5 Essential JavaScript Sort by Multiple Fields Tricks to Master in 2024

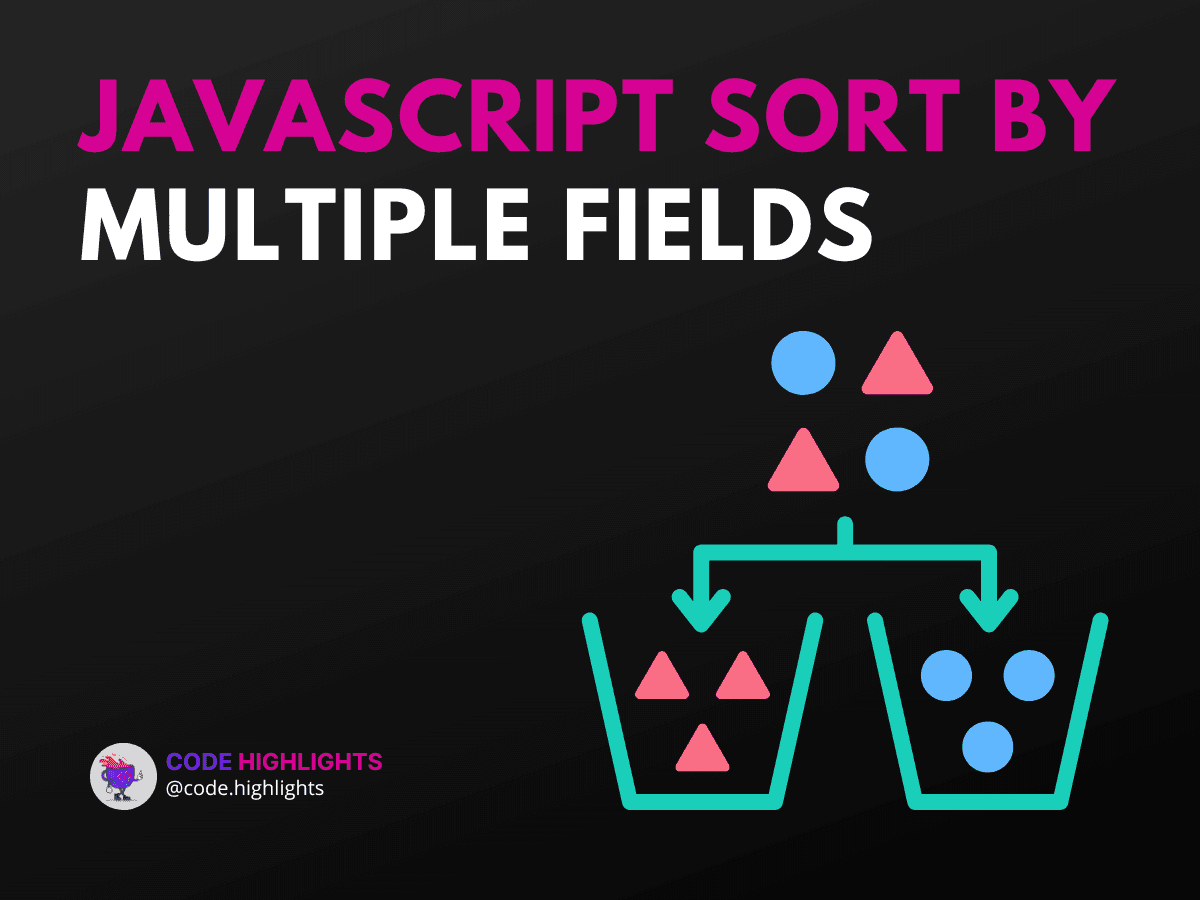
- Quick Code Example
- Understanding the Syntax
- Parameters
- Return Values
- Example 1: Sorting by Two Fields
- Sort by Age and then Name
- Example 2: Sorting by Multiple Criteria
- Sort by Category and Price
- Example 3: Sorting with Custom Logic
- Sort by Year and then Rating
- Example 4: Sorting by Nested Properties
- Sort by Author and then Title
- Example 5: Sorting with Dynamic Criteria
- Sort by User Input
- Browser Compatibility
- Key Takeaways
Sorting data is a fundamental concept in programming, especially in JavaScript. When you have an array of objects and want to sort them based on multiple fields, it can be tricky. This tutorial will guide you through five essential tricks to effectively javascript sort by multiple fields. By the end, you'll understand how to sort your data efficiently and apply these techniques in real-world scenarios.
Quick Code Example
Here's a quick example to get you started:
1const users = [
2 { name: 'Alice', age: 30 },
3 { name: 'Bob', age: 25 },
4 { name: 'Charlie', age: 30 },
5];
6
7users.sort((a, b) => {
8 if (a.age === b.age) {
9 return a.name.localeCompare(b.name);
10 }
11 return a.age - b.age;
12});
13
14console.log(users);
This code sorts users first by age and then by name. Let's dive deeper into the syntax and explore more examples.
