How to Calculate Last Day of Month JavaScript Easily

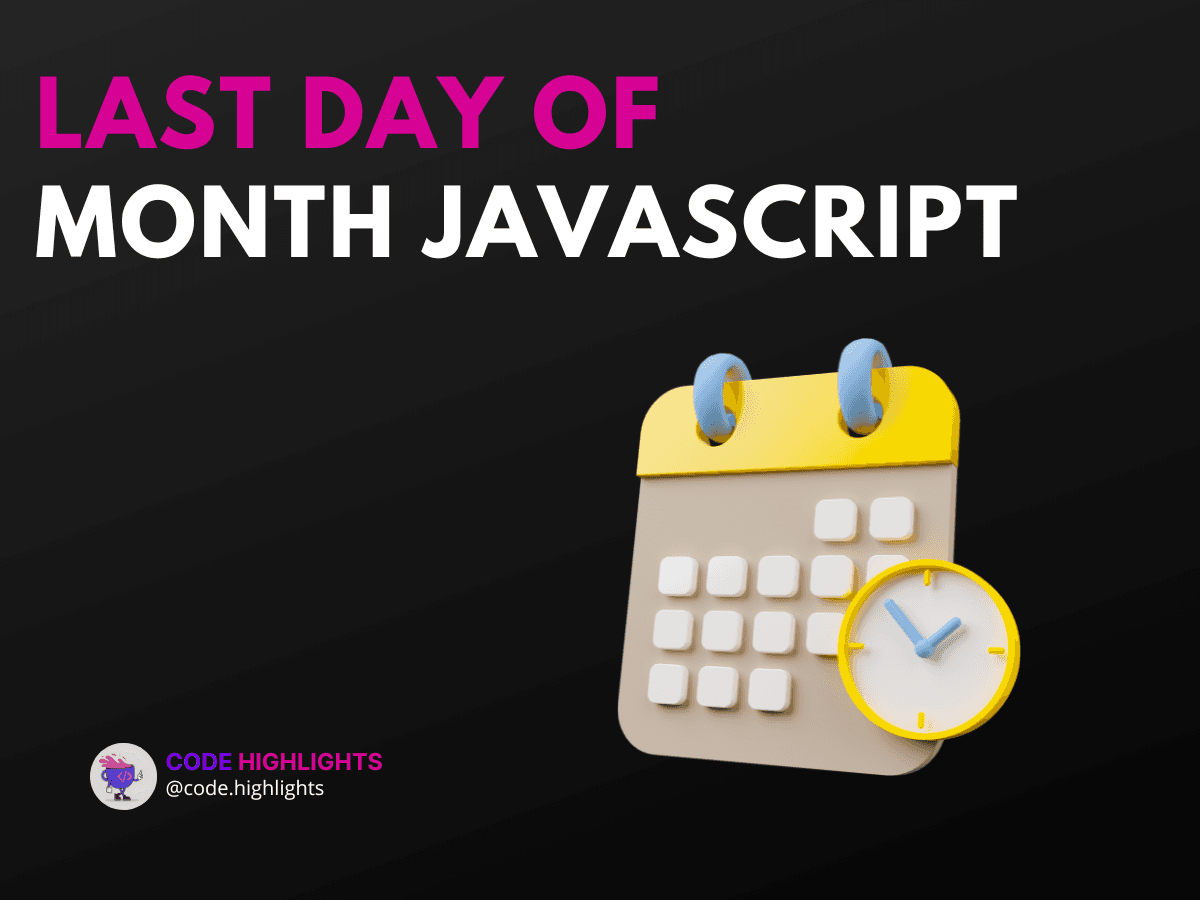
- Understanding the Syntax
- Key Points:
- Example 1: Get Last Day of Current Month
- Example 2: Last Day of Last Month
- Example 3: Get Day of the Month
- Example 4: Last Day of Current Year
- Browser Compatibility
- Summary
Calculating the last day of a month in JavaScript is a common task that can come in handy for various applications. Whether you're building a calendar app or managing date-related functionalities, knowing how to find the last day of a month is essential. In this tutorial, we will explore different ways to achieve this using JavaScript.
