How to Wait 1 Second in JavaScript

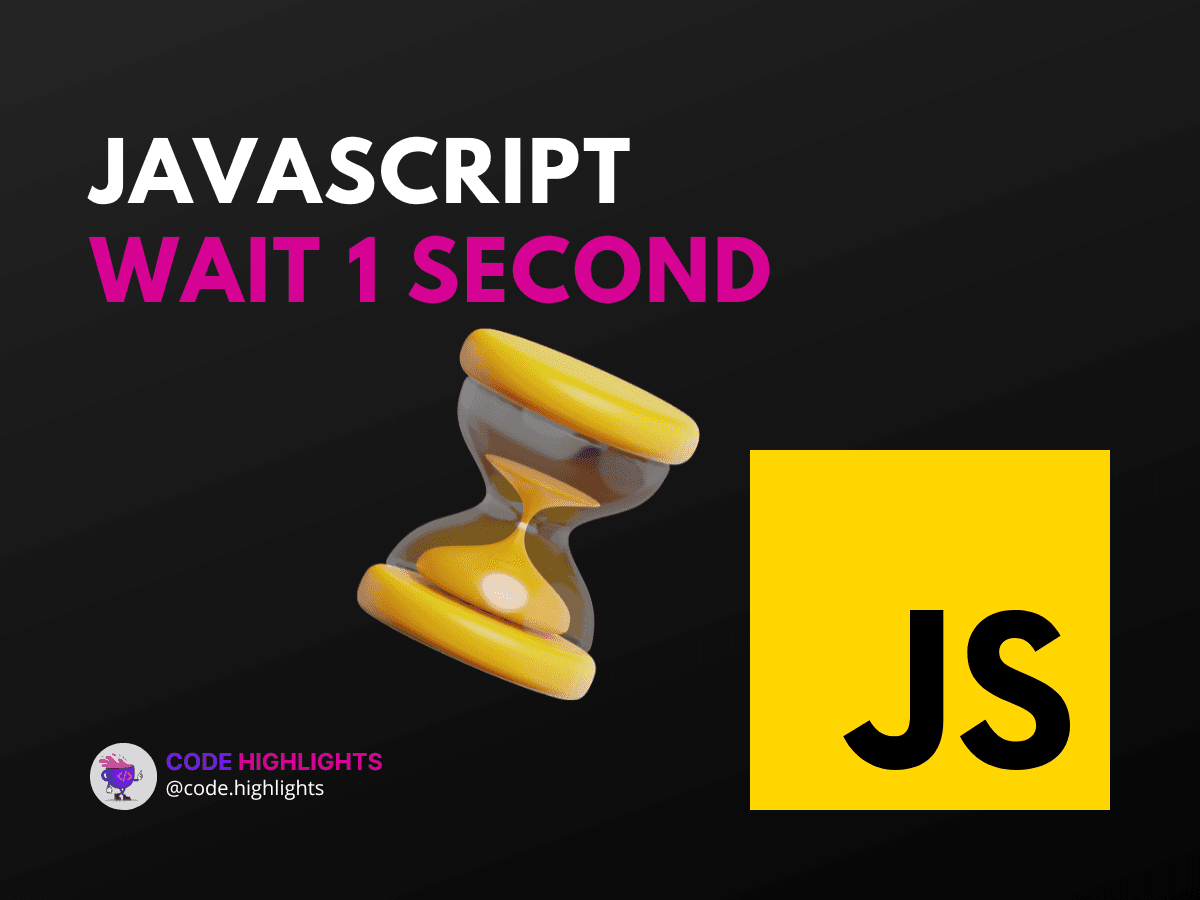
- Understanding setTimeout()
- Example: Using setTimeout() in a Web Page
- Advanced Use: Promises and async/await
- Conclusion
Waiting for a specific amount of time before executing a piece of code is a common task in programming. In JavaScript, this can be achieved with a few simple methods. Whether you're developing dynamic web applications or just starting with JavaScript, understanding how to implement a delay is essential. In this tutorial, we'll explore how to make JavaScript wait for 1 second before proceeding with the execution of subsequent code.
In the example above, we use the setTimeout()
function, which is built into the JavaScript language, to pause execution for 1000 milliseconds—which is equivalent to 1 second. This function is incredibly useful when dealing with asynchronous operations or adding a delay for a better user experience.
Understanding setTimeout()
The setTimeout()
function is a part of the Window interface in the Web APIs, but it's also available in Node.js environments. It accepts two parameters: a callback function and a delay in milliseconds.
callbackFunction
: The function to execute after the delay.delay
: The time to wait before executing the function, in milliseconds.
Example: Using setTimeout()
in a Web Page
Imagine you're working on a web project. You've already learned some HTML fundamentals and CSS, and now you're adding interactivity with JavaScript.
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>JavaScript Delay Example</title>
6</head>
7<body>
8 <button id="showMessageButton">Show Message After 1 Second</button>
9 <script>
10 document.getElementById('showMessageButton').addEventListener('click', function() {
11 setTimeout(function() {
12 alert('Hello! This message was delayed by 1 second.');
13 }, 1000);
14 });
15 </script>
16</body>
17</html>
Here, we've added an event listener to a button. When clicked, it triggers setTimeout()
to display an alert box after a 1-second delay.
Advanced Use: Promises and async/await
As you venture further into web development, you might encounter situations where using setTimeout()
within promises or async functions is beneficial. This allows you to use the modern async/await
syntax for cleaner, more readable code.
1function waitOneSecond() {
2 return new Promise(resolve => setTimeout(resolve, 1000));
3}
4
5async function runWithDelay() {
6 console.log('Starting delay');
7 await waitOneSecond();
8 console.log('1 second has passed');
9}
10
11runWithDelay();
In the waitOneSecond
function, we return a promise that resolves after a 1-second delay. Then, in runWithDelay
, we use await
to pause the function execution until the promise is resolved.
Conclusion
You now know how to make JavaScript wait for 1 second using setTimeout()
. This method is a fundamental part of JavaScript that can be applied in various scenarios, such as handling API calls or creating animations. Remember that while setTimeout()
is non-blocking, its use within promises and async/await
can help you manage asynchronous code more effectively.
For further reading and best practices in JavaScript timing events, check out resources from Mozilla Developer Network (MDN) and W3Schools. These reputable sources provide in-depth knowledge and are excellent for expanding your understanding of JavaScript and its features.
Happy coding, and may your timed executions always be punctual!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
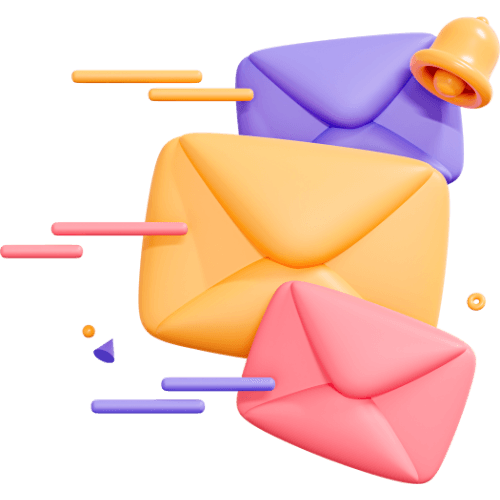
Related articles
9 Articles
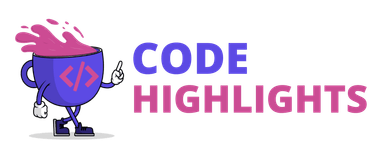
Copyright © Code Highlights 2025.