Mastering JavaScript Promises

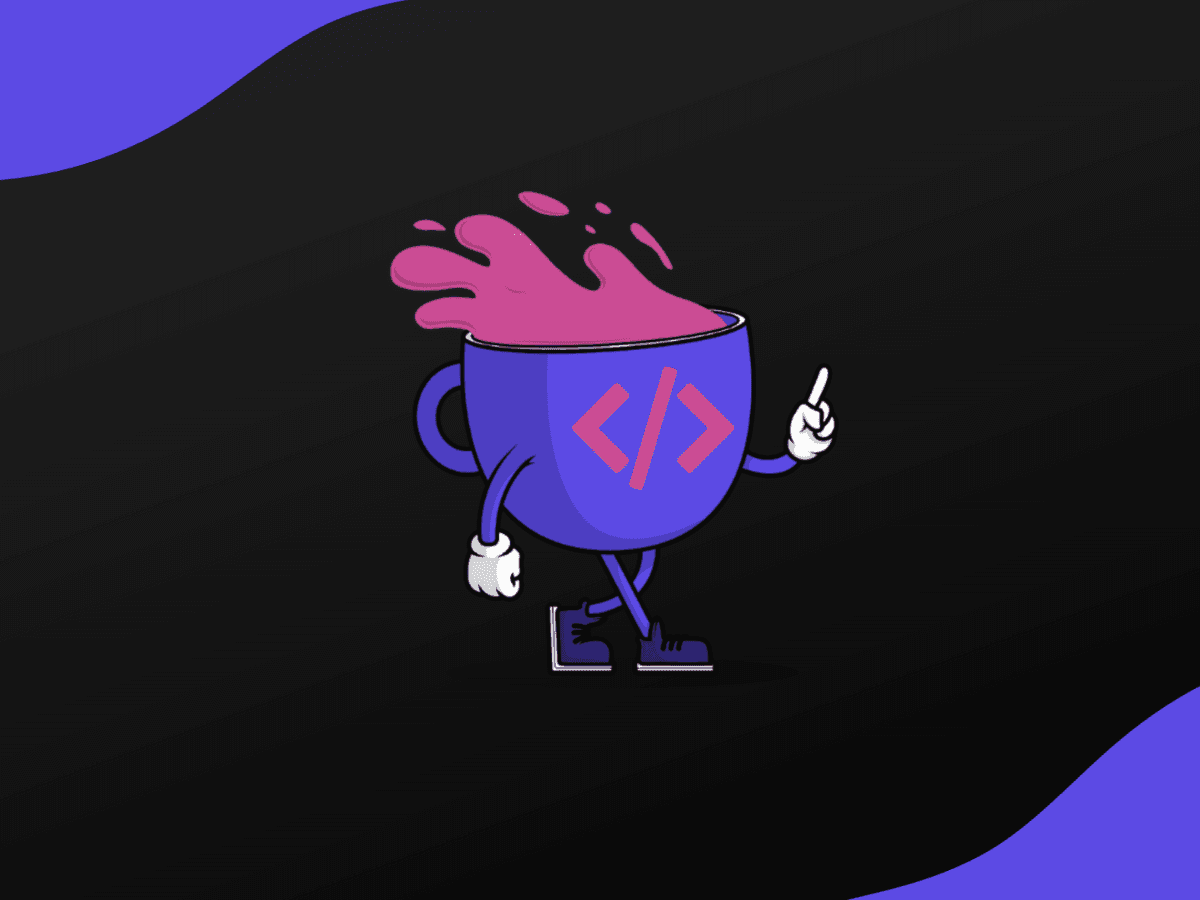
- Understanding JavaScript Promises
- Utilizing Promise.all()
- Practical Examples and External References
- Conclusion
Promises in JavaScript are a fundamental concept that every web developer should understand. They are the backbone of writing asynchronous code in a manageable way. If you've ever found yourself lost in callback hell, tangled up in nested functions, then understanding promises can be your path to redemption. Let's embark on a journey to master JavaScript promises and make our code cleaner, more readable, and efficient.
