How to Use JavaScript Toggle Boolean for Better User Experience

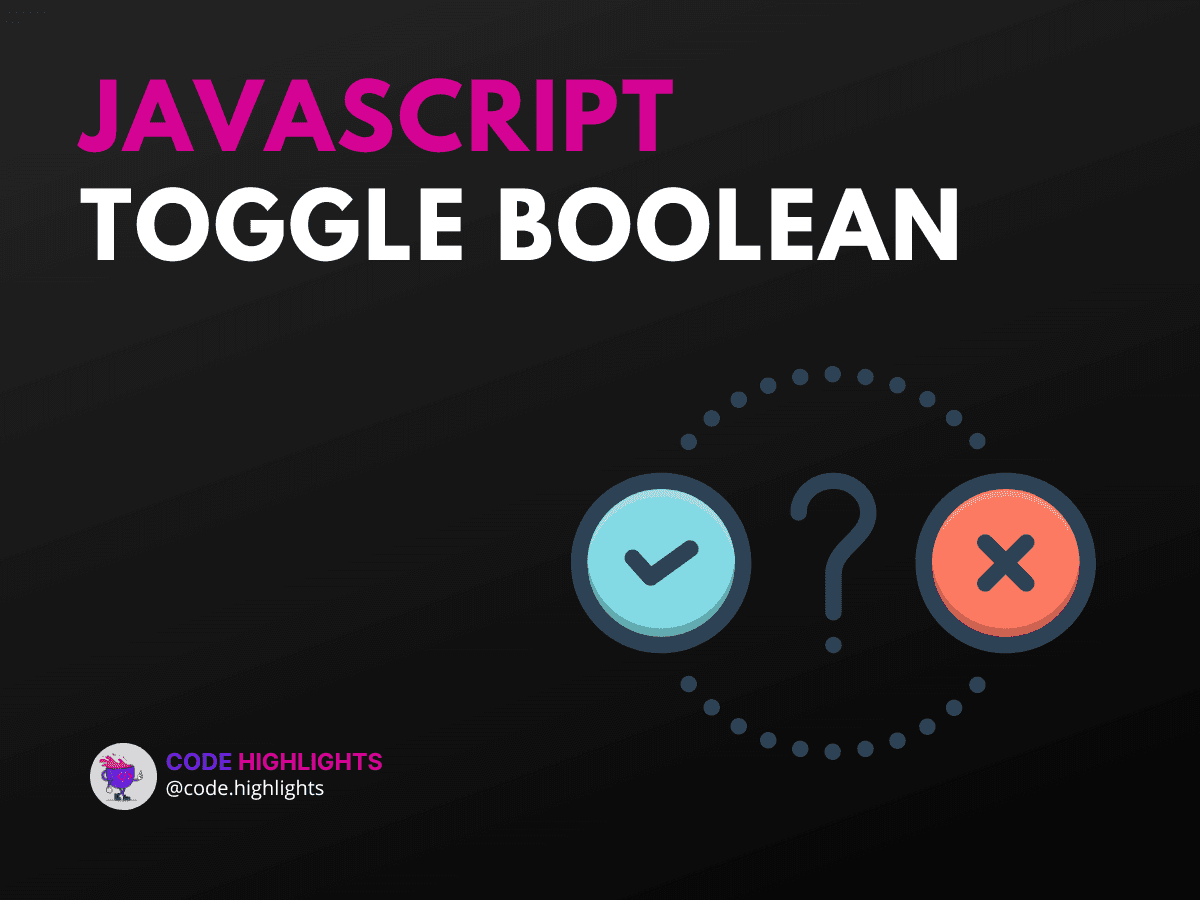
- Quick Code Example
- Understanding the Syntax
- Parameters and Return Values
- Variations in Syntax
- Example 1: Toggle Button Visibility
- Description
- Explanation
- Example 2: Toggle Class for Styling
- Description
- Explanation
- Example 3: Toggle Dark Mode
- Description
- Explanation
- Browser Compatibility
- Conclusion
Creating a smooth user experience on your website is essential. One way to achieve this is by using a JavaScript toggle boolean. This technique allows you to switch between two states, like showing and hiding elements. In this tutorial, we'll explore how to implement this concept effectively.
