Mastering Modulus in JavaScript: A Step-by-Step Guide

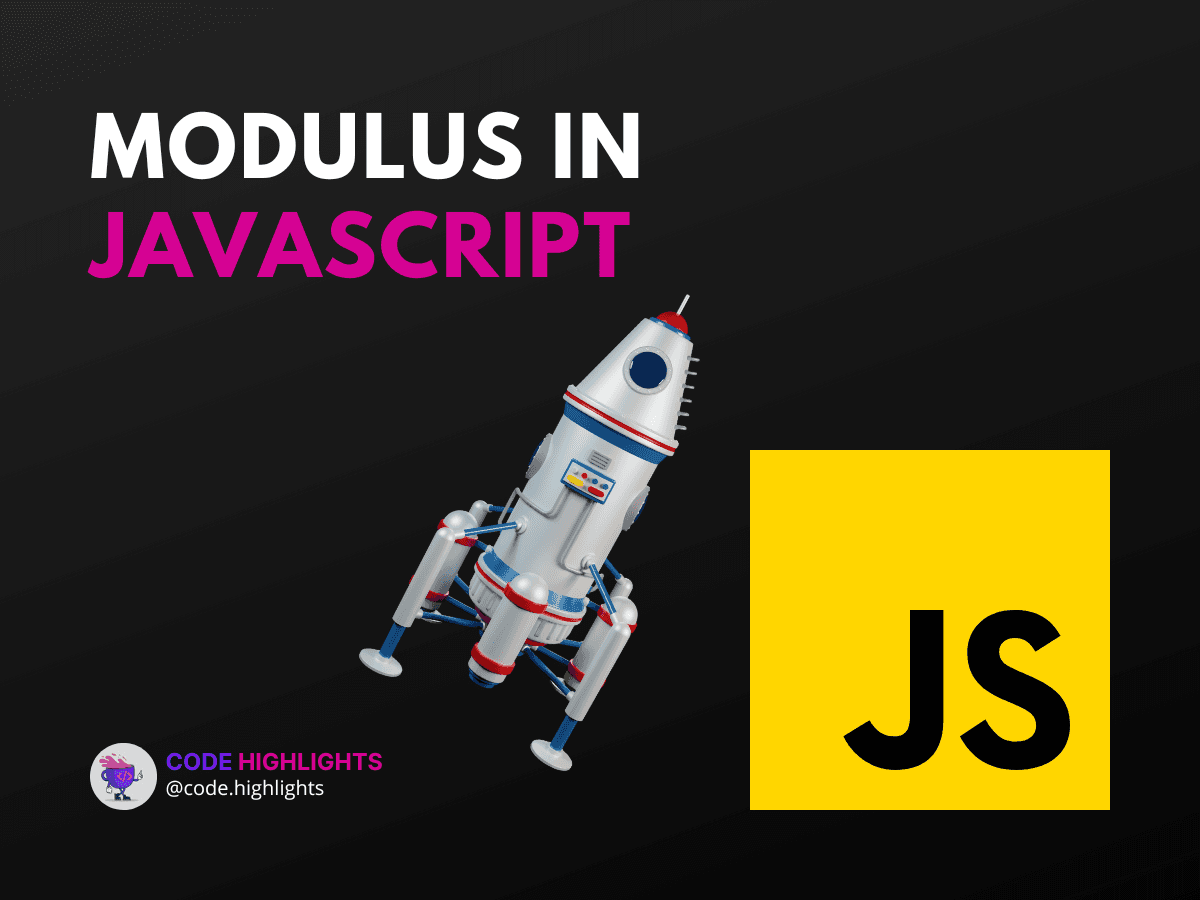
- What Does Modulus (%) Do?
- What is '%' in JavaScript?
- What is Modular Function in JavaScript?
- How to Use Modulo
- Practical Applications
- Zebra Stripes Example
- Conclusion
Are you ready to unlock the secrets of the modulus operator in JavaScript? This mathematical marvel might seem intimidating at first, but with this guide, you'll be wielding it like a pro in no time! Modulus isn't just about finding remainders; it's a key player in various coding scenarios. Let's dive in with a quick taste of how modulus works:
In this example, %
is our modulus operator, effortlessly calculating the remainder of dividing 10 by 3. Intrigued? Keep reading as we explore the depths of modulus in javascript
, ensuring you come out as a modulo maven!
