7 Simple JavaScript Swap Array Elements Tricks for Beginners

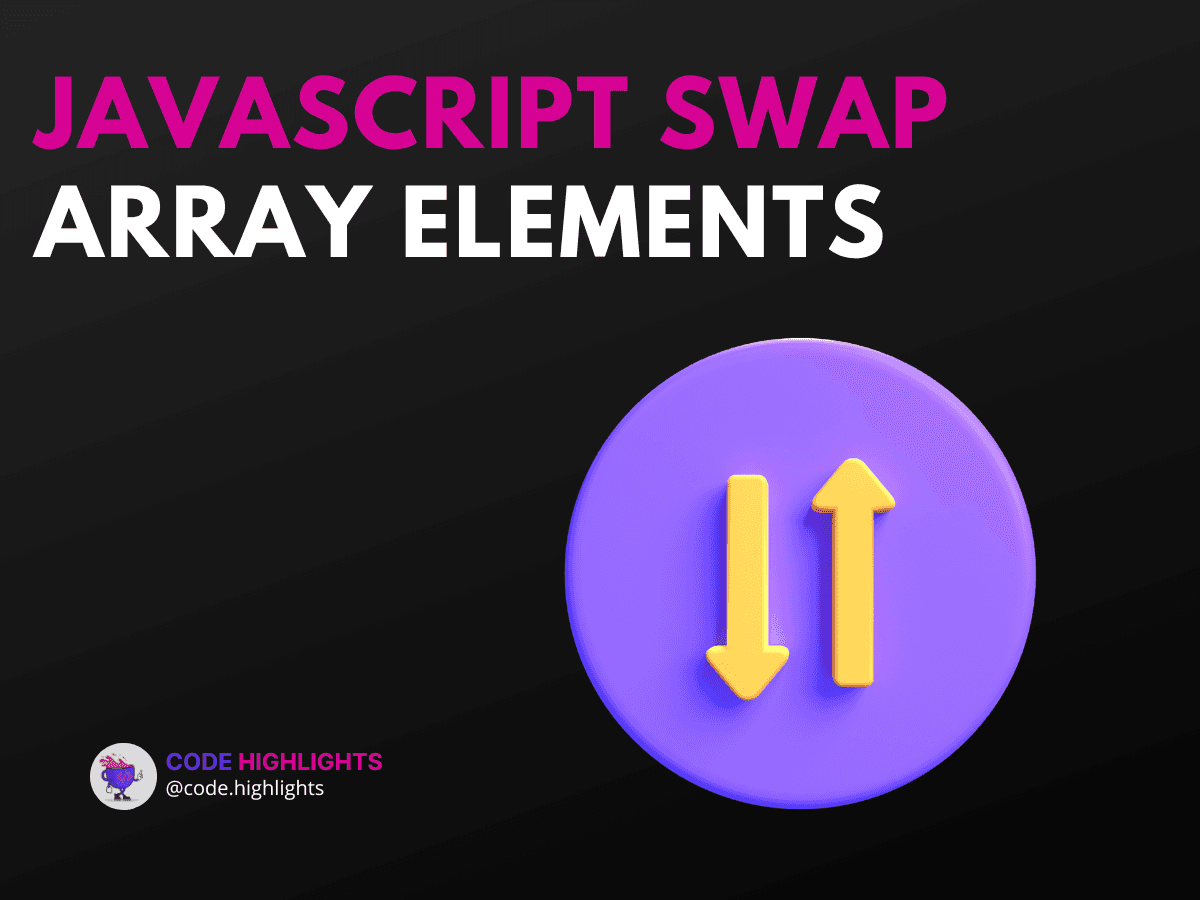
- Quick Code Example
- Understanding the Syntax
- Parameters and Return Values
- Example 1: Using a Temporary Variable
- Swapping with a Temporary Variable
- Example 2: Using Array Destructuring
- Swapping with Destructuring Assignment
- Example 3: Using a Function
- Creating a Swap Function
- Example 4: Swapping Without a Temporary Variable
- Swapping Using Arithmetic Operations
- Example 5: Using the Spread Operator
- Swapping with the Spread Operator
- Browser Compatibility
- Summary
Swapping elements in an array is a common task in programming. In JavaScript, understanding how to manipulate arrays can enhance your coding skills and help you solve various problems more efficiently. This tutorial will explore JavaScript swap array elements techniques that are perfect for beginners. We'll cover essential syntax, practical examples, and tips to make your coding journey smoother.
