How to Fix 'Map is Not a Function JavaScript' for Smooth Coding

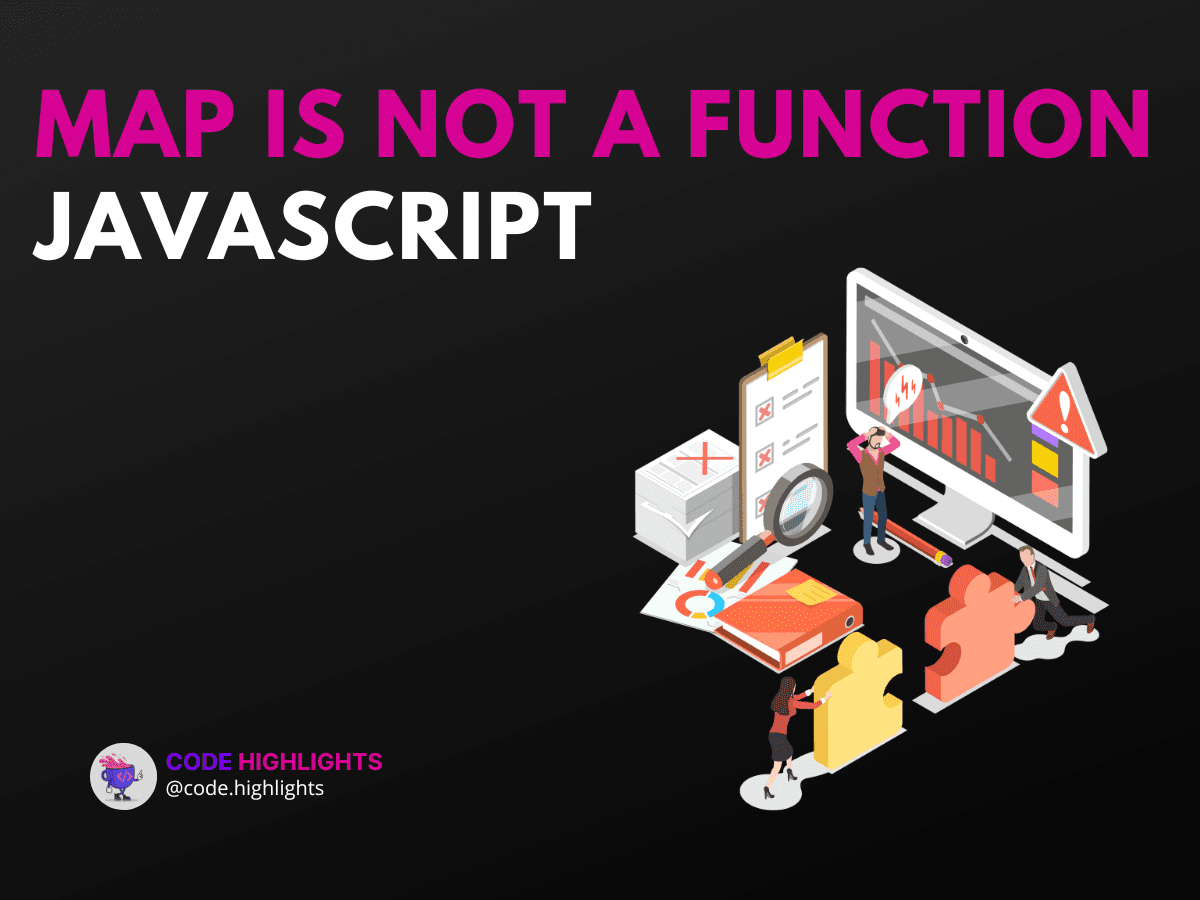
- Quick Code Example
- Understanding the map Function
- Common Causes of the Error
- Fixing the Error
- Alternative Methods
- Compatibility with Browsers
- Summary
If you've ever encountered the error message map is not a function javascript
, you're not alone. This common issue can disrupt your coding flow, especially when working with arrays. In this tutorial, we'll explore what causes this error and how to fix it effectively. By the end, you'll have a clearer understanding of the map
method in JavaScript and how to avoid these pitfalls.
Quick Code Example
Before diving into the details, let's look at a simple example of using the map
function:
1const numbers = [1, 2, 3, 4];
2const doubled = numbers.map(num => num * 2);
3console.log(doubled); // Output: [2, 4, 6, 8]
Understanding the map
Function
The map
function is an essential method in JavaScript that allows you to create a new array by applying a function to each element of an existing array. Here’s the basic syntax:
-
Parameters:
callback
: A function that is called for every element in the array.currentValue
: The current element being processed.index
(optional): The index of the current element.array
(optional): The arraymap
was called upon.thisArg
(optional): Value to use asthis
when executingcallback
.
-
Return Value: A new array with each element being the result of the callback function.
Common Causes of the Error
-
Not an Array: The most common reason for the error is calling
map
on something that isn’t an array. For instance, if you mistakenly call it on an object or a string, you’ll see this error. -
Undefined or Null: If the variable you’re trying to use
map
on isundefined
ornull
, the same error will occur.
Fixing the Error
Example 1: Ensure You're Working with an Array
Make sure the variable you're calling map
on is indeed an array. You can check this using Array.isArray()
:
1const data = null;
2if (Array.isArray(data)) {
3 const results = data.map(item => item * 2);
4} else {
5 console.log("Data is not an array");
6}
Example 2: Default to an Empty Array
Another approach is to default to an empty array if the variable is not defined:
1const items = undefined;
2const results = (items || []).map(item => item * 2);
3console.log(results); // Output: []
Alternative Methods
If you find yourself in a situation where map
isn't suitable, consider using other methods like forEach
or reduce
.
For example, if you just want to iterate over an array without creating a new one, forEach
can be handy:
Compatibility with Browsers
The map
function is widely supported across all major browsers, including Chrome, Firefox, Safari, and Edge. However, if you're targeting older browsers, make sure to check compatibility on resources like MDN Web Docs.
Summary
In this tutorial, we explored the map
function in JavaScript and the common error message map is not a function javascript
. We learned how to identify the causes of this error and implement solutions to fix it. Remember to always ensure that you're working with an array before using map
.
For more in-depth learning, check out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
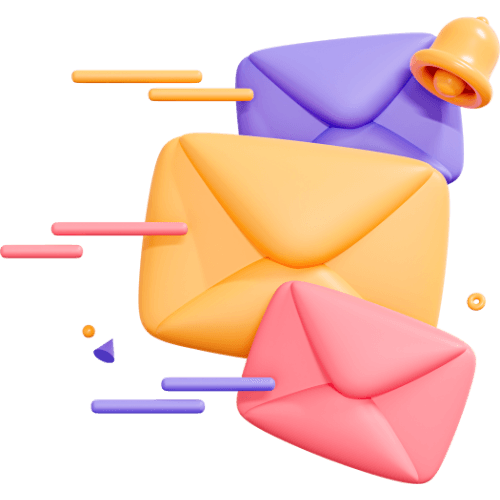
Related articles
9 Articles
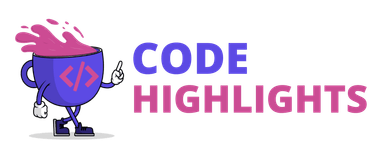
Copyright © Code Highlights 2025.