7 Mistakes to Avoid with Object.entries JavaScript

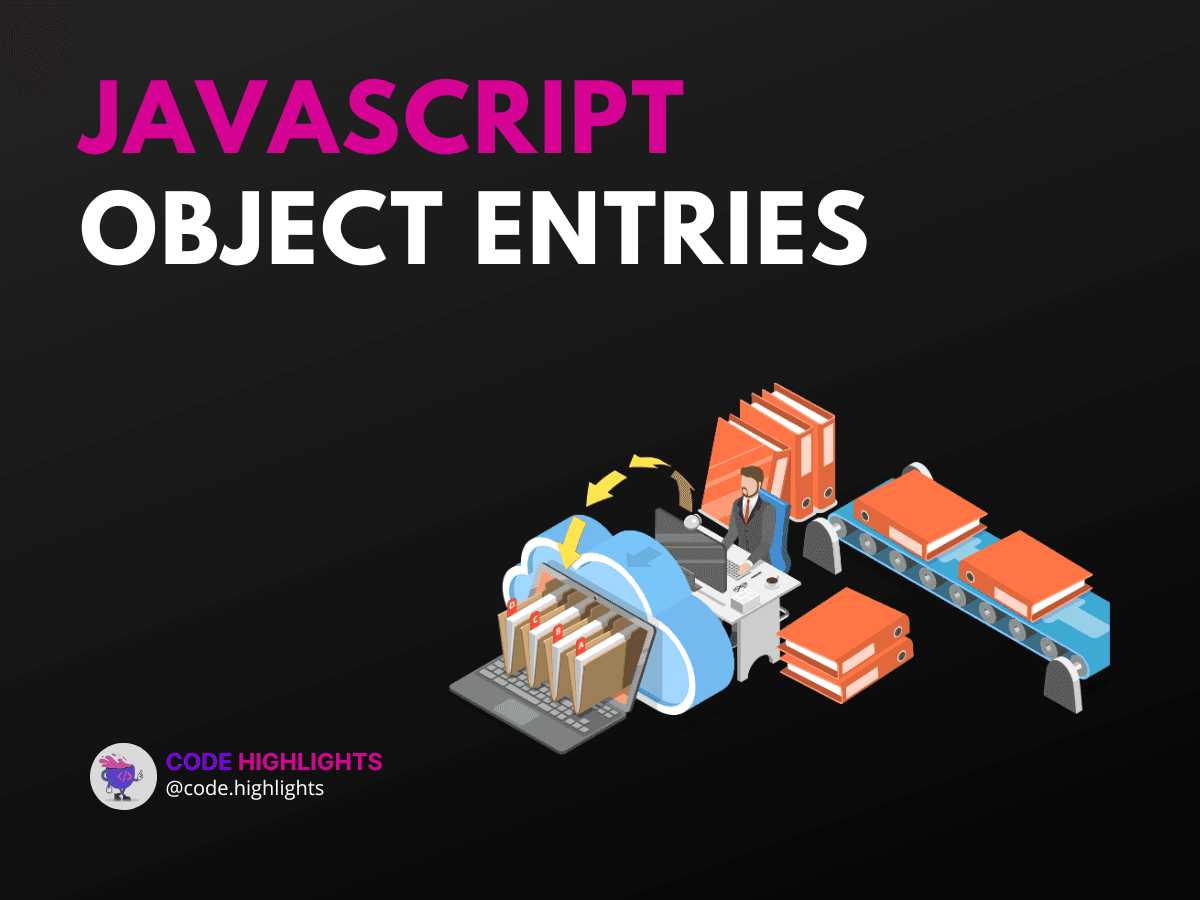
- Understanding Object.entries in JavaScript
- Mistake 1: Not Checking for Empty or Undefined Objects
- Mistake 2: Confusing Object.entries with Object.keys
- Mistake 3: Modifying the Original Object Unintentionally
- Mistake 4: Ignoring the Order of Entries
- Mistake 5: Overlooking the Use of Object.entries for Iteration
- Mistake 6: Not Utilizing Destructuring with Object.entries
- Mistake 7: Adding Entries Incorrectly
- Conclusion
JavaScript offers a plethora of tools for developers to manipulate and interact with objects. One such tool is the Object.entries
method, which can be a game-changer when you need to iterate over object properties. However, misuse or lack of understanding can lead to errors that are both time-consuming and frustrating. In this tutorial, we'll delve into common pitfalls associated with Object.entries
and provide practical examples to help you avoid them.
