How to Choose JavaScript Tools for Maximum Efficiency

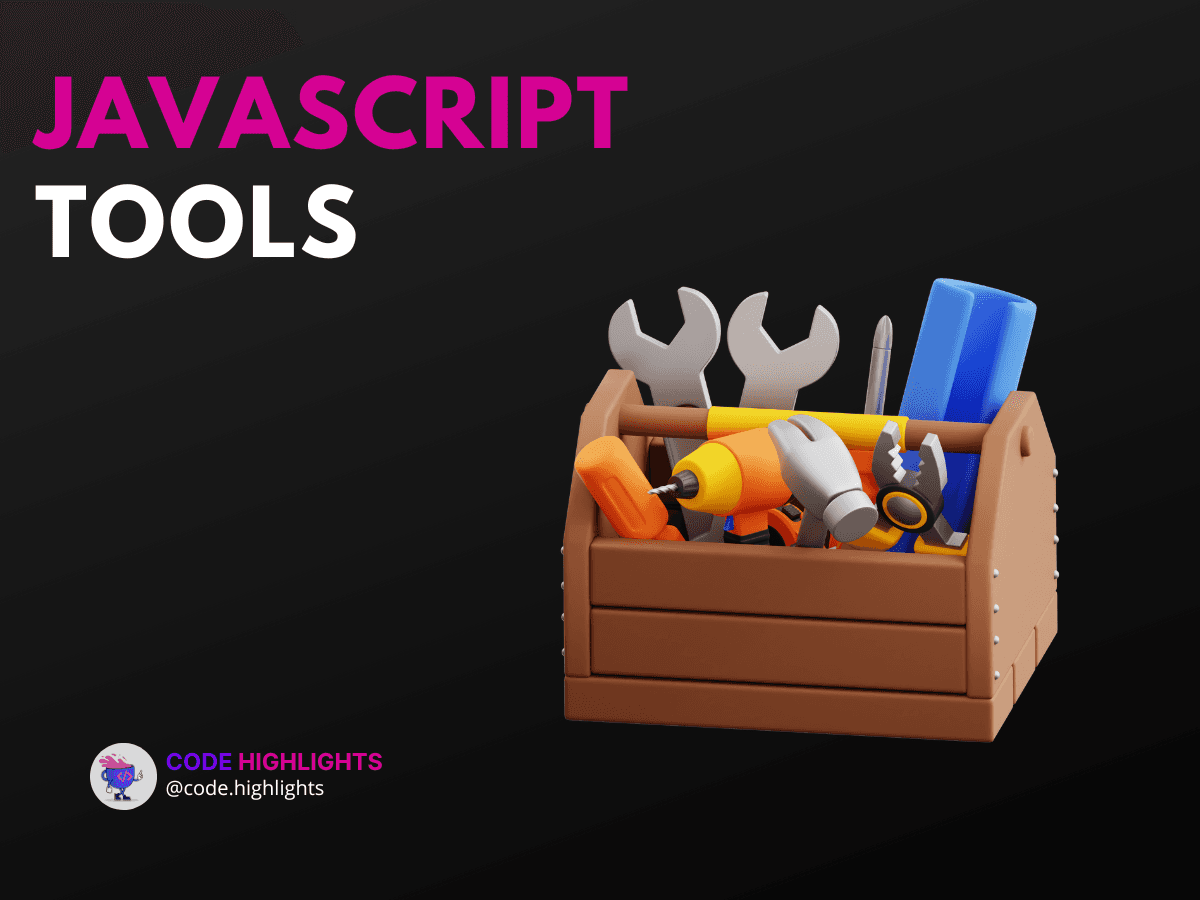
- Quick Code Example
- Understanding JavaScript Tools
- Key Parameters and Return Values
- Example 1: Using jQuery for DOM Manipulation
- Example 2: Building a Simple App with React
- Example 3: Setting Up a Development Environment with Visual Studio Code
- Browser Compatibility
- Conclusion
Choosing the right JavaScript tools can greatly enhance your coding experience and productivity. With so many options available, it can be overwhelming to decide which tools are best for your projects. This guide will help you understand the essential tools available and how to pick the ones that suit your needs.
