How to Resolve 'Javascript Split is Not a Function' Quickly

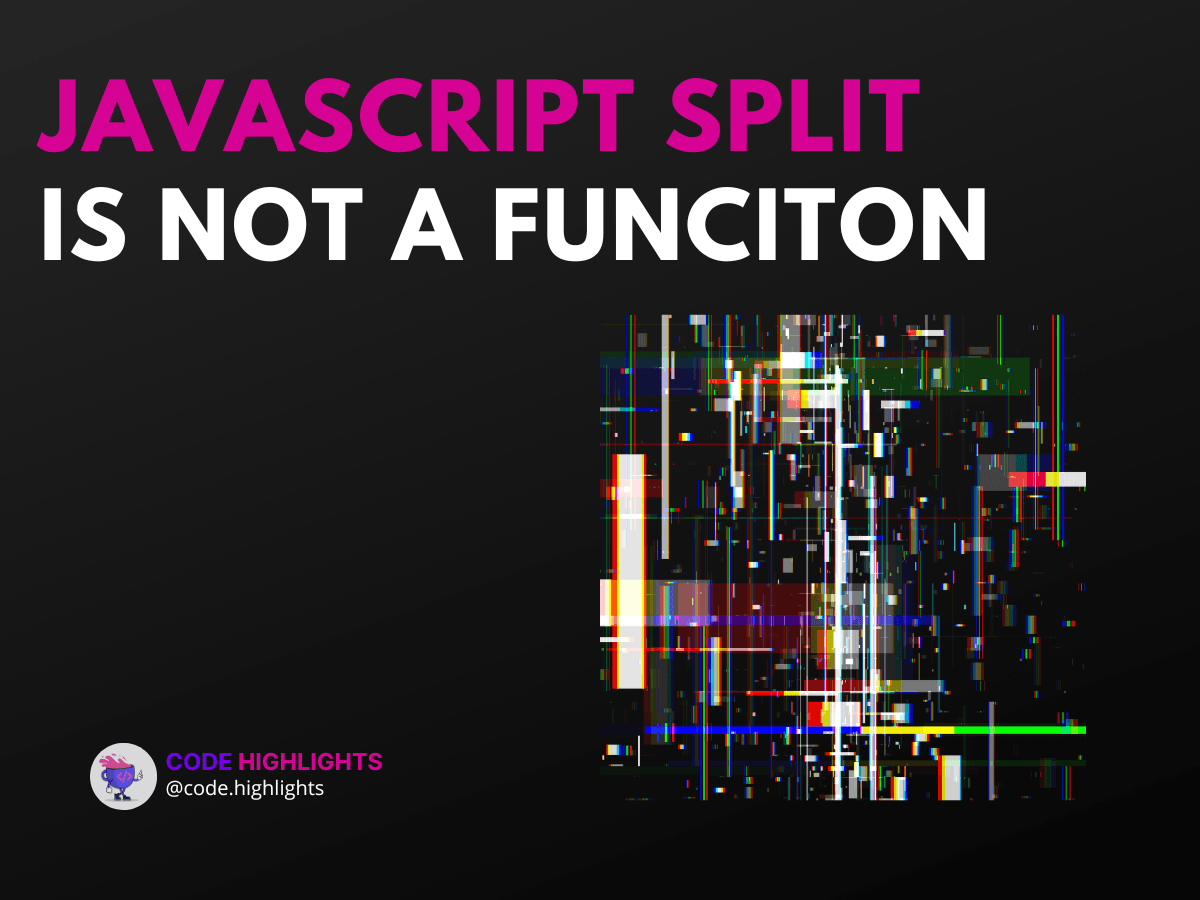
- Quick Code Example
- Understanding the .split() Method
- Return Value
- Syntax Variations
- Resolving the Error
- Example: Checking Variable Type
- Compatibility with Browsers
- Summary
If you've ever encountered the error message javascript split is not a function
, it can be frustrating. This issue typically arises when you try to use the .split()
method on a variable that isn't a string. In this tutorial, we will explore how to fix this error and ensure your code runs smoothly.
Quick Code Example
Here's a simple example to illustrate the problem:
In this code, we attempt to call .split()
on a number, which leads to the error. Let's dive deeper into understanding the .split()
method and how to resolve this issue.
Understanding the .split()
Method
The .split()
method is used to divide a string into an array of substrings based on a specified delimiter. The syntax for the method is as follows:
- separator: This is the character or regular expression that defines where the string should be split.
- limit (optional): This specifies the maximum number of splits to be found.
Return Value
The method returns an array containing the substrings. If the separator is not found, the original string is returned in an array.
Syntax Variations
Here are some variations of how you can use the .split()
method:
- Using a Comma as a Separator
1let fruits = "apple,banana,cherry";
2let fruitArray = fruits.split(',');
3console.log(fruitArray); // ['apple', 'banana', 'cherry']
- Using a Space as a Separator
1let sentence = "Hello world!";
2let words = sentence.split(' ');
3console.log(words); // ['Hello', 'world!']
- Using a Regular Expression
1let mixed = "one1two2three3four";
2let numbers = mixed.split(/\d/);
3console.log(numbers); // ['one', 'two', 'three', 'four']
Resolving the Error
To fix the javascript split is not a function
error, make sure the variable you're trying to split is indeed a string. Here’s how to check and convert a variable before using .split()
:
Example: Checking Variable Type
1let myVar = 12345;
2
3if (typeof myVar === 'string') {
4 let result = myVar.split('');
5} else {
6 myVar = String(myVar); // Convert to string
7 let result = myVar.split('');
8}
This code checks the type of myVar
. If it's not a string, it converts it to one before applying .split()
.
Compatibility with Browsers
The .split()
method is widely supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. You should not encounter compatibility issues when using this method in your web applications.
Summary
In this tutorial, we covered how to resolve the javascript split is not a function
error effectively. We learned about the .split()
method, its syntax, and variations. Additionally, we discussed how to ensure the variable is a string before using the method.
For more detailed learning, check out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. These resources will help you strengthen your web development skills.
Remember, always verify the type of your variables to avoid common errors like this one. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
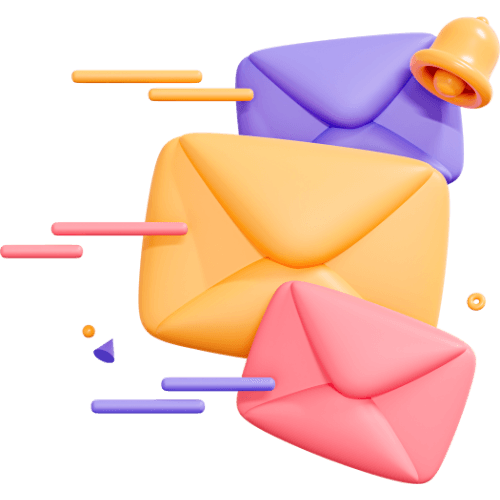
Related articles
9 Articles
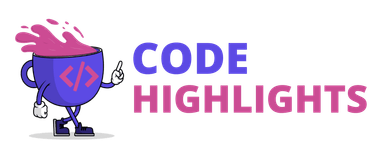
Copyright © Code Highlights 2025.