What Does Modulus (%) Do?
The modulus operator, represented by %
, is a tool that determines the remainder after division of one number by another. In essence, it answers the question: "What's left over?" This operation is crucial when you need to perform tasks that require division with a need to know the remaining value. For instance, it's indispensable for algorithms that deal with cyclical events like rotating images in a gallery or determining even and odd numbers.
What is '%' in JavaScript?
In JavaScript, the %
symbol is known as the modulus or modulo operator. It's a binary operator that takes two operands: the dividend and the divisor. Here's a simple formula:
For example, if you want to check whether a number is even or odd, you could use the modulus operator:
1let number = 4;
2console.log(number % 2); // Outputs: 0 (even)
3number = 5;
4console.log(number % 2); // Outputs: 1 (odd)
What is Modular Function in JavaScript?
A modular function in JavaScript isn't a built-in feature but rather a concept where you create functions that utilize the modulus operator to achieve a specific task. For example, a function that checks for divisibility:
1function isDivisible(dividend, divisor) {
2 return dividend % divisor === 0;
3}
4
5console.log(isDivisible(10, 5)); // Outputs: true
6console.log(isDivisible(10, 3)); // Outputs: false
How to Use Modulo
Using modulo is straightforward. Consider a scenario where you need to loop through an array and apply an action every nth time. The modulo comes in handy:
1for (let i = 0; i < array.length; i++) {
2 if (i % n === 0) {
3 // Perform the action every nth iteration
4 }
5}
This is just scratching the surface. To deepen your understanding, consider checking out Learn JavaScript, which can help you solidify your knowledge of JavaScript fundamentals.
Practical Applications
Modulus is not just a theoretical concept; it has practical applications. For instance, in web development, you might want to create zebra-striped tables. This is where HTML and CSS come into play. Take a look at HTML Fundamentals Course and Learn CSS Introduction to get started. If you're new to web development as a whole, the Introduction to Web Development course is a great resource.
Zebra Stripes Example
Here's how you might use modulus in JavaScript to alternate row colors:
1const rows = document.querySelectorAll('table tr');
2
3for (let i = 0; i < rows.length; i++) {
4 if (i % 2 === 0) {
5 rows[i].classList.add('even-row');
6 } else {
7 rows[i].classList.add('odd-row');
8 }
9}
Conclusion
You've now stepped through the basics of using modulus in JavaScript! Remember, the modulus operator is more than just a quirky way to find remainders—it's a versatile tool that can simplify your coding tasks and logic. With practice, you'll find even more innovative ways to implement it in your projects.
For further reading and examples, explore reputable sources such as MDN Web Docs or W3Schools. Happy coding!
Remember to keep practicing and exploring the power of modulus in your JavaScript endeavors. As you grow more comfortable with this operator, you'll discover its potential to streamline your code and solve complex problems with elegance and efficiency.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
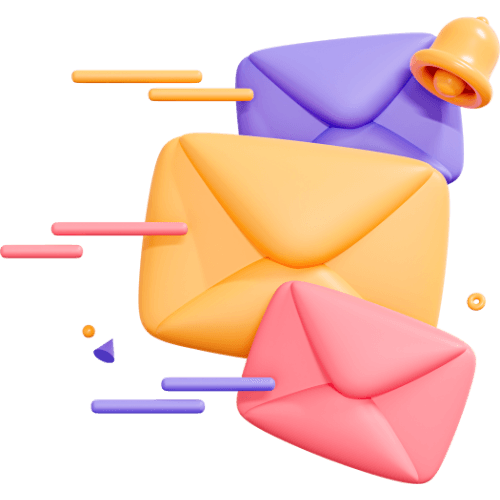
Related articles
9 Articles
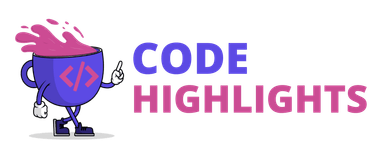
Copyright © Code Highlights 2024.