How to Loop Object in JavaScript: Best Practices!

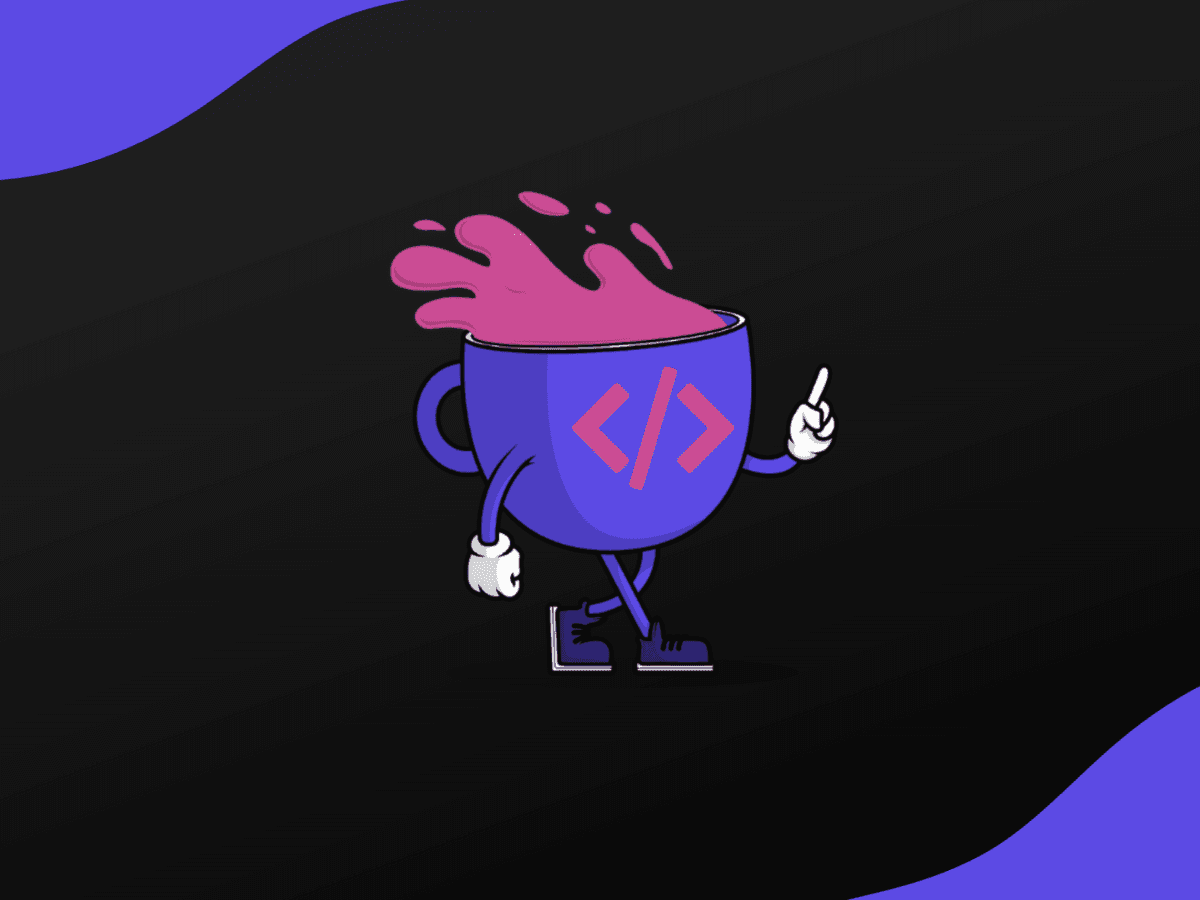
- for...in Loop
- Object.keys() and Array.prototype.forEach()
- Object.entries()
- Conclusion
In JavaScript, objects are a fundamental building block of the language. They allow us to group related data and functions together, which can make our code more readable and maintainable. However, one common task that can be somewhat tricky is looping over an object's properties. In this tutorial, we'll explore the best ways to do this.
for...in
Loop
The for...in
loop is a method built into JavaScript that allows us to iterate over all enumerable properties of an object.
1var obj = {a: 1, b: 2, c: 3};
2
3for (var prop in obj) {
4 console.log(`obj.${prop} = ${obj[prop]}`);
5}
This will output:
obj.a = 1
obj.b = 2
obj.c = 3
Object.keys()
and Array.prototype.forEach()
Another approach is to use the Object.keys()
method in combination with Array.prototype.forEach()
. This allows us to loop over the keys of an object, and then access each value inside the loop.
1var obj = {a: 1, b: 2, c: 3};
2
3Object.keys(obj).forEach(function(key) {
4 console.log(`obj.${key} = ${obj[key]}`);
5});
This will output the same result as the for...in
loop example above.
Object.entries()
The Object.entries()
method returns an array of an object's own enumerable string-keyed property [key, value] pairs. This can be useful for looping over objects in a more functional manner.
1var obj = {a: 1, b: 2, c: 3};
2
3Object.entries(obj).forEach(([key, value]) => {
4 console.log(`obj.${key} = ${value}`);
5});
This will also output the same result as the previous examples.
Conclusion
In conclusion, there are several ways to loop over an object in JavaScript. The best method for you will depend on your specific needs and coding style. For a more in-depth look at JavaScript objects, check out our Learn JavaScript course. If you're new to web development, you might find our Introduction to Web Development helpful.
For further reading, check out MDN's guide on loops and iteration and W3Schools' tutorial on JavaScript Objects.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
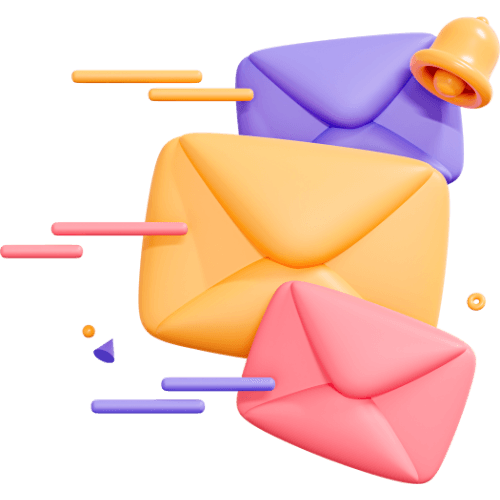
Related articles
9 Articles
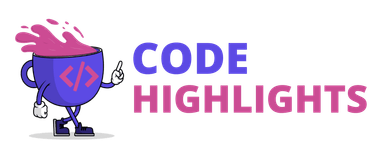
Copyright © Code Highlights 2025.