5 Modulo JavaScript Tricks to Simplify Your Code

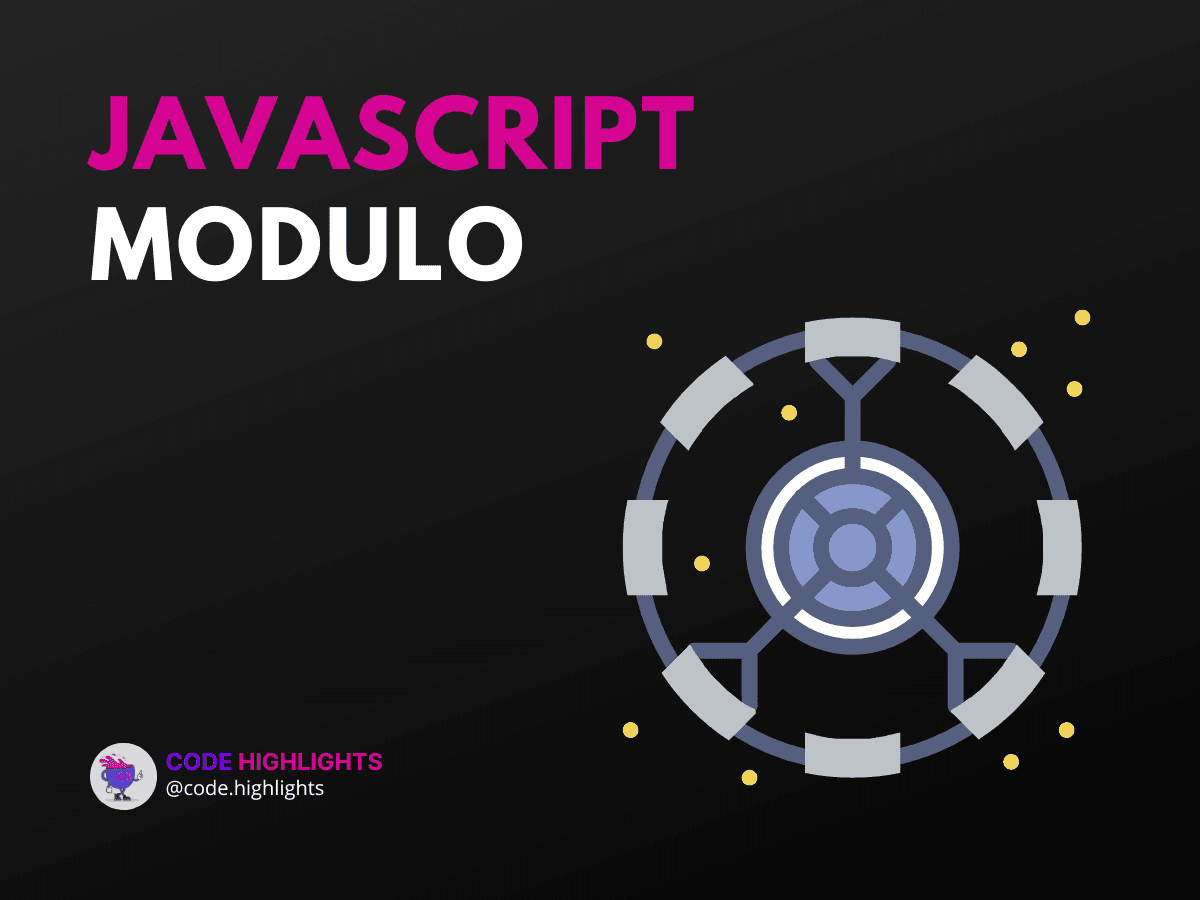
- Understanding Modulo in JavaScript
- Trick 1: Determining Even or Odd Numbers
- Trick 2: Creating a Loop That Wraps Around
- Trick 3: Formatting Time Correctly
- Trick 4: Implementing a Simple Hash Function
- Trick 5: Cycling Through Array Indices
- Addressing Common Questions
- Conclusion
Have you ever found yourself lost in the labyrinth of complex code? Let's simplify that journey with a mathematical operator that might just be the Swiss Army knife in your coding toolkit: the modulo operator. In this tutorial, we'll uncover five ingenious tricks using modulo javascript
to streamline your code and make it more efficient.
Before we dive deep, here's a quick taste of what modulo can do in JavaScript:
This snippet demonstrates how the modulo operator returns the remainder of a division. But there's so much more to it!
