Ultimate Guide to Multidimensional Array in JavaScript

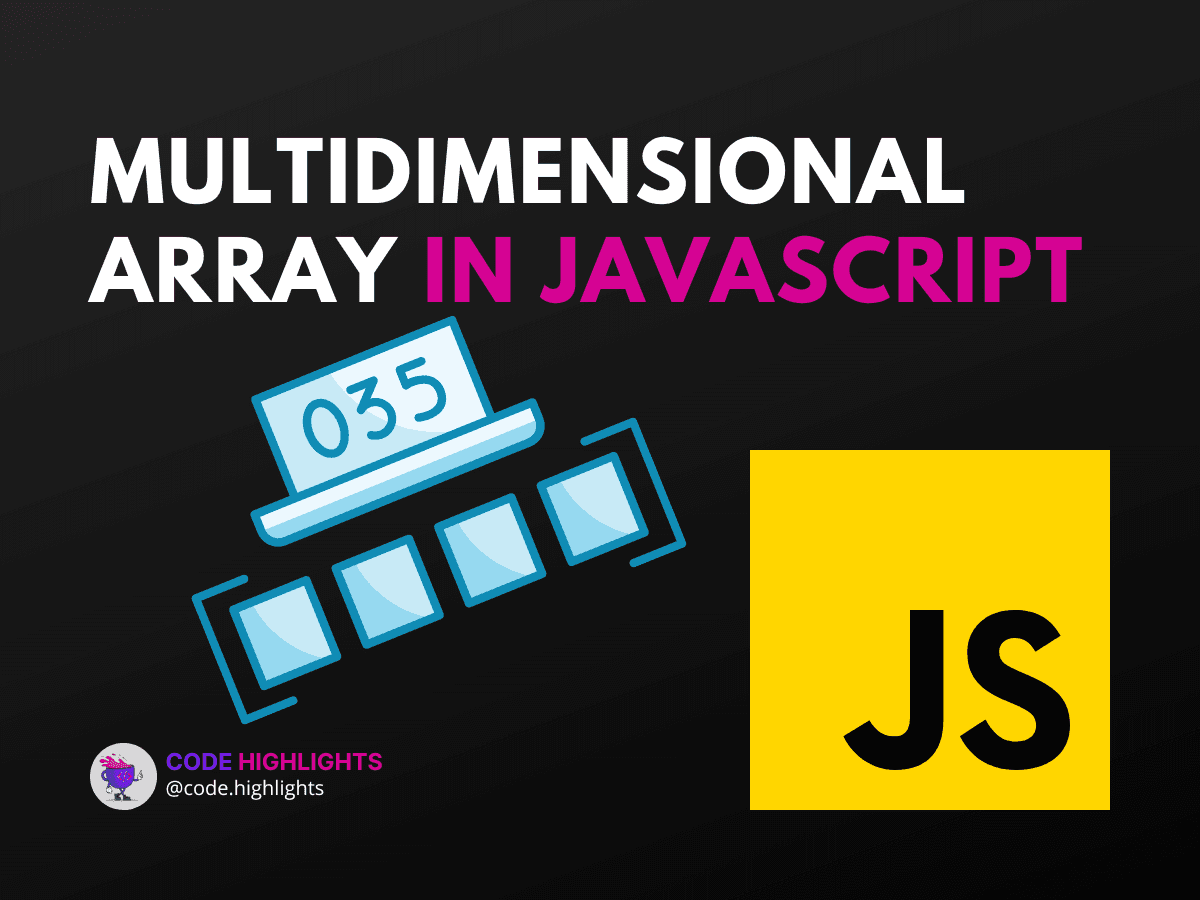
- What is a Multidimensional Array with Example?
- How to Insert a Multidimensional Array in JavaScript?
- How Do You Push a 2 Dimensional Array in JavaScript?
- How to Make a 2D Array in JavaScript?