1// Introductory code example
2const user = {
3 name: 'Alice',
4 age: 25,
5 occupation: 'Developer'
6};
7
8console.log(Object.entries(user));
9// Output: [['name', 'Alice'], ['age', 25], ['occupation', 'Developer']]
By the end of this guide, not only will you be adept at using Object.entries
in JavaScript, but you'll also know how to sidestep the mistakes that could trip you up. Let's enhance your coding skills as we explore the intricacies of Object.entries
together.
Understanding Object.entries
in JavaScript
Before diving into common blunders, let's first address the question: What is Object.entries
in JavaScript? Simply put, Object.entries
is a method that returns an array of a given object's own enumerable string-keyed property [key, value]
pairs. This can be particularly useful for various operations, such as transforming objects into maps, merging objects, or simply iterating over them.
Mistake 1: Not Checking for Empty or Undefined Objects
One common mistake is not checking whether the object passed to Object.entries
is empty or undefined. This can cause your code to throw an error or behave unexpectedly.
1let emptyObject;
2console.log(Object.entries(emptyObject));
3// TypeError: Cannot convert undefined or null to object
To avoid this, always ensure that the object is defined and has properties before calling Object.entries
.
Mistake 2: Confusing Object.entries
with Object.keys
Developers often confuse Object.entries
with Object.keys
, which leads to our next question: What is the difference between Object.entries
and Object.keys
? While Object.keys
returns an array of an object's keys, Object.entries
provides key-value pairs. Knowing when to use each method is crucial for achieving the desired outcome.
Mistake 3: Modifying the Original Object Unintentionally
When working with Object.entries
, it's easy to forget that the method returns a reference to the original object's values. Any changes made to these values will affect the original object.
1const userEntries = Object.entries(user);
2userEntries[1][1] = 30; // Changing the age to 30
3console.log(user.age); // Output: 30
Always clone the values if you need to work with them without altering the original object.
Mistake 4: Ignoring the Order of Entries
Remember that Object.entries
does not guarantee the order of entries. If your code relies on the order, you might encounter bugs. For predictable ordering, consider using a Map
object or sorting the entries post-retrieval.
Mistake 5: Overlooking the Use of Object.entries
for Iteration
Many developers overlook the power of Object.entries
for iteration. By converting an object to entries, you can use array methods like forEach
, map
, filter
, and more.
Mistake 6: Not Utilizing Destructuring with Object.entries
Destructuring enhances the readability and simplicity of your code when working with Object.entries
. It allows you to extract key-value pairs directly within the loop.
Mistake 7: Adding Entries Incorrectly
Adding entries to an object is not the direct responsibility of Object.entries
, but developers often wonder: How to add entries to an object in JavaScript? The correct approach is to assign new properties directly to the object.
And if you're curious about how to get a specific entry from an object in JavaScript, you can do so by accessing the property directly or by finding the entry with the desired key:
1console.log(user.name); // Direct access
2console.log(Object.entries(user).find(entry => entry[0] === 'name')); // Find entry
Conclusion
By understanding and avoiding these seven common mistakes with Object.entries
in JavaScript, you'll write more robust and maintainable code. Remember to check for empty objects, understand the difference between Object.entries
and Object.keys
, avoid unintentional mutations, and embrace the power of iteration and destructuring.
For further learning, consider exploring our comprehensive JavaScript course, deepening your knowledge with our HTML fundamentals course, styling your applications with our CSS introduction, or getting a broader overview with our introduction to web development.
Keep experimenting, keep coding, and stay curious!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
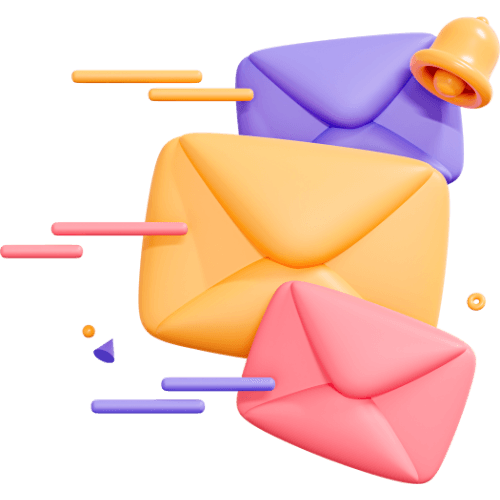
Related articles
9 Articles
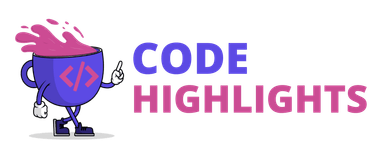
Copyright © Code Highlights 2025.