How to Use JavaScript Scroll to Element for Smooth Navigation

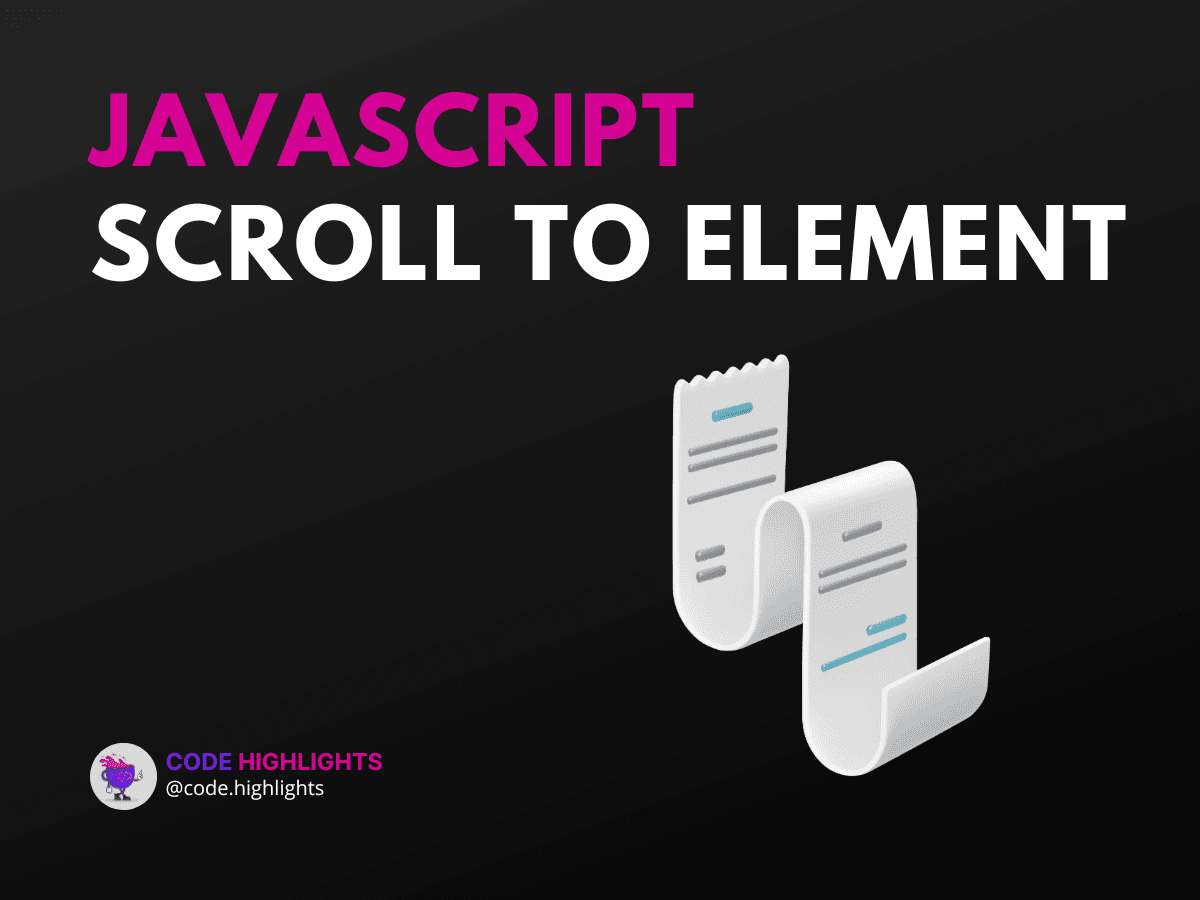
- Introduction Code Example
- Syntax and Parameters
- Parameters:
- Variations:
- Detailed Code Examples
- Example 1: Scroll to Specific Element
- Example 2: Scroll Within a Container
- Example 3: Scroll Until Element is Visible
- Browser Compatibility
- Summary
Navigating a web page can sometimes be cumbersome, especially when dealing with long content. Fortunately, JavaScript offers a handy method to scroll to specific elements, enhancing user experience with smooth navigation. In this tutorial, we'll explore how to use the scrollTo
method in JavaScript to achieve this.
