The Secret to Efficient JavaScript Sort by Boolean

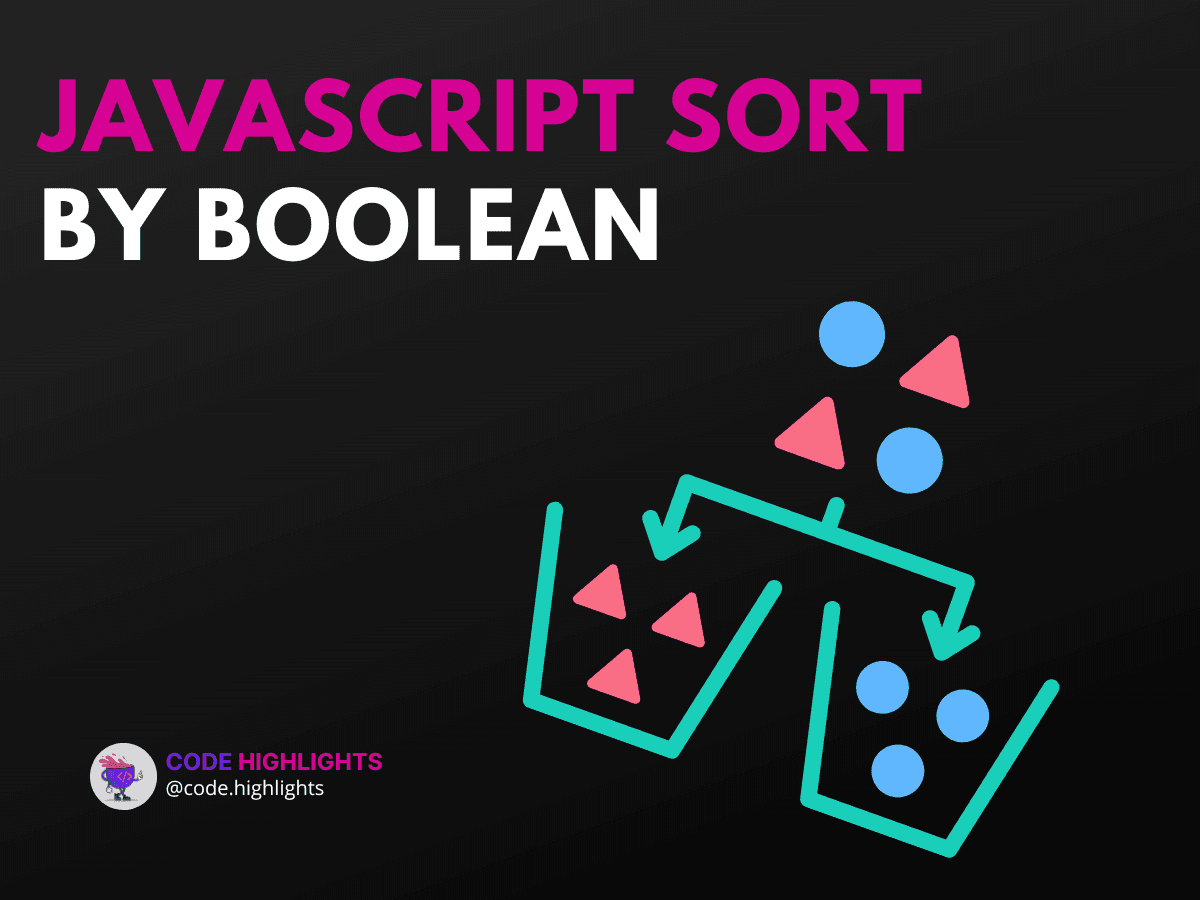
- Quick Code Example
- Understanding the Syntax
- Sorting by Boolean Values
- Example 1: Basic Boolean Sorting
- Sorting Active Users
- Example 2: Sorting with Multiple Criteria
- Sorting Products by Availability
- Example 3: Sorting Mixed Data Types
- Sorting Employees by Status
- Compatibility with Browsers
- Summary
Sorting data is a crucial task in programming. When dealing with arrays of objects, you might want to sort them based on boolean values. This can help you organize your data effectively. In this tutorial, we will explore how to use JavaScript to sort data by boolean values. We'll look at examples and explain the syntax clearly to ensure you understand every step.
