How to Link JavaScript to HTML: Elevate Your Web Development

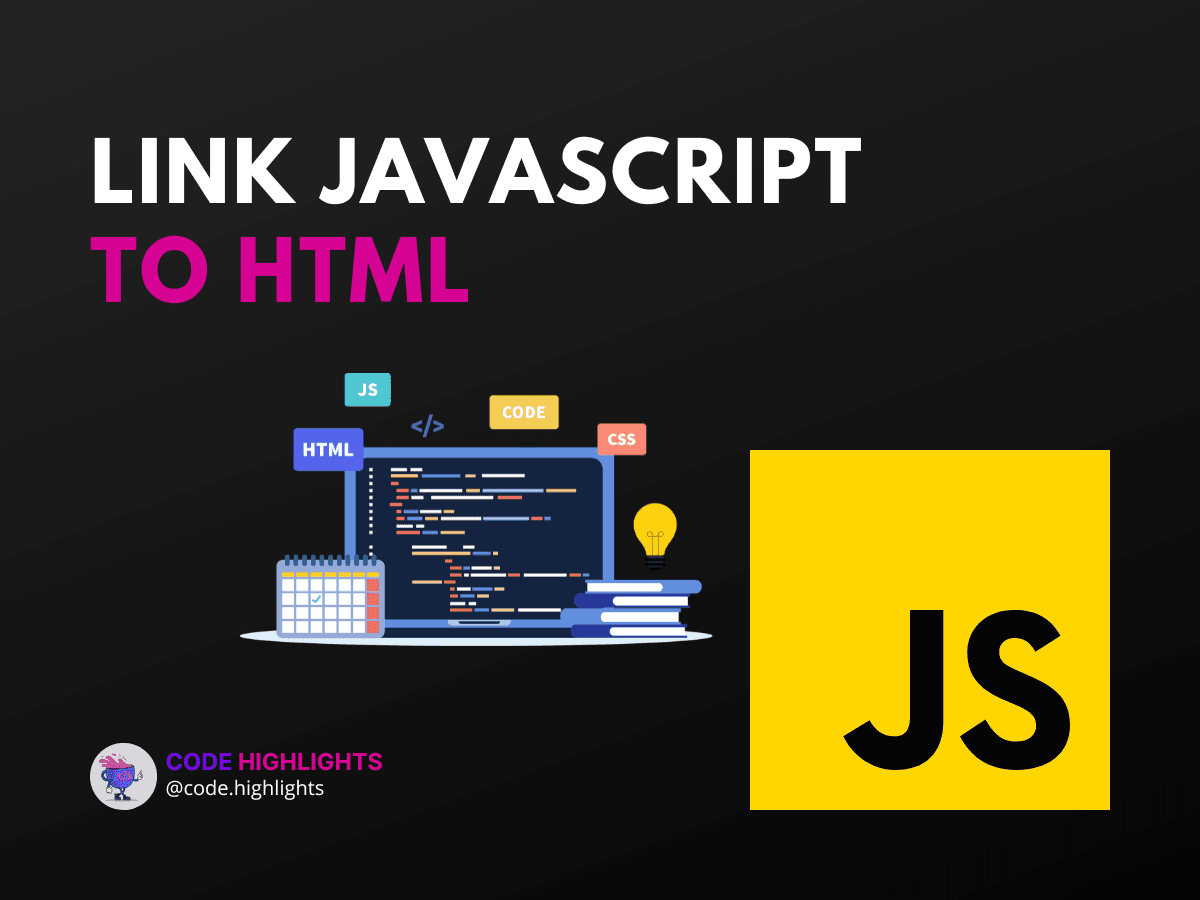
- Linking JavaScript to HTML
- The Script Tag
- Inline Event Handlers
- External JavaScript Files
- Document Object Model (DOM)
- Best Practices and Resources
- Conclusion
Welcome to this step-by-step tutorial where we'll explore how to seamlessly link JavaScript to HTML, a fundamental skill that can elevate your web development prowess. Have you ever wondered how the dynamic and interactive elements on a website come to life? It's through the power of JavaScript, brought into play within the structure of HTML. Let's dive in with a quick example:
1<!DOCTYPE html>
2<html>
3<head>
4 <title>Interactive Page</title>
5</head>
6<body>
7
8<h1>Welcome to My Web Page</h1>
9<button onclick="showMessage()">Click Me!</button>
10
11<script>
12 function showMessage() {
13 alert('Hello, welcome to the site!');
14 }
15</script>
16
17</body>
18</html>
In the code above, we see a simple button that, when clicked, triggers a JavaScript function directly linked within our HTML document. This is just the tip of the iceberg. By the end of this tutorial, you'll understand the ins and outs of integrating JavaScript into your HTML documents, creating interactive and dynamic user experiences.