1let myFirstPromise = new Promise((resolve, reject) => {
2 // We'll simulate a task that takes time, like an API call
3 setTimeout(function () {
4 resolve("Success!"); // Yay! Everything went well!
5 }, 250);
6});
7
8myFirstPromise.then((successMessage) => {
9 console.log("Yay! " + successMessage);
10});
The above snippet is a simple example of creating and using a promise. But what exactly is a promise? A Promise in JavaScript represents an operation that hasn't completed yet but is expected in the future. It's a placeholder for a value that will be available later, allowing you to write code that assumes a value will be there eventually without stopping everything to wait for it.
Understanding JavaScript Promises
To get a better grasp on JavaScript promises, let's answer the question: What is a Promise in Javascript?
A promise is an object that represents the eventual completion or failure of an asynchronous operation. It allows you to write code that assumes a value will be there eventually without stopping everything to wait for it. Promises are a way to handle asynchronous operations in JavaScript. They are used to avoid callback hell and make code more readable.
A promise has three states:
- Pending: The initial state—neither fulfilled nor rejected.
- Fulfilled: The operation completed successfully.
- Rejected: The operation failed.
When a promise is fulfilled or rejected, it is settled, and its state won't change after that. This behavior ensures consistency throughout the execution of your code.
Utilizing Promise.all()
Sometimes, you might need to execute multiple promises and wait for all of them to be resolved. This is where Promise.all()
comes in. But what does Promise All
do? Promise.all()
is a method that takes an array of promises and returns a new promise that resolves when all the promises in the array have been resolved or rejects if any of the promises are rejected.
Here's an example of using Promise.all()
:
1let promise1 = Promise.resolve(3);
2let promise2 = 42;
3let promise3 = new Promise((resolve, reject) => {
4 setTimeout(resolve, 100, "foo");
5});
6
7Promise.all([promise1, promise2, promise3]).then((values) => {
8 console.log(values); // Output: [3, 42, "foo"]
9});
In this example, Promise.all()
is used to aggregate the results of multiple promises. This is particularly useful when you have several asynchronous operations that are independent of each other, and you want to wait for them all to complete before proceeding.
Practical Examples and External References
To further your understanding, check out these external resources:
- MDN Web Docs on Promises - A comprehensive guide to promises.
- JavaScript.info on Promises - An interactive tutorial on the basics of promises.
Conclusion
Promises in JavaScript are a powerful tool for managing asynchronous operations. By mastering promises, you'll write code that's not only easier to understand and maintain but also more robust and reliable. As you continue to explore web development, be sure to dive into HTML fundamentals, CSS, and other web development courses to build a strong foundation for your coding skills.
Remember, a promise is a commitment to a future value. Keep practicing, and soon you'll be creating complex applications with ease, all thanks to the power of JavaScript promises. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
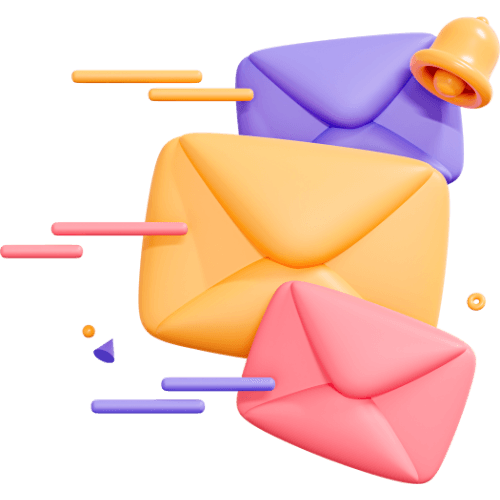
Related articles
9 Articles
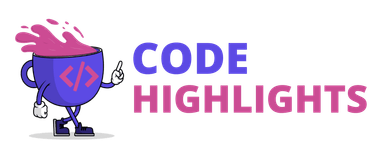
Copyright © Code Highlights 2025.