Math.floor JavaScript: Avoid Rounding Mistakes

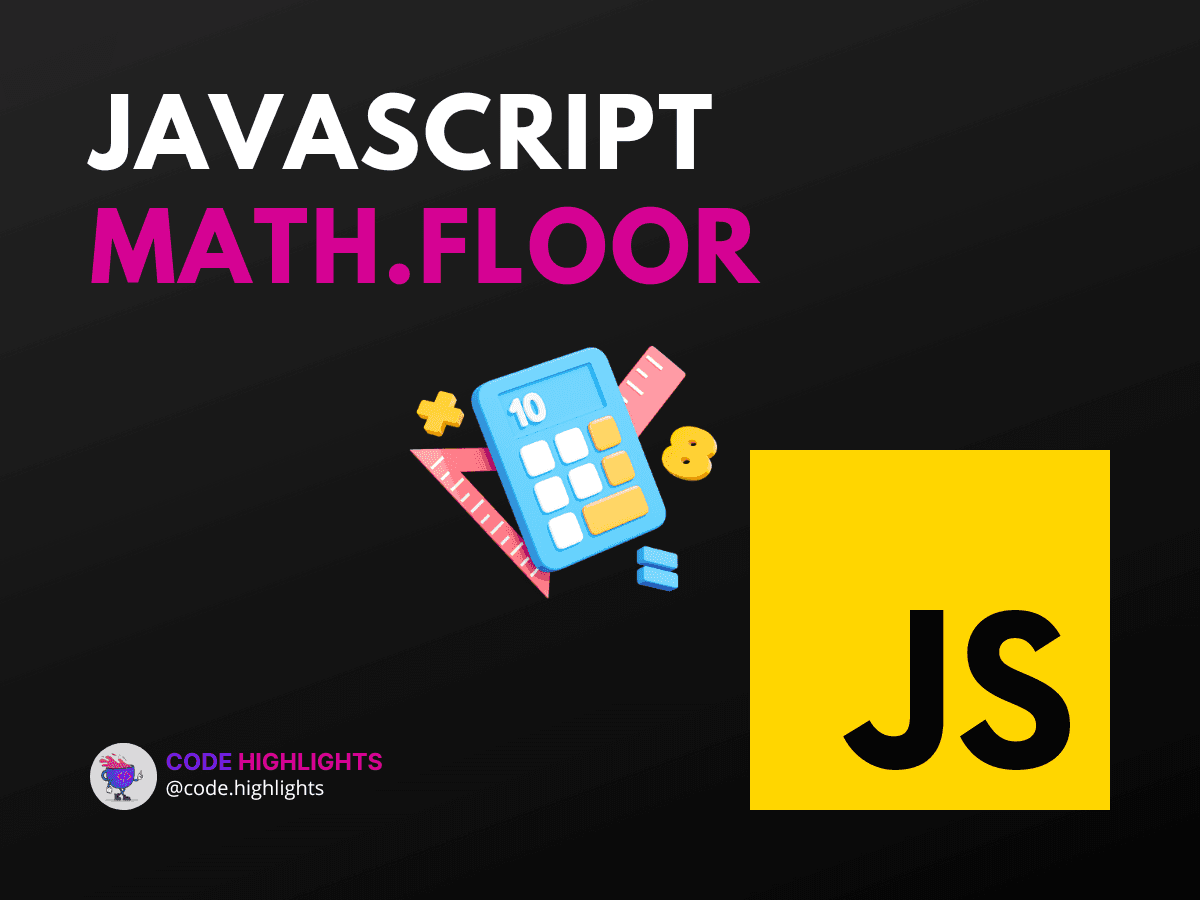
- What is Math.floor in JavaScript?
- What does the floor() method do?
- The Difference Between Math.ceil and floor in JS
- How to Floor a Variable in JavaScript
Have you ever faced a situation in your coding journey where precise number rounding was crucial? Maybe you're calculating currency or setting up a game score system, and you need to round down to the nearest whole number. That's where Math.floor
in JavaScript comes into play, ensuring your numbers aren't just rounded—they're rounded correctly.
This simple line of code is the beginning of understanding how Math.floor
can be a lifesaver. Let's dive deeper into this function and see how it can help you avoid rounding mistakes in your JavaScript projects.
When you're working with numbers in JavaScript, rounding them can often lead to unexpected results if not done properly. This is where the Math.floor
function becomes invaluable.
What is Math.floor in JavaScript?
Math.floor
is a built-in method in JavaScript that rounds a number down to its nearest integer. No matter what the decimal part is, Math.floor
will always go to the lower integer.
What does the floor() method do?
The floor()
method takes a floating-point number and chops off the decimal points, leaving you with the largest integer less than or equal to the original number. It's like knocking down the number to its floor value without giving it a chance to climb up to the ceiling.
The Difference Between Math.ceil and floor in JS
While Math.floor
always rounds down, its counterpart Math.ceil
(short for "ceiling") does the opposite—it always rounds up to the nearest integer. Imagine you have a number like 2.3; Math.floor
would give you 2, while Math.ceil
would bump it up to 3.
How to Floor a Variable in JavaScript
Flooring a variable is straightforward:
1let temperature = 36.6;
2let normalTemp = Math.floor(temperature);
3console.log(normalTemp); // Outputs: 36
In this example, we've taken a body temperature reading and floored it, which could be useful in a medical application where only whole numbers are considered.
To expand your knowledge on JavaScript and web development, consider enrolling in these courses:
Also, mastering HTML and CSS will further enhance your web projects:
For more context and examples, reputable sources such as Mozilla Developer Network (MDN) and W3Schools offer extensive documentation on JavaScript methods.
By now, you should have a clear understanding of how Math.floor
works in JavaScript and why it's essential for precise number handling. Remember, when in doubt, floor it out—your code will thank you for the precision!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
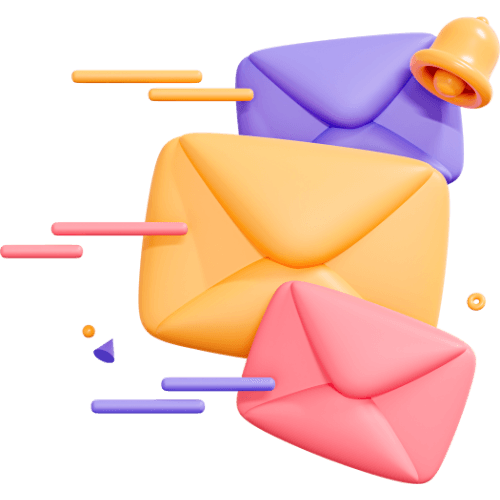
Related articles
114 Articles
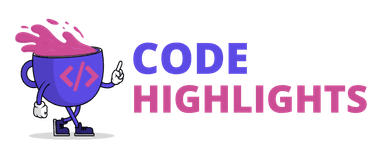
Copyright © Code Highlights 2024.