Introduction Code Example
Here’s a quick example to show you how scrollTo
works:
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>Scroll to Element Example</title>
6</head>
7<body>
8 <button onclick="scrollToElement()">Scroll to Section</button>
9 <div style="height: 1000px;"></div>
10 <div id="target">Target Section</div>
11
12 <script>
13 function scrollToElement() {
14 document.getElementById('target').scrollIntoView({ behavior: 'smooth' });
15 }
16 </script>
17</body>
18</html>
In this example, clicking the button scrolls the page smoothly to the target section.
Syntax and Parameters
The scrollTo
method has a straightforward syntax:
Parameters:
- options: An object that defines the scrolling behavior. It includes:
- behavior: Defines the transition animation. Can be
'auto'
(default) or'smooth'
. - block: Defines vertical alignment. Can be
'start'
,'center'
,'end'
, or'nearest'
. - inline: Defines horizontal alignment. Can be
'start'
,'center'
,'end'
, or'nearest'
.
- behavior: Defines the transition animation. Can be
Variations:
Basic Scroll
Smooth Scroll
Detailed Code Examples
Example 1: Scroll to Specific Element
This example demonstrates how to scroll to a specific element on the page.
1<button onclick="scrollToElement()">Go to Content</button>
2<div style="height: 800px;"></div>
3<div id="content">Content Section</div>
4
5<script>
6 function scrollToElement() {
7 document.getElementById('content').scrollIntoView({ behavior: 'smooth' });
8 }
9</script>
Example 2: Scroll Within a Container
Learn how to scroll to an element inside a scrollable container.
1<div style="height: 200px; overflow-y: scroll;">
2 <div style="height: 600px;">
3 <div id="inner-content" style="margin-top: 500px;">Inner Content</div>
4 </div>
5</div>
6<button onclick="scrollToInnerElement()">Scroll to Inner Content</button>
7
8<script>
9 function scrollToInnerElement() {
10 document.getElementById('inner-content').scrollIntoView({ behavior: 'smooth' });
11 }
12</script>
Example 3: Scroll Until Element is Visible
This snippet ensures the element is visible by scrolling to it if it's not already in view.
1<button onclick="ensureVisible()">Ensure Visibility</button>
2<div style="height: 1500px;"></div>
3<div id="visible-section">Visible Section</div>
4
5<script>
6 function ensureVisible() {
7 const element = document.getElementById('visible-section');
8 if (!element.getBoundingClientRect().top < window.innerHeight && element.getBoundingClientRect().bottom >= 0) {
9 element.scrollIntoView({ behavior: 'smooth' });
10 }
11 }
12</script>
Browser Compatibility
The scrollIntoView
method is compatible with all modern browsers, including Chrome, Firefox, Safari, Edge, and Opera. For older versions of Internet Explorer, consider using polyfills or alternative methods.
Summary
In this tutorial, we've covered how to use JavaScript to scroll to specific elements, ensuring smooth navigation and improved user experience. From basic usage to advanced scenarios like scrolling within containers and ensuring visibility, these techniques are essential for modern web development. For more on JavaScript fundamentals, check out our JavaScript courses and web development introduction.
For further reading, consider exploring resources on MDN Web Docs, W3Schools, and CSS-Tricks.
By mastering these techniques, you can create more dynamic and user-friendly web pages, keeping your audience engaged and satisfied.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
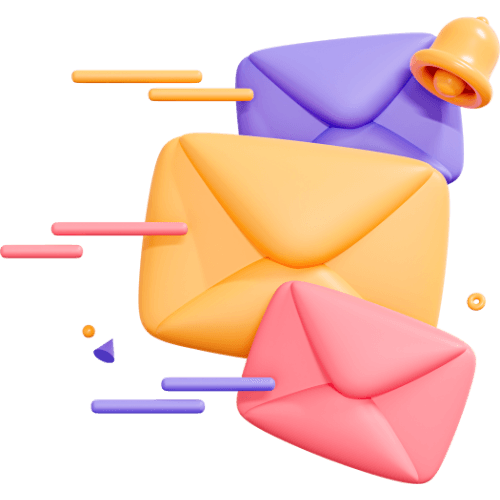
Related articles
9 Articles
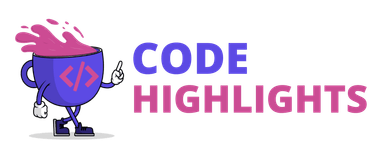
Copyright © Code Highlights 2025.