Quick Code Example
Here's a simple code snippet to get you started:
1let isVisible = false;
2
3function toggleVisibility() {
4 isVisible = !isVisible;
5 const element = document.getElementById("myElement");
6 element.style.display = isVisible ? "block" : "none";
7}
This example toggles the visibility of an HTML element by changing its display property.
Understanding the Syntax
The syntax for a toggle boolean in JavaScript is straightforward. You typically use a variable that holds a boolean value (true
or false
). When you call a function, it flips the value of this variable and performs an action based on the new value.
Parameters and Return Values
- Parameters: Often, you won't need to pass parameters directly to the toggle function. Instead, it uses a predefined variable.
- Return Values: The function usually doesn’t return a value; it simply updates the state of the UI.
Variations in Syntax
You can also use arrow functions or event listeners to create a toggle effect. Here’s a variation using an arrow function:
1let isActive = false;
2
3const toggleActiveState = () => {
4 isActive = !isActive;
5 console.log(isActive);
6};
Example 1: Toggle Button Visibility
Description
In this example, we will create a button that shows or hides a message when clicked.
1<button onclick="toggleVisibility()">Toggle Message</button>
2<div id="myElement" style="display:none;">Hello, World!</div>
Explanation
When the button is clicked, the toggleVisibility
function is called, which changes the display property of the myElement
div.
Example 2: Toggle Class for Styling
Description
This example demonstrates how to toggle a class for styling purposes.
1<button onclick="toggleClass()">Toggle Class</button>
2<div id="myDiv" class="box">Style Me!</div>
1function toggleClass() {
2 const div = document.getElementById("myDiv");
3 div.classList.toggle("active");
4}
Explanation
The toggleClass
function adds or removes the active
class from myDiv
, which can change its appearance based on CSS rules.
Example 3: Toggle Dark Mode
Description
Here’s how you can use a toggle boolean to switch between light and dark mode.
1<button onclick="toggleDarkMode()">Toggle Dark Mode</button>
1let isDarkMode = false;
2
3function toggleDarkMode() {
4 isDarkMode = !isDarkMode;
5 document.body.className = isDarkMode ? "dark-mode" : "";
6}
Explanation
This function changes the body class to apply different styles for dark mode.
Browser Compatibility
The JavaScript toggle boolean method works well across all major web browsers, including Chrome, Firefox, Safari, and Edge. However, always ensure your scripts are tested in various environments for consistent behavior.
Conclusion
In this tutorial, we explored how to use a JavaScript toggle boolean to enhance user experience. We covered basic syntax, parameters, and several practical examples. Whether you're toggling visibility, classes, or even themes, this technique is valuable for making your web applications more interactive.
For more hands-on learning, check out our HTML Fundamentals Course or Introduction to Web Development.
If you're interested in deepening your JavaScript knowledge, consider our Learn JavaScript course.
For styling elements with CSS, visit our Learn CSS Introduction course.
Feel free to explore further resources on MDN Web Docs or W3Schools for additional insights into JavaScript functionalities.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
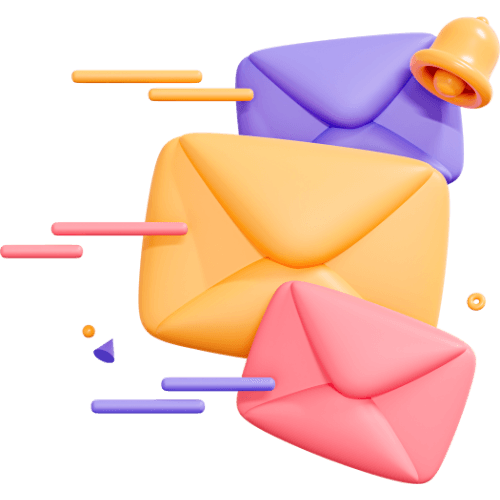
Related articles
9 Articles
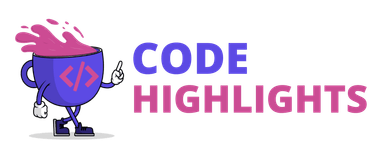
Copyright © Code Highlights 2024.