Here’s a quick code example to get you started:
1function getLastDayOfMonth(year, month) {
2 return new Date(year, month + 1, 0).getDate();
3}
4
5console.log(getLastDayOfMonth(2023, 0)); // Output: 31 (January)
Understanding the Syntax
The function getLastDayOfMonth
takes two parameters: year
and month
. The month
parameter is zero-based, meaning January is 0, February is 1, and so on. The function creates a new date by setting the day to 0 of the next month. This effectively gives us the last day of the current month.
Key Points:
- Parameters:
year
: The year as a number (e.g., 2023).month
: The month as a number (0-11).
- Return Value: The function returns the last day of the specified month as a number.
Example 1: Get Last Day of Current Month
1const today = new Date();
2const lastDay = getLastDayOfMonth(today.getFullYear(), today.getMonth());
3console.log(lastDay); // Output: Last day of current month
This example retrieves the current date, then uses our function to find the last day of the current month. It’s a straightforward way to keep track of dates dynamically.
Example 2: Last Day of Last Month
1function getLastDayOfLastMonth() {
2 const today = new Date();
3 return getLastDayOfMonth(today.getFullYear(), today.getMonth() - 1);
4}
5
6console.log(getLastDayOfLastMonth()); // Output: Last day of last month
In this case, we calculate the last day of the previous month. By subtracting one from the current month, we can easily find the last day of the last month.
Example 3: Get Day of the Month
1function getDayOfMonth(date) {
2 return date.getDate();
3}
4
5const someDate = new Date(2023, 0, 15);
6console.log(getDayOfMonth(someDate)); // Output: 15
This example shows how to retrieve the day of the month for a specific date. This can be useful when you need to compare dates or perform calculations based on the day.
Example 4: Last Day of Current Year
1function getLastDayOfCurrentYear() {
2 const year = new Date().getFullYear();
3 return getLastDayOfMonth(year, 11); // December is month 11
4}
5
6console.log(getLastDayOfCurrentYear()); // Output: 31
Here, we find the last day of the current year by passing December (month 11) to our function. This is useful for yearly reports or summaries.
Browser Compatibility
The methods used in this tutorial work well across major browsers like Chrome, Firefox, Safari, and Edge. However, it’s always good practice to test your code in different environments to ensure compatibility.
Summary
In this tutorial, we covered how to calculate the last day of a month in JavaScript. We explored various examples, including how to get the last day of the current month, the last day of the previous month, and even how to find the last day of the current year. Understanding these concepts can enhance your date handling skills in JavaScript.
For more in-depth learning, check out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. If you're just starting with web development, consider our Introduction to Web Development course.
By mastering these techniques, you can efficiently manage dates in your projects and applications!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
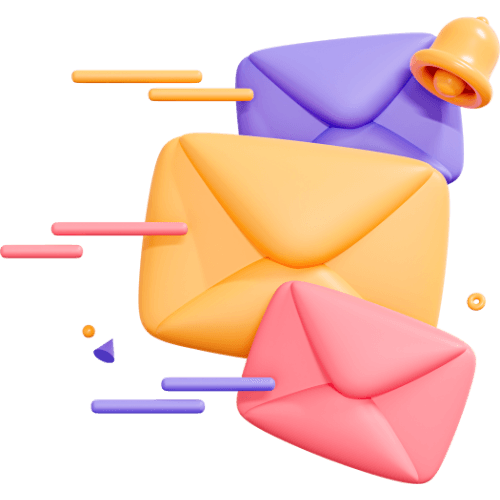
Related articles
9 Articles
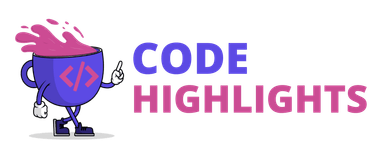
Copyright © Code Highlights 2025.