Understanding Modulo in JavaScript
Modulo, often represented by the percentage sign %
, is an arithmetic operator that gives you the remainder of a division between two numbers. For example, 7 % 2
would return 1
because when you divide 7 by 2, you have a remainder of 1.
Trick 1: Determining Even or Odd Numbers
One common use of the modulo operator is to check whether a number is even or odd:
If you're curious about the fundamentals behind this, our JavaScript course provides a comprehensive look into operators in JavaScript.
Trick 2: Creating a Loop That Wraps Around
When dealing with arrays or lists, you might want to create a loop that goes back to the start after reaching the end. This is where modulo shines:
1let array = ['a', 'b', 'c', 'd'];
2for (let i = 0; i < 10; i++) {
3 console.log(array[i % array.length]);
4}
Trick 3: Formatting Time Correctly
Imagine you're building a clock and need to convert minutes to hours and minutes. The modulo operator can help with that:
1function formatTime(totalMinutes) {
2 let hours = Math.floor(totalMinutes / 60);
3 let minutes = totalMinutes % 60;
4 return `${hours}h ${minutes}m`;
5}
Trick 4: Implementing a Simple Hash Function
A hash function can distribute inputs into many "buckets" for things like a hash table. Modulo can be used to ensure the result stays within a certain range:
1function simpleHash(input, bucketSize) {
2 // Here, a simple sum of char codes is used as an example
3 let sum = input.split('').reduce((acc, char) => acc + char.charCodeAt(0), 0);
4 return sum % bucketSize;
5}
Trick 5: Cycling Through Array Indices
When creating a slideshow or carousel, you might want to cycle through images. Modulo helps you to loop back to the first image after the last one:
1let images = ['image1.jpg', 'image2.jpg', 'image3.jpg'];
2let currentIndex = 0;
3
4function getNextImage() {
5 currentIndex = (currentIndex + 1) % images.length;
6 return images[currentIndex];
7}
Addressing Common Questions
- Was macht Modulo? In C++ or Python, modulo (
%
) works similarly to JavaScript, returning the remainder of a division. This behavior is consistent across many programming languages. - Was sind Operatoren Javascript? Operators in JavaScript are symbols that tell the interpreter to perform specific mathematical, relational, or logical operations and produce a result.
- Was bedeutet Modulo in C++? In C++, modulo (
%
) also calculates the remainder of a division between two integers. It's a fundamental operator in arithmetic operations. - Was ist Modulo in Python? In Python, the modulo operator (
%
) functions the same way as in JavaScript and C++, returning the remainder after division.
Conclusion
The modulo operator is a powerful tool that can help you write cleaner and more efficient JavaScript code. By mastering these tricks, you'll be well on your way to becoming a more proficient developer.
If you're looking to expand your web development skills further, consider exploring our HTML fundamentals course, CSS introduction, or our broader introduction to web development.
Remember to practice these modulo JavaScript tricks in your next project to see how they can simplify your code. Happy coding!
For further reading on the modulo operator and its applications in various programming languages, consider these resources from reputable sources:
- MDN Web Docs on Arithmetic Operators
- Harvard CS50 Introduction to Computer Science
- Python's Official Documentation on Operators
By incorporating these tricks and resources into your coding practice, you'll be leveraging the full potential of the modulo operator to write more elegant and maintainable code.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
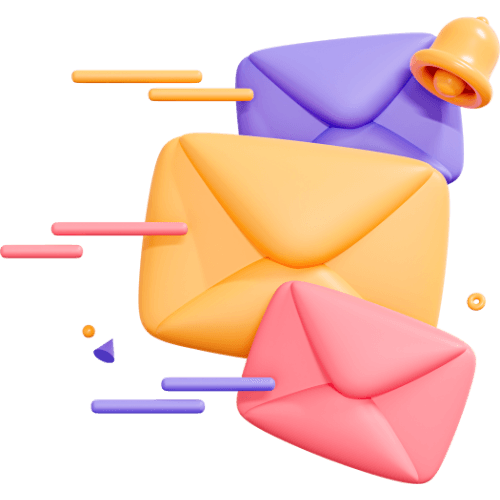
Related articles
9 Articles
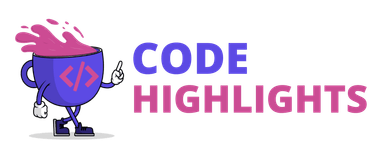
Copyright © Code Highlights 2025.