Quick Code Example
Here's a quick look at how to swap two elements in an array:
1let arr = [1, 2, 3, 4];
2let temp = arr[0];
3arr[0] = arr[1];
4arr[1] = temp;
5console.log(arr); // Output: [2, 1, 3, 4]
This example shows the basic idea of swapping using a temporary variable. Now, let's dive deeper into different ways to achieve this!
Understanding the Syntax
When swapping array elements, you typically use indices to identify the positions of the elements you want to exchange. The general syntax looks like this:
Parameters and Return Values
- array: The array you are working with.
- index1: The first index of the element to swap.
- index2: The second index of the element to swap.
The return value is the modified array with the elements swapped.
Example 1: Using a Temporary Variable
Swapping with a Temporary Variable
1let fruits = ['apple', 'banana'];
2let temp = fruits[0];
3fruits[0] = fruits[1];
4fruits[1] = temp;
5console.log(fruits); // Output: ['banana', 'apple']
In this example, we swap 'apple' and 'banana' using a temporary variable. This method is straightforward and easy to understand.
Example 2: Using Array Destructuring
Swapping with Destructuring Assignment
1let numbers = [10, 20];
2[numbers[0], numbers[1]] = [numbers[1], numbers[0]];
3console.log(numbers); // Output: [20, 10]
Array destructuring allows us to swap elements in a single line. It's a modern JavaScript feature that makes the code cleaner.
Example 3: Using a Function
Creating a Swap Function
1function swapElements(arr, index1, index2) {
2 let temp = arr[index1];
3 arr[index1] = arr[index2];
4 arr[index2] = temp;
5}
6
7let myArray = [5, 15, 25];
8swapElements(myArray, 0, 2);
9console.log(myArray); // Output: [25, 15, 5]
Here, we define a function to swap elements. This approach is reusable and keeps your code organized.
Example 4: Swapping Without a Temporary Variable
Swapping Using Arithmetic Operations
1let values = [3, 8];
2values[0] = values[0] + values[1];
3values[1] = values[0] - values[1];
4values[0] = values[0] - values[1];
5console.log(values); // Output: [8, 3]
This method uses arithmetic operations to swap values without a temporary variable. However, be cautious as it may lead to overflow issues with large numbers.
Example 5: Using the Spread Operator
Swapping with the Spread Operator
1let arr = [1, 2, 3];
2let newArr = [...arr.slice(0, 1), arr[2], arr[1]];
3console.log(newArr); // Output: [1, 3, 2]
Using the spread operator, we create a new array with swapped elements. This method is functional and avoids mutating the original array.
Browser Compatibility
All the methods discussed are compatible with major web browsers, including Chrome, Firefox, Safari, and Edge. For more details on browser compatibility, check out MDN Web Docs.
Summary
In this tutorial, we've covered seven simple tricks for JavaScript swap array elements. From using a temporary variable to leveraging modern features like destructuring and the spread operator, these techniques will help you manipulate arrays effectively. Understanding these concepts is crucial for any aspiring developer.
If you're looking to expand your knowledge further, consider exploring our courses on JavaScript, HTML Fundamentals, and CSS Introduction. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
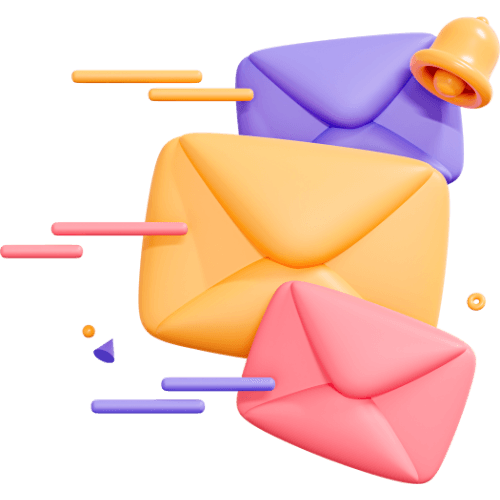
Related articles
9 Articles
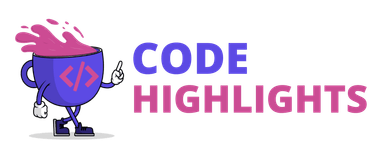
Copyright © Code Highlights 2025.