Quick Code Example
Before diving in, here’s a quick example of using a popular JavaScript tool, Node.js.
1const http = require('http');
2
3const server = http.createServer((req, res) => {
4 res.statusCode = 200;
5 res.setHeader('Content-Type', 'text/plain');
6 res.end('Hello World\n');
7});
8
9server.listen(3000, () => {
10 console.log('Server running at http://localhost:3000/');
11});
In this example, we create a simple web server using Node.js. This is just a taste of what you can do with the right tools!
Understanding JavaScript Tools
JavaScript tools include libraries, frameworks, and environments that help you write and manage your code more effectively. Here are some common types:
-
Libraries: These are collections of pre-written code that you can use to perform common tasks. For example, jQuery simplifies HTML document manipulation.
-
Frameworks: A framework provides a structure for building applications. Popular frameworks include React and Angular.
-
Development Environments: Tools like Visual Studio Code or WebStorm offer features like syntax highlighting and debugging.
Key Parameters and Return Values
When evaluating JavaScript tools, consider the following:
- Ease of Use: How straightforward is the tool to learn?
- Community Support: Is there a large community for troubleshooting?
- Performance: Does it run efficiently on various devices?
- Compatibility: Does it work well with major browsers like Chrome, Firefox, and Safari?
Example 1: Using jQuery for DOM Manipulation
In this example, we use jQuery to hide all paragraphs when a button is clicked. jQuery is a powerful library for simplifying DOM manipulation.
Example 2: Building a Simple App with React
1import React from 'react';
2import ReactDOM from 'react-dom';
3
4function App() {
5 return <h1>Hello, world!</h1>;
6}
7
8ReactDOM.render(<App />, document.getElementById('root'));
Here, we create a simple React application that displays "Hello, world!" This framework is excellent for building interactive user interfaces.
Example 3: Setting Up a Development Environment with Visual Studio Code
Visual Studio Code (VS Code) is a popular choice for JavaScript developers. It supports extensions, allowing you to customize your environment. Here’s how to set it up:
- Download VS Code from the official site.
- Install extensions like ESLint for code linting and Prettier for code formatting.
Browser Compatibility
Most JavaScript tools are compatible with major web browsers. However, always check the documentation for any specific requirements or limitations. Tools like Babel can help ensure compatibility by transpiling modern JavaScript into older versions that work across different browsers.
Conclusion
Choosing the right JavaScript tools is crucial for maximizing efficiency in your development process. By understanding the types of tools available and their unique features, you can select the best options for your projects. Whether you’re working with libraries like jQuery, frameworks like React, or development environments like Visual Studio Code, the right tools can make a significant difference in your coding experience.
For more information on JavaScript and web development, consider exploring our courses on JavaScript, HTML Fundamentals, and CSS Introduction. Additionally, if you're new to web development, check out our Introduction to Web Development.
By carefully selecting and utilizing the right tools, you can streamline your workflow and enhance your coding skills!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
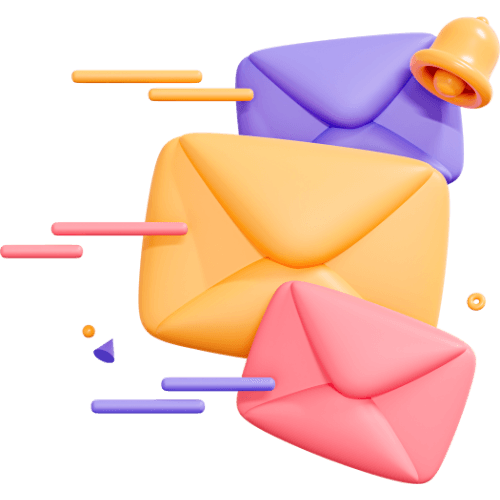
Related articles
9 Articles
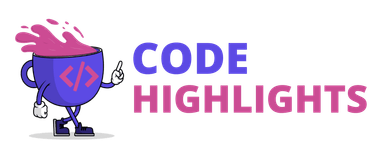
Copyright © Code Highlights 2024.