7 Easy Ways to JavaScript String Compare Case Insensitive

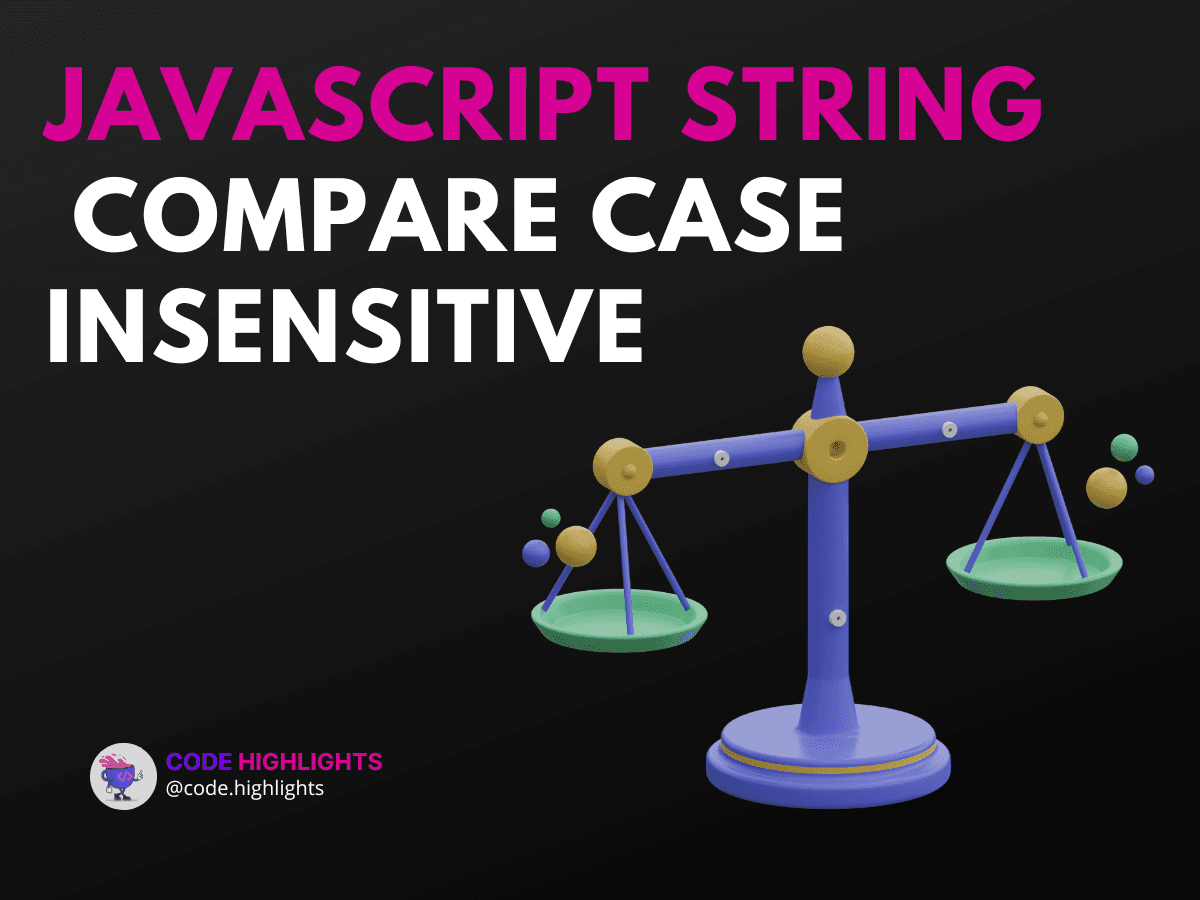
- Quick Example
- Method 1: Using toLowerCase()
- Explanation
- Method 2: Using toUpperCase()
- Explanation
- Method 3: Regular Expressions
- Explanation
- Method 4: Locale-Sensitive Comparison
- Explanation
- Method 5: Custom Function
- Explanation
- Method 6: Using String.prototype.includes()
- Explanation
- Method 7: Using String.prototype.indexOf()
- Explanation
- Browser Compatibility
- Summary
When working with strings in JavaScript, you might need to compare them without worrying about uppercase or lowercase letters. This is known as case-insensitive comparison. Understanding how to do this can help you build more flexible applications. Let’s dive into some easy methods to compare strings in a case-insensitive manner.
Quick Example
Here’s a quick example to get you started. This snippet checks if two strings are equal, ignoring their case:
1const str1 = "Hello World";
2const str2 = "hello world";
3
4const areEqual = str1.toLowerCase() === str2.toLowerCase();
5console.log(areEqual); // Output: true
Method 1: Using toLowerCase()
The simplest way to compare strings is by converting both to lowercase. This makes the comparison straightforward.