Understanding the Syntax
The sort()
method in JavaScript sorts the elements of an array in place. It takes a comparison function as an argument. The comparison function should return:
- A negative number if the first argument comes before the second.
- A positive number if the first argument comes after the second.
- Zero if they are equal.
Parameters
- Comparison Function:
(a, b) => { /* comparison logic */ }
Return Values
- Negative Number:
a
comes beforeb
- Positive Number:
a
comes afterb
- Zero:
a
andb
are equal
Example 1: Sorting by Two Fields
Sort by Age and then Name
1const people = [
2 { name: 'John', age: 22 },
3 { name: 'Jane', age: 22 },
4 { name: 'Tom', age: 25 },
5];
6
7people.sort((a, b) => {
8 if (a.age === b.age) {
9 return a.name.localeCompare(b.name);
10 }
11 return a.age - b.age;
12});
13
14console.log(people);
In this example, we sort first by age and then alphabetically by name. This technique is useful when you want to maintain order among similar values.
Example 2: Sorting by Multiple Criteria
Sort by Category and Price
1const products = [
2 { category: 'Electronics', price: 100 },
3 { category: 'Clothing', price: 50 },
4 { category: 'Electronics', price: 80 },
5];
6
7products.sort((a, b) => {
8 if (a.category === b.category) {
9 return a.price - b.price;
10 }
11 return a.category.localeCompare(b.category);
12});
13
14console.log(products);
Here, we sort products first by category and then by price. This is handy for e-commerce applications where you need to display items in a structured way.
Example 3: Sorting with Custom Logic
Sort by Year and then Rating
1const movies = [
2 { title: 'Movie A', year: 2020, rating: 8.5 },
3 { title: 'Movie B', year: 2020, rating: 9.0 },
4 { title: 'Movie C', year: 2019, rating: 7.5 },
5];
6
7movies.sort((a, b) => {
8 if (a.year === b.year) {
9 return b.rating - a.rating; // Higher ratings first
10 }
11 return a.year - b.year;
12});
13
14console.log(movies);
This example sorts movies by year and then by rating, showcasing how to prioritize one field over another.
Example 4: Sorting by Nested Properties
Sort by Author and then Title
1const books = [
2 { author: { lastName: 'Smith', firstName: 'John' }, title: 'Book A' },
3 { author: { lastName: 'Doe', firstName: 'Jane' }, title: 'Book B' },
4 { author: { lastName: 'Smith', firstName: 'Alice' }, title: 'Book C' },
5];
6
7books.sort((a, b) => {
8 if (a.author.lastName === b.author.lastName) {
9 return a.author.firstName.localeCompare(b.author.firstName);
10 }
11 return a.author.lastName.localeCompare(b.author.lastName);
12});
13
14console.log(books);
In this case, we sort books by the author's last name and then by their first name. This is common in libraries and book collections.
Example 5: Sorting with Dynamic Criteria
Sort by User Input
1const data = [
2 { name: 'Zoe', score: 90 },
3 { name: 'Alex', score: 85 },
4 { name: 'Mia', score: 95 },
5];
6
7function dynamicSort(property) {
8 return (a, b) => {
9 return a[property] < b[property] ? -1 : a[property] > b[property] ? 1 : 0;
10 };
11}
12
13data.sort(dynamicSort('score'));
14console.log(data);
This example shows how to sort based on user-defined criteria, making your application more flexible.
Browser Compatibility
Most modern browsers, including Chrome, Firefox, Safari, and Edge, support the sort()
method. However, always check compatibility if you're working with older browsers. For more details, visit MDN Web Docs.
Key Takeaways
In this tutorial, we've explored how to javascript sort by multiple fields using various examples. Sorting by multiple criteria is essential for organizing data effectively. Whether you're sorting users, products, or any other data, understanding these techniques will help you create better applications.
For further learning, consider checking out our courses on HTML Fundamentals and Introduction to Web Development.
Master these tricks, and you'll be well on your way to becoming proficient in JavaScript!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
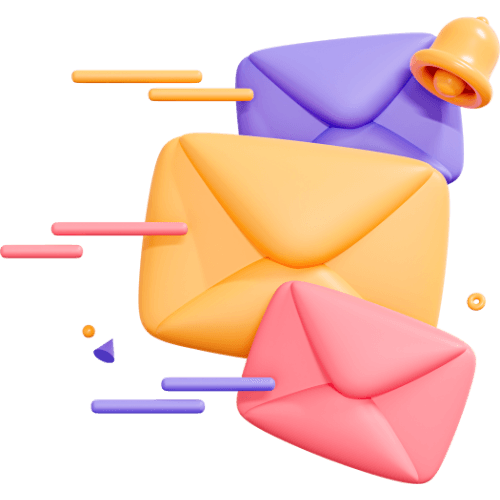
Related articles
9 Articles
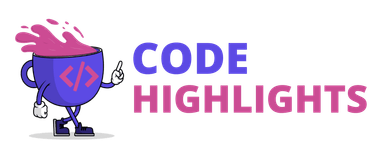
Copyright © Code Highlights 2024.