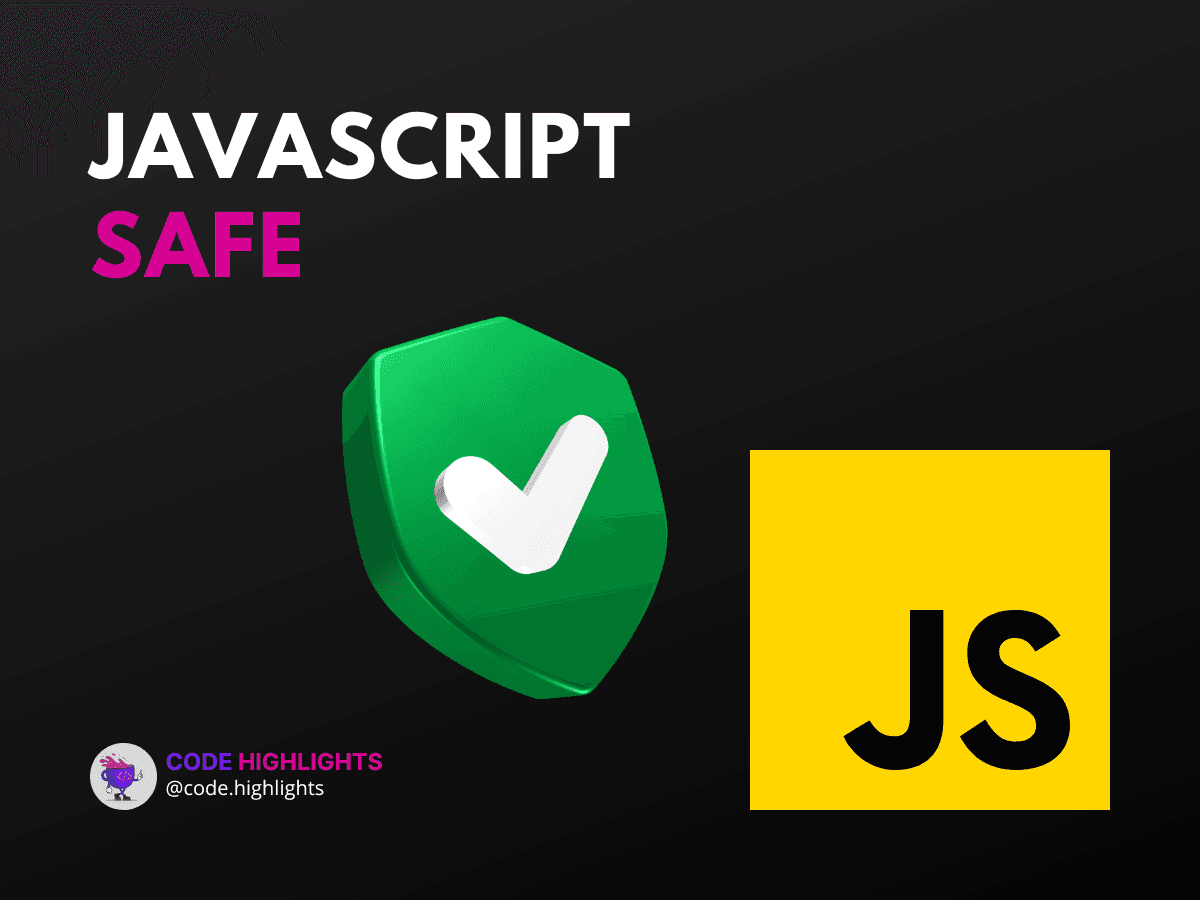
- Understanding JavaScript Safety
- Avoid Global Variables
- Validate User Input
- Use Modern JavaScript Features
- Tools and Libraries
- External Resources for Safe JavaScript Practices
Welcome to the world of JavaScript, where the possibilities are as vast as the potential pitfalls. As you embark on this journey, it's crucial to arm yourself with practices that ensure your code is not just functional but also secure. In this tutorial, we'll dive into the concept of JavaScript safe programming, exploring techniques that will help fortify your applications against common vulnerabilities. Let's kick things off with a simple code snippet that highlights the essence of writing safe JavaScript:
1// Example of using strict mode for safer JavaScript code
2'use strict';
3
4function safeFunction() {
5 // Code here is executed in strict mode
6}
By using 'use strict';
, we instruct the JavaScript engine to enforce stricter parsing and error handling, preventing common coding mistakes and "unsafe" actions. This is merely the tip of the iceberg when it comes to JavaScript safety.
