5 Quick Tips to Javascript Sum Array Like a Pro

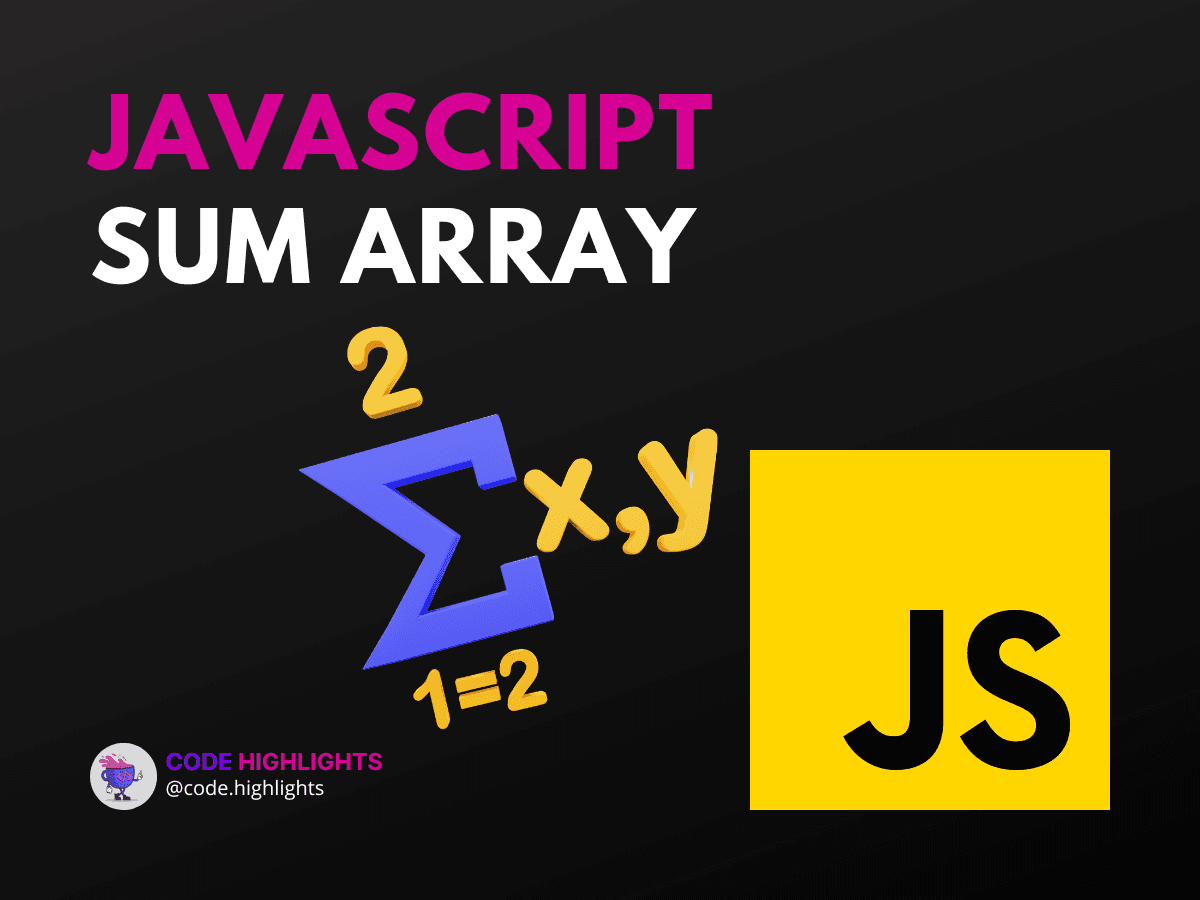
- Syntax Deep Dive: Understanding the JavaScript Sum Array Technique
- Practical Examples: JavaScript Sum Array in Action
- Example 1: Basic Summation of Array Elements
- Example 2: Summing Even Numbers Only
- Example 3: Combining Two Arrays Before Summing
- Browser Compatibility: Ensuring Universal Functionality
- Wrapping Up: The Power of Array Summation
Arrays are the treasure chests of JavaScript, holding precious data that can be manipulated in countless ways. One common task is summing up all the elements within an array—a fundamental operation that can reveal insights or calculate results dynamically. Let's dive right into a simple yet powerful example that showcases how you can quickly sum an array in JavaScript.
1let numbers = [1, 2, 3, 4, 5];
2let sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
3console.log(sum); // Output: 15
Syntax Deep Dive: Understanding the JavaScript Sum Array Technique
To perform an array summation in JavaScript, the reduce()
method is your trusty ally. This method takes a callback function with two parameters: the accumulator
(the accumulated value previously returned in the last invocation) and the currentValue
(the current element being processed).
Variations of this syntax allow for additional parameters, such as currentIndex
and array
, but let's keep our focus on summing values for now.
Practical Examples: JavaScript Sum Array in Action
Example 1: Basic Summation of Array Elements
Here's how to sum items in an array using reduce()
:
1let prices = [19.99, 45.49, 23.50];
2let total = prices.reduce((total, price) => total + price, 0);
3console.log(total); // Output: 88.98
Example 2: Summing Even Numbers Only
Filter out odd numbers first, then sum the evens:
1let mixedNumbers = [1, 2, 3, 4, 5, 6];
2let evenSum = mixedNumbers
3 .filter(number => number % 2 === 0)
4 .reduce((sum, number) => sum + number, 0);
5console.log(evenSum); // Output: 12
Example 3: Combining Two Arrays Before Summing
Concatenate two arrays, then find their sum:
1let array1 = [10, 20];
2let array2 = [30, 40];
3let combinedSum = array1.concat(array2).reduce((sum, number) => sum + number, 0);
4console.log(combinedSum); // Output: 100
Browser Compatibility: Ensuring Universal Functionality
The reduce()
method works seamlessly across all major web browsers, including Chrome, Firefox, Safari, and Edge. This ensures your JavaScript sum array operations run smoothly regardless of your audience's browser choice.
Wrapping Up: The Power of Array Summation
In conclusion, summing array elements in JavaScript is a versatile tool that can be adapted to various scenarios, from calculating totals to combining datasets. By mastering the reduce()
method and its applications, you'll unlock new possibilities in your web development projects. Remember to check out our JavaScript course to deepen your understanding, and explore our HTML fundamentals course, CSS introduction, and web development introduction to become a well-rounded web developer.
For further reading on JavaScript arrays and other related topics, consider visiting these reputable sources:
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
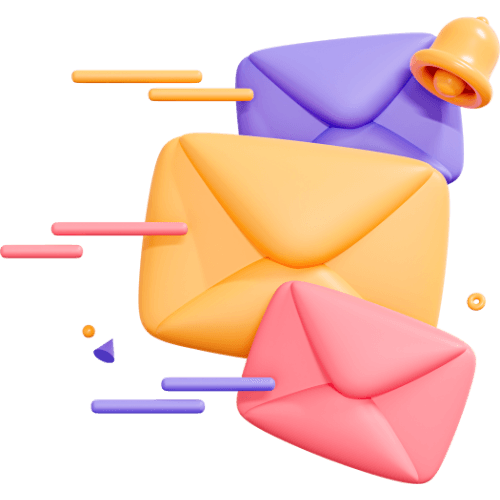
Related articles
9 Articles
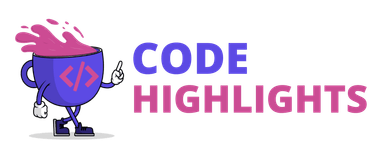
Copyright © Code Highlights 2025.