1const strA = "JavaScript";
2const strB = "javascript";
3
4if (strA.toLowerCase() === strB.toLowerCase()) {
5 console.log("Strings are equal!");
6}
Explanation
- Parameters: None.
- Return Value: A boolean indicating whether the strings are equal.
Method 2: Using toUpperCase()
Similar to toLowerCase()
, you can also convert both strings to uppercase.
1const str1 = "Hello";
2const str2 = "HELLO";
3
4if (str1.toUpperCase() === str2.toUpperCase()) {
5 console.log("They match!");
6}
Explanation
- Parameters: None.
- Return Value: A boolean indicating equality.
Method 3: Regular Expressions
You can use regular expressions for a more powerful comparison.
1const regex = new RegExp("hello", "i"); // 'i' makes it case insensitive
2const text = "Hello there!";
3
4if (regex.test(text)) {
5 console.log("Substring found!");
6}
Explanation
- Parameters: The string to search for and the flag
'i'
. - Return Value: A boolean indicating if the substring exists.
Method 4: Locale-Sensitive Comparison
JavaScript provides the localeCompare
method, which allows for locale-sensitive string comparison.
1const str1 = "éclair";
2const str2 = "ECLAIR";
3
4if (str1.localeCompare(str2, undefined, { sensitivity: 'accent' }) === 0) {
5 console.log("Strings are equal!");
6}
Explanation
- Parameters: Locale and options object.
- Return Value: A number indicating the comparison result.
Method 5: Custom Function
Creating a custom function can streamline your comparisons.
1function compareStrings(str1, str2) {
2 return str1.toLowerCase() === str2.toLowerCase();
3}
4
5console.log(compareStrings("Test", "test")); // Output: true
Explanation
- Parameters: Two strings to compare.
- Return Value: A boolean indicating equality.
Method 6: Using String.prototype.includes()
Check if a string contains another string, ignoring case.
1const mainStr = "JavaScript is fun!";
2const subStr = "javascript";
3
4if (mainStr.toLowerCase().includes(subStr.toLowerCase())) {
5 console.log("Substring exists!");
6}
Explanation
- Parameters: The substring to search for.
- Return Value: A boolean indicating presence.
Method 7: Using String.prototype.indexOf()
Older but still effective, indexOf()
can check for substrings.
1const phrase = "Learning JavaScript";
2const keyword = "javascript";
3
4if (phrase.toLowerCase().indexOf(keyword.toLowerCase()) !== -1) {
5 console.log("Found it!");
6}
Explanation
- Parameters: The substring to find.
- Return Value: The index of the substring or -1 if not found.
Browser Compatibility
All methods mentioned are widely supported across major browsers, including Chrome, Firefox, Safari, and Edge. For the best results, always test your code in different environments.
Summary
In this tutorial, we explored 7 easy ways to compare strings in JavaScript without considering case sensitivity. From using toLowerCase()
and toUpperCase()
to leveraging regular expressions and localeCompare
, you now have a variety of tools at your disposal. These methods will help you ensure that your string comparisons are accurate and effective.
For more on JavaScript, consider checking out our JavaScript course. If you're looking to enhance your web skills, our HTML Fundamentals Course and CSS Introduction are great resources. Finally, if you want to start from scratch, our Introduction to Web Development course is perfect for beginners!
By mastering these techniques, you can make your JavaScript applications more robust and user-friendly. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
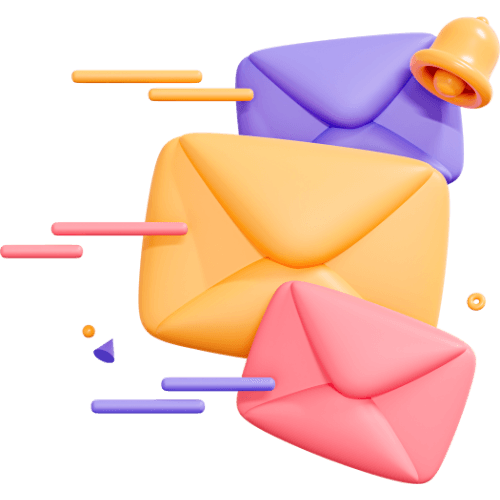
Related articles
9 Articles
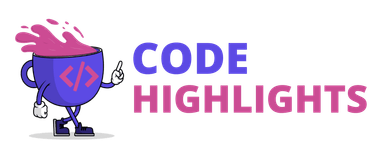
Copyright © Code Highlights 2025.