Understanding JavaScript Safety
When we talk about JavaScript safety, we're referring to the practice of writing code that is robust against errors, bugs, and security vulnerabilities. This encompasses everything from avoiding global variables to preventing cross-site scripting (XSS) attacks. If you're new to JavaScript or need a refresher, consider taking a JavaScript course to solidify your understanding.
Avoid Global Variables
Global variables can lead to unpredictable behavior in your code. Instead, encapsulate your variables within functions or use block-level scoping with let
and const
:
1function exampleFunction() {
2 let localVariable = 'I am safe';
3 console.log(localVariable);
4}
5exampleFunction();
Validate User Input
Always validate and sanitize user input to prevent XSS and other injection attacks. If you're building web pages, a solid understanding of HTML and CSS is essential. Brush up on your skills with an HTML fundamentals course and a CSS introduction course.
Use Modern JavaScript Features
Modern JavaScript provides features that enhance security. For instance, template literals can reduce the risk of injection attacks:
1const userInput = '<script>alert("not safe")</script>';
2const safeOutput = `User input: ${userInput}`;
3console.log(safeOutput); // Outputs the user input safely
Tools and Libraries
Utilize libraries like DOMPurify to sanitize HTML content, and consider static analysis tools to catch vulnerabilities early. These are just a few steps towards safer JavaScript programming. For a comprehensive guide, check out an introduction to web development course.
External Resources for Safe JavaScript Practices
- Mozilla Developer Network - A trusted resource for web technologies, including JavaScript.
- OWASP Foundation - Learn about web security best practices from the Open Web Application Security Project.
- Google Developers - Google's resource hub for modern web development, including security considerations.
- Node.js Security Best Practices - If you're using JavaScript on the server-side with Node.js, these are essential reads.
- ECMA International - Understand the standards behind JavaScript for deeper insights into safe coding practices.
In conclusion, JavaScript safe programming is about being proactive and mindful of the code you write. By adopting best practices and leveraging modern JavaScript features, you ensure that your applications are not just powerful but also secure. Remember, the key to mastering JavaScript is continuous learning and application of knowledge. Happy coding, and stay safe!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
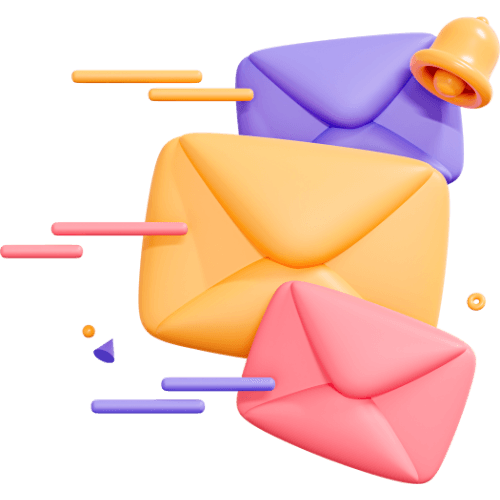
Related articles
125 Articles
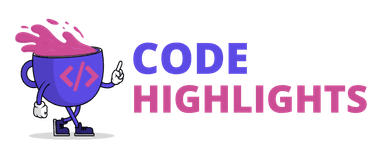
Copyright © Code Highlights 2024.