In Javascript Set to Array conversion - How to

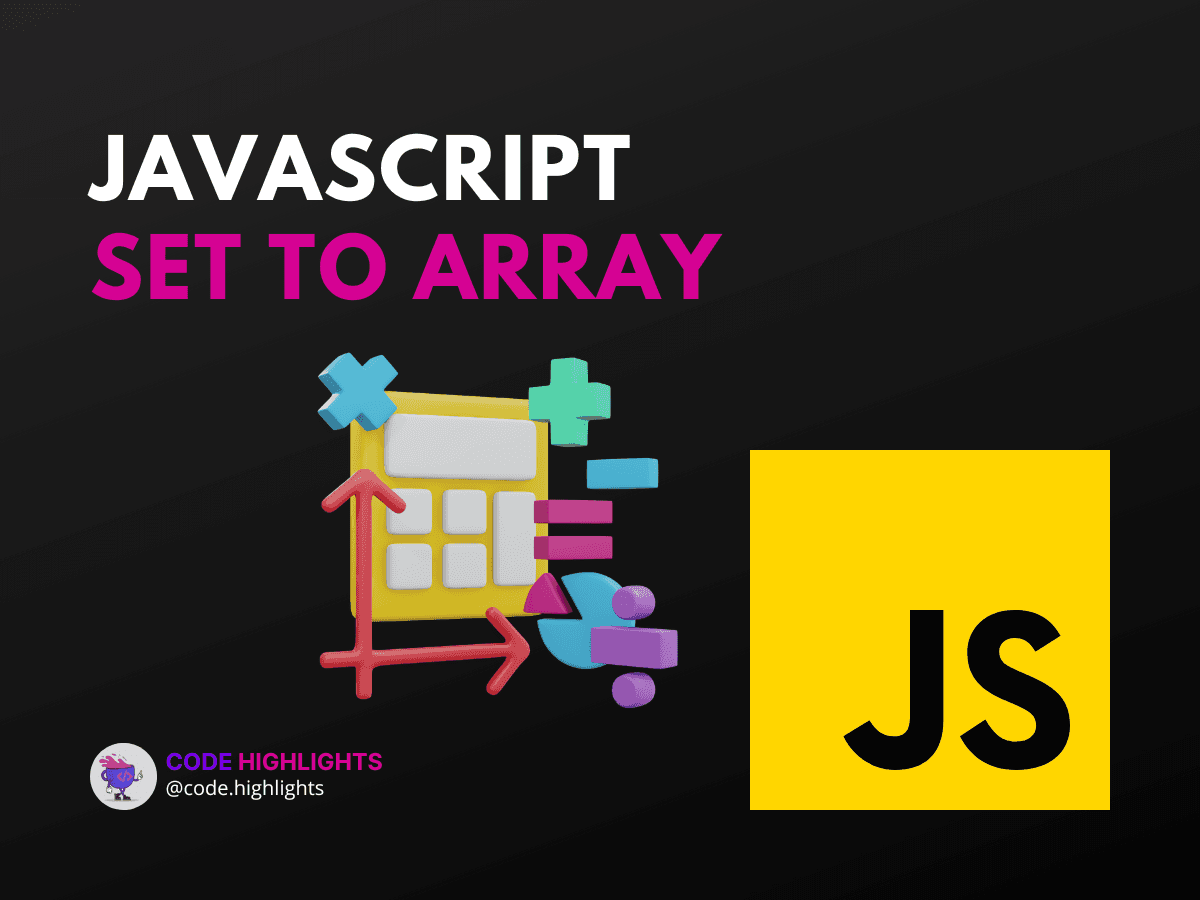
- Understanding Sets in JavaScript
- Converting Using Spread Syntax
- Utilizing Array.from Method
- Setting Object to Array
- Conclusion
JavaScript is a versatile language that often surprises us with its array of data structures and their utility. Among these structures, the Set
object lets you store unique values of any type. But what if you need to work with these values in an array format? In this tutorial, we'll explore how to transform a Set
into an array using JavaScript, ensuring you can manipulate your data exactly as you need.
1// Simple code example
2const mySet = new Set([1, 2, 3]);
3const myArray = [...mySet];
4console.log(myArray); // Output: [1, 2, 3]
This snippet showcases the syntax we'll delve into, providing a glimpse of the simplicity and elegance of JavaScript's capabilities. Whether you're honing your skills through a JavaScript course, or you're just starting with the fundamentals of HTML, understanding how to convert a Set
to an array is a valuable addition to your web development toolkit.
Understanding Sets in JavaScript
Before we dive into conversion techniques, let's briefly touch upon what a Set
is. A Set
is a collection of values without duplicates, making it perfect for cases where you need to ensure uniqueness. If you're coming from a background in CSS, think of a Set
as a way to apply a unique id selector, akin to learning about selectors in a CSS introduction course.
Converting Using Spread Syntax
One of the most straightforward ways to turn a Set
into an array in JavaScript is by using the spread syntax:
The spread syntax (...
) effectively 'spreads' the elements of the Set
into the array. This method is both concise and efficient, answering the question of how to assign an array to a Set
in JavaScript.
Utilizing Array.from Method
Alternatively, the Array.from
method offers a direct approach:
Array.from
creates a new, shallow-copied array instance from an array-like or iterable object, which includes our Set
. This method is particularly useful when you need to transform or map the elements during the conversion process.
Setting Object to Array
If you have a Set
of objects and you're pondering how to set the object to an array in JavaScript, these methods still apply. Whether it's a Set
of numbers or objects, the conversion process remains the same.
Conclusion
Converting a Set
to an array in JavaScript is an essential skill, especially when you're dealing with unique collections of data that you later need in an array format. We've covered two primary methods: using the spread syntax and the Array.from
method. Both are effective and can be used interchangeably based on your preference.
As you continue to build your web development proficiency, remember that these skills are part of a larger picture. Consider exploring an introduction to web development to broaden your understanding of how JavaScript interacts with HTML and CSS to create dynamic web experiences.
For further reading and best practices, make sure to check out external resources such as the Mozilla Developer Network (MDN), W3Schools, and Stack Overflow. These sites offer a wealth of information and community insight that can help solidify your understanding of JavaScript and its array of functionalities.
By now, you should feel confident in converting a Set
to an array in JavaScript, and you can incorporate this knowledge into your projects, ensuring you can handle data structures with ease. Keep practicing, keep learning, and don't forget to experiment with the code snippets provided to see firsthand how they work!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
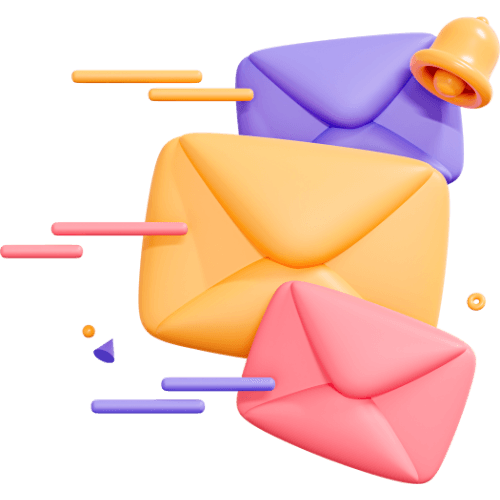
Related articles
114 Articles
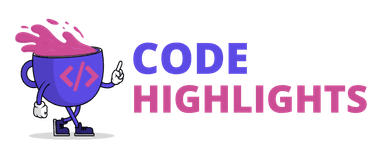
Copyright © Code Highlights 2024.