Quick Code Example
Here's a quick snippet to show you how to sort an array of objects by a boolean property:
1const items = [
2 { name: 'Item 1', active: true },
3 { name: 'Item 2', active: false },
4 { name: 'Item 3', active: true }
5];
6
7items.sort((a, b) => a.active === b.active ? 0 : a.active ? -1 : 1);
8console.log(items);
This code sorts the items
array, putting all active items first. Let's dive deeper into the syntax and how it works.
Understanding the Syntax
The sort()
method in JavaScript sorts the elements of an array in place. It takes a comparison function as an argument. This function has two parameters, often named a
and b
. The return value determines their order:
- Return a negative number if
a
should come beforeb
. - Return zero if they are equal.
- Return a positive number if
b
should come beforea
.
Sorting by Boolean Values
When sorting by boolean values, you can use the following approach:
1array.sort((a, b) => {
2 return a.booleanProperty === b.booleanProperty ? 0 : a.booleanProperty ? -1 : 1;
3});
In this case, booleanProperty
is the property you want to sort by. This method ensures that all true
values come before false
values.
Example 1: Basic Boolean Sorting
Sorting Active Users
Let’s look at an example where we sort users by their active status:
1const users = [
2 { username: 'Alice', active: true },
3 { username: 'Bob', active: false },
4 { username: 'Charlie', active: true }
5];
6
7users.sort((a, b) => a.active === b.active ? 0 : a.active ? -1 : 1);
8console.log(users);
In this example, Alice and Charlie will appear before Bob since they are active. This is a simple yet effective way to manage user data.
Example 2: Sorting with Multiple Criteria
Sorting Products by Availability
You may also want to sort products based on their availability and then by price. Here’s how you can do that:
1const products = [
2 { name: 'Laptop', available: false, price: 1000 },
3 { name: 'Phone', available: true, price: 500 },
4 { name: 'Tablet', available: true, price: 300 }
5];
6
7products.sort((a, b) => {
8 if (a.available === b.available) {
9 return a.price - b.price; // Sort by price if availability is the same
10 }
11 return a.available ? -1 : 1; // Sort by availability
12});
13console.log(products);
This code first sorts by availability and then by price if the availability is the same. Such methods can be useful for e-commerce applications.
Example 3: Sorting Mixed Data Types
Sorting Employees by Status
Sometimes, your data may include mixed types. Here’s how to handle that:
1const employees = [
2 { name: 'John', active: true },
3 { name: 'Jane', active: null },
4 { name: 'Doe', active: false }
5];
6
7employees.sort((a, b) => {
8 if (a.active === b.active) return 0;
9 if (a.active === null) return 1; // Treat null as inactive
10 return a.active ? -1 : 1; // Active first
11});
12console.log(employees);
This example sorts employees, treating null
as inactive. This flexibility can be essential in real-world applications.
Compatibility with Browsers
The sort()
method is widely supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. You can use it confidently in your projects without worrying about compatibility issues.
Summary
In this tutorial, we learned how to sort data by boolean values in JavaScript. We covered the basic syntax, provided examples for sorting users, products, and employees, and discussed handling mixed data types. Sorting by boolean can help you quickly organize your data, making it easier to work with.
For further learning, check out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. If you're interested in web development, consider exploring our Introduction to Web Development course.
By mastering these sorting techniques, you'll improve your data management skills and enhance your programming capabilities!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
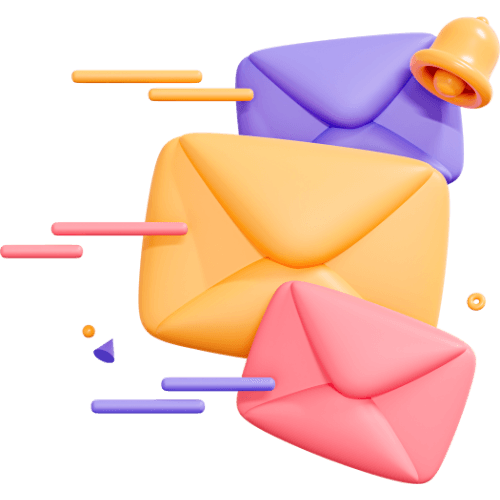
Related articles
9 Articles
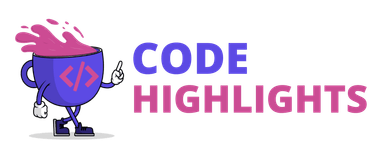
Copyright © Code Highlights 2025.