How to Javascript Uncheck Checkbox for Better UX

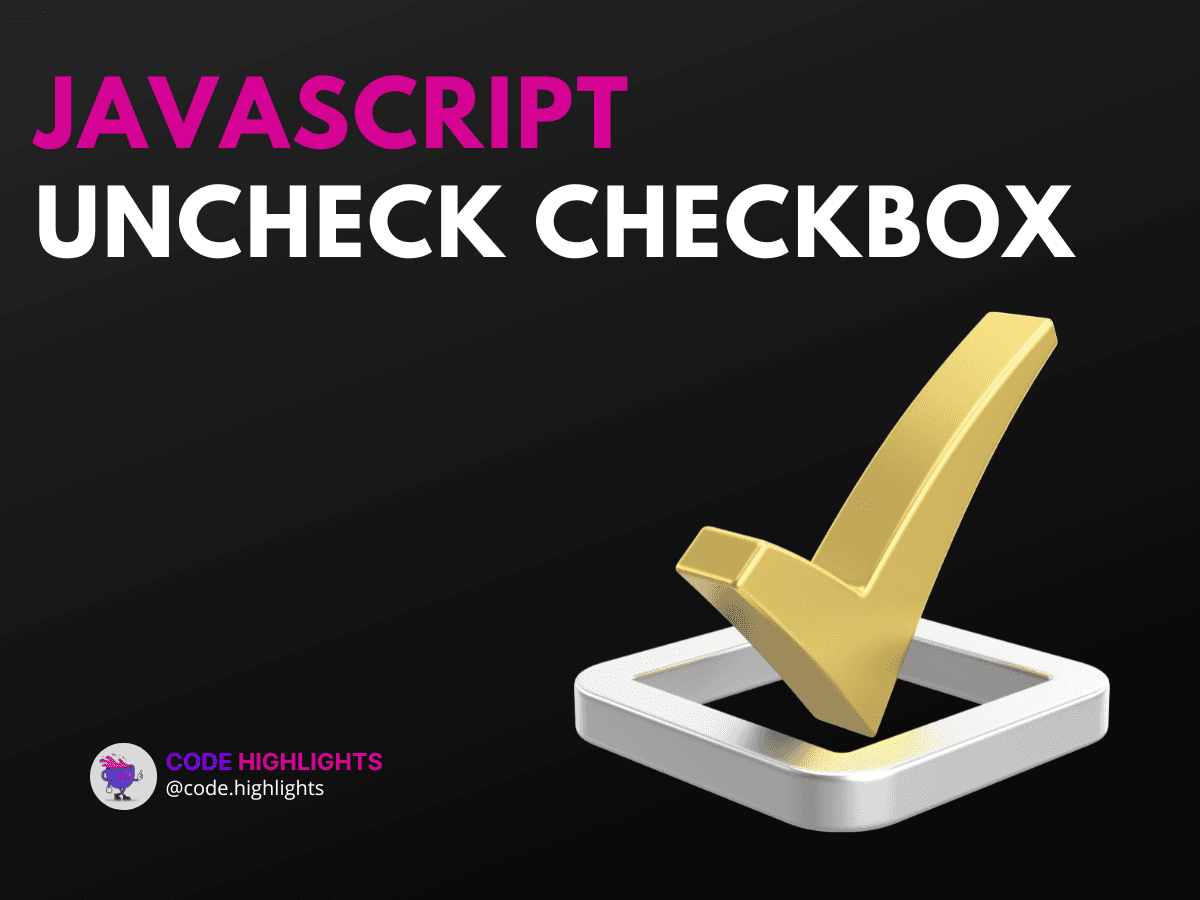
- Quick Example
- Understanding the Syntax
- Parameters
- Return Values
- Simple Uncheck Example
- Unchecking on Form Submission
- Unchecking with Event Listeners
- Uncheck on Checkbox Change
- Compatibility with Major Web Browsers
- Summary
Checkboxes are a common feature in web forms. They allow users to select one or more options. However, sometimes you may want to uncheck a checkbox automatically to improve user experience. In this tutorial, we will explore how to use JavaScript to uncheck checkboxes effectively.
Quick Example
Here’s a quick example of how to uncheck a checkbox using JavaScript:
1<input type="checkbox" id="myCheckbox" checked>
2<button onclick="uncheckCheckbox()">Uncheck</button>
3
4<script>
5function uncheckCheckbox() {
6 document.getElementById("myCheckbox").checked = false;
7}
8</script>
In this example, clicking the button will uncheck the checkbox.
Understanding the Syntax
To uncheck a checkbox in JavaScript, you can set its checked
property to false
. Here’s the basic syntax:
Parameters
checkboxElement
: This is the DOM element representing the checkbox.
Return Values
This operation does not return a value; it simply changes the state of the checkbox.
Simple Uncheck Example
Unchecking on Form Submission
Sometimes, you might want to uncheck checkboxes when a form is submitted. Here's how to do that:
1<form onsubmit="uncheckAll()">
2 <input type="checkbox" id="option1" checked>
3 <input type="checkbox" id="option2" checked>
4 <button type="submit">Submit</button>
5</form>
6
7<script>
8function uncheckAll() {
9 document.getElementById("option1").checked = false;
10 document.getElementById("option2").checked = false;
11}
12</script>
In this case, both checkboxes will be unchecked when the form is submitted.
Unchecking with Event Listeners
Uncheck on Checkbox Change
You can also uncheck a checkbox when another checkbox is checked. Here’s an example:
1<input type="checkbox" id="masterCheckbox" onchange="toggleCheckboxes()">
2<input type="checkbox" id="childCheckbox" checked>
3
4<script>
5function toggleCheckboxes() {
6 const master = document.getElementById("masterCheckbox");
7 document.getElementById("childCheckbox").checked = !master.checked;
8}
9</script>
In this example, checking the master checkbox will uncheck the child checkbox.
Compatibility with Major Web Browsers
The method to uncheck checkboxes using JavaScript is widely supported across all major browsers, including Chrome, Firefox, Safari, and Edge. Therefore, you can use this technique without worrying about compatibility issues.
Summary
In this tutorial, we covered how to use JavaScript to uncheck checkboxes effectively. We learned how to uncheck checkboxes on form submission, using event listeners, and by manipulating their properties directly. Understanding these techniques can significantly enhance user experience on your web pages. For further learning, check out our courses on JavaScript, HTML Fundamentals, and CSS Introduction.
For more information about checkboxes, you can refer to resources like MDN Web Docs and W3Schools. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
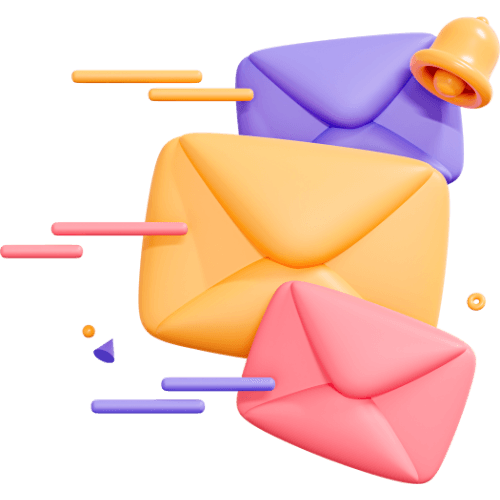
Related articles
9 Articles
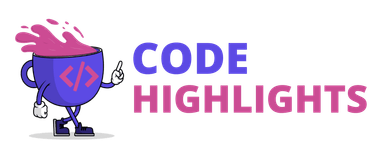
Copyright © Code Highlights 2025.