Welcome to the world of JavaScript arrays, where we delve into the fascinating concept of multidimensional arrays. If you've ever wondered how to manage lists within lists or create a table-like data structure in JavaScript, you're about to find out. This guide is your one-stop resource for understanding and implementing multidimensional arrays in JavaScript.
To kick things off, here's a basic example of a multidimensional array, also known as an array of arrays:
This code snippet represents a simple 3x3 matrix, a perfect starting point for our journey.
What is a Multidimensional Array with Example?
A multidimensional array in JavaScript is an array whose elements are arrays themselves. These nested arrays can contain more arrays, and so on, allowing you to create complex data structures such as matrices, tables, or even tensors.
Here's an example of a 2D array that models a chessboard:
1let chessboard = [
2 ['R', 'N', 'B', 'Q', 'K', 'B', 'N', 'R'],
3 ['P', 'P', 'P', 'P', 'P', 'P', 'P', 'P'],
4 // ... other rows
5 ['p', 'p', 'p', 'p', 'p', 'p', 'p', 'p'],
6 ['r', 'n', 'b', 'q', 'k', 'b', 'n', 'r']
7];
Each inner array represents a row on the chessboard, with individual pieces denoted by letters.
How to Insert a Multidimensional Array in JavaScript?
Inserting a new sub-array into an existing multidimensional array can be done using the push
method if you want to add it at the end of the outer array:
Now, twoDimArray
has a new row, making it [[1, 2], [3, 4], [5, 6]]
.
How Do You Push a 2 Dimensional Array in JavaScript?
Pushing a 2D array in JavaScript is similar to adding any other element. If you have a 2D array and you want to add another array to it, you would do it like this:
With this, we've added a new pair of colors to our collection, expanding the colors
array to include three sub-arrays.
How to Make a 2D Array in JavaScript?
Creating a 2D array from scratch in JavaScript is straightforward. You start by declaring an empty array and then pushing sub-arrays into it:
1let grid = [];
2for (let i = 0; i < 3; i++) {
3 grid[i] = []; // Initialize a new sub-array
4 for (let j = 0; j < 3; j++) {
5 grid[i][j] = i * j; // Assign values
6 }
7}
This loop creates a 3x3 grid with values that are the product of their row and column indices.
Now that you have a solid grasp of multidimensional arrays in JavaScript, why not expand your knowledge further? Check out these related courses to enhance your web development skills:
- For a deeper dive into JavaScript, our Learn JavaScript Course will take your skills to the next level.
- Master the basics of structuring your web pages with our HTML Fundamentals Course.
- Style your sites effectively after learning from our Introduction to CSS.
- Begin your journey in web development with our comprehensive Introduction to Web Development.
In addition to these resources, enrich your understanding with information from reputable sources such as Mozilla Developer Network (MDN), W3Schools, and Stack Overflow for community-driven insights.
By now, you should feel confident working with multidimensional arrays in JavaScript. Remember, practice makes perfect, so experiment with creating and manipulating these arrays to handle data with ease. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
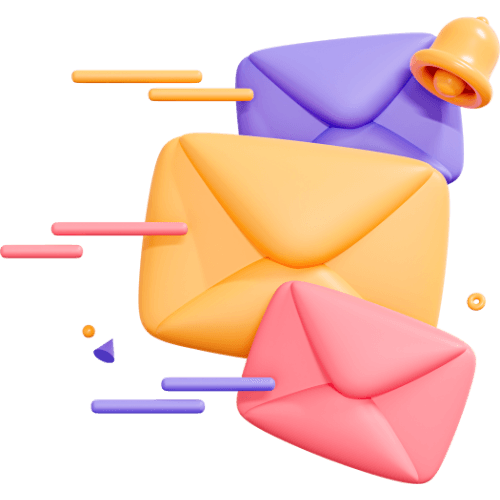
Related articles
9 Articles
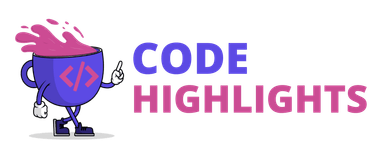
Copyright © Code Highlights 2025.