Linking JavaScript to HTML
When it comes to making your web pages interactive, linking JavaScript to HTML is essential. There are several methods to achieve this, each with its own use cases and benefits.
The Script Tag
The most common way to link JavaScript to HTML is by using the <script>
tag. This tag can be placed within the <head>
or <body>
sections of your HTML. If you're looking to learn the basics of JavaScript, consider enrolling in a JavaScript course to build a solid foundation.
Here's how you can include a script in your HTML document:
1<!DOCTYPE html>
2<html>
3<head>
4 <title>My Interactive Page</title>
5 <!-- JavaScript linked in the head -->
6 <script src="path/to/your-script.js"></script>
7</head>
8<body>
9 <!-- Your HTML content goes here -->
10</body>
11</html>
Inline Event Handlers
You might be wondering, "How do you link between HTML and JavaScript?" One way is through inline event handlers. These are attributes within HTML tags that respond to user events, such as clicks or keystrokes. For instance, to link a JavaScript function to an HTML button, you could use an onclick
attribute like so:
1<button onclick="myFunction()">Press me</button>
However, this approach can quickly become unmanageable for larger projects, and it's generally recommended to separate your JavaScript from your HTML for better maintainability.
External JavaScript Files
For a cleaner and more organized approach, linking an external JavaScript file is the way to go. This not only helps to link JavaScript function in HTML but also promotes reusability across different pages. Here's how you do it:
- Create a
.js
file – typically named something likescripts.js
. - Write your JavaScript code in this file.
- Link this file to your HTML with the
<script>
tag'ssrc
attribute:
1<script src="scripts.js"></script>
Place this tag right before your closing </body>
tag to ensure that your HTML is fully loaded before the JavaScript runs.
Document Object Model (DOM)
Understanding the Document Object Model (DOM) is crucial for dynamic web development. It's the bridge between HTML and JavaScript, allowing scripts to access and manipulate the webpage. If you're new to web development, a comprehensive introduction to web development course can give you a head start.
Best Practices and Resources
While learning how to link JavaScript to HTML, it's beneficial to follow best practices and consult reputable sources. Here are a few tips and resources:
- Always place your
<script>
tags at the end of the body for better page load performance. - Use Mozilla Developer Network (MDN) as a reliable source for learning and reference.
- Keep your code clean and well-commented for future reference and collaboration.
Conclusion
Linking JavaScript to HTML is a critical skill set for any aspiring web developer. By understanding the various methods and best practices outlined in this tutorial, you're well on your way to creating engaging, interactive websites. Remember to keep experimenting and learning; web development is an ever-evolving field with no shortage of new techniques to master.
To further enhance your skills, don't hesitate to explore courses on HTML fundamentals and CSS introduction, as these are the building blocks of web development alongside JavaScript. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
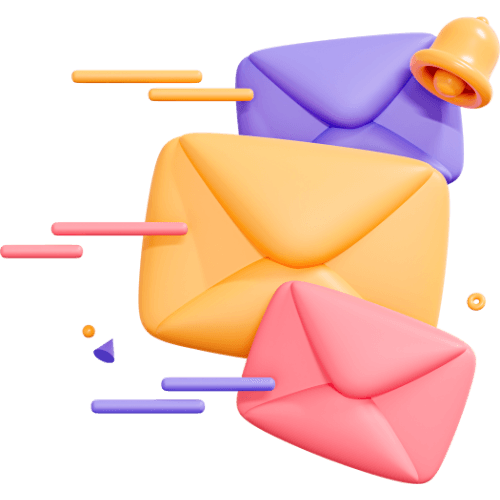
Related articles
114 Articles
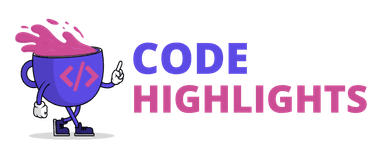
Copyright © Code Highlights 2024.