How to Read Property File in JavaScript: A Step-by-Step Guide

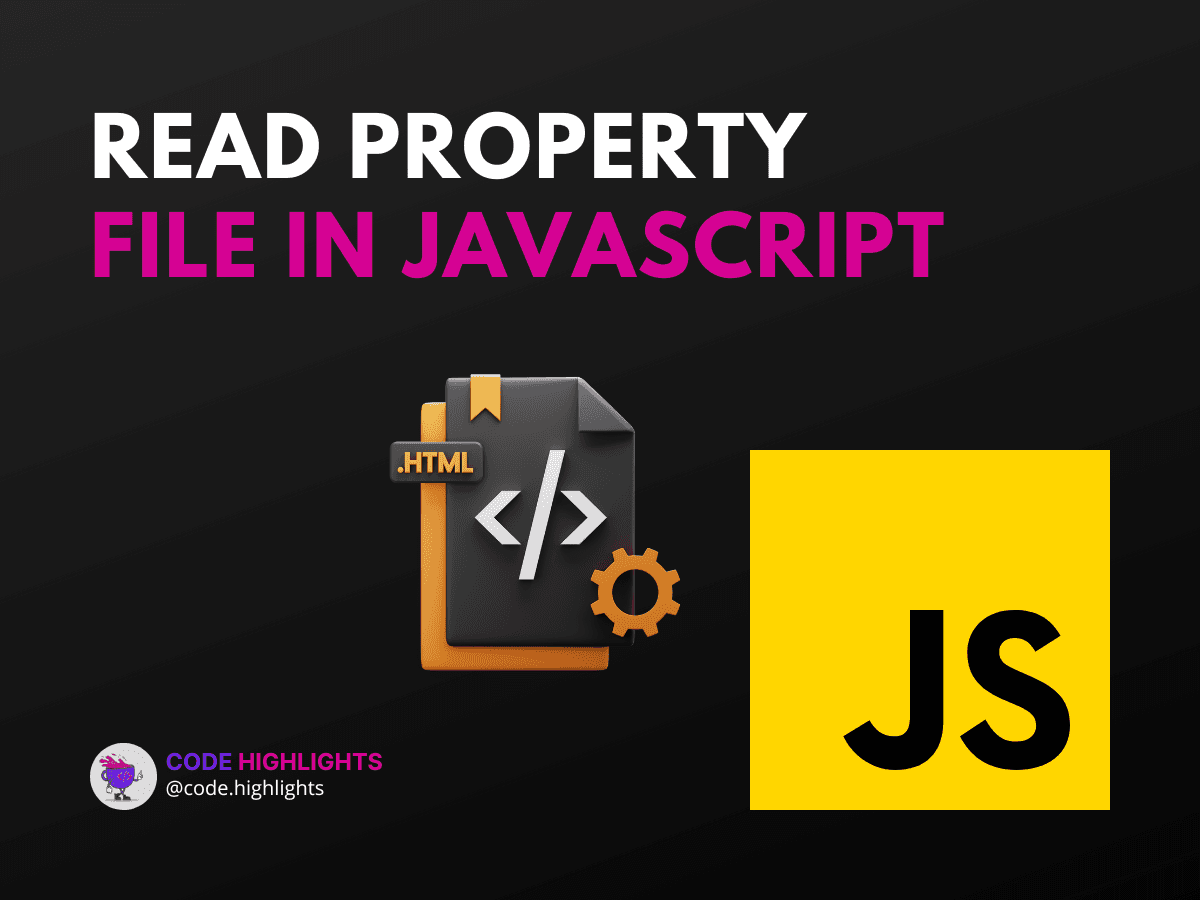
- Step 1: Understanding Property Files
- Step 2: Fetching the Property File
- Step 3: Parsing the Property File
- Step 4: Reading Specific Properties
- Additional Resources
- Conclusion
Have you ever needed to get your hands on specific settings or configurations within a property file using JavaScript? Well, you're in luck because this tutorial will walk you through the process step by step. By the end of this guide, you'll have a solid understanding of how to read property files in JavaScript with ease.
Let's kick things off with a simple example. Imagine you have a config.properties
file with some application settings. Here's a quick peek at what reading a property from this file might look like in JavaScript:
1// Example code snippet to showcase reading a property file
2fetch('/path/to/config.properties')
3 .then(response => response.text())
4 .then(text => console.log(text));
This snippet is just a teaser; we'll delve into the details shortly. If you're eager to learn more about JavaScript, check out this JavaScript course.
Step 1: Understanding Property Files
Before we dive into the code, it's important to know that a property file, often a .properties
file, contains key-value pairs. These files are commonly used to store configuration data and can be easily read by various programming languages, including JavaScript.
Step 2: Fetching the Property File
To read a property file in JavaScript, we first need to fetch it. We can use the fetch
API, which provides an easy and logical way to fetch resources asynchronously across the network.
1fetch('/path/to/config.properties')
2 .then(response => {
3 if (!response.ok) {
4 throw new Error('Network response was not ok');
5 }
6 return response.text();
7 })
8 .then(text => {
9 // We now have the contents of the property file as text
10 // Next, we'll parse this text
11 })
12 .catch(error => {
13 console.error('There has been a problem with your fetch operation:', error);
14 });
For a deeper understanding of web development fundamentals, consider this introduction to web development course.
Step 3: Parsing the Property File
Once we've fetched the file, we need to parse the text to access individual properties. There isn't a built-in parser for property files in JavaScript, but we can write a simple one:
1function parseProperties(text) {
2 const lines = text.split('\n');
3 let properties = {};
4 lines.forEach(line => {
5 // Ignore comments and empty lines
6 if (line && !line.startsWith('#')) {
7 const [key, value] = line.split('=');
8 properties[key.trim()] = value.trim();
9 }
10 });
11 return properties;
12}
Step 4: Reading Specific Properties
Now, to answer the question, "How do I read file Properties?" we simply call our parseProperties
function with the text of the property file:
1fetch('/path/to/config.properties')
2 .then(response => response.text())
3 .then(parseProperties)
4 .then(properties => {
5 console.log(properties['myKey']); // Access the property value using the key
6 });
And there you have it, a straightforward way to access application properties in JavaScript.
Additional Resources
For those interested in expanding their front-end skills, here are some courses worth exploring:
Conclusion
Reading and parsing property files in JavaScript might seem daunting at first, but with the right approach, it becomes a piece of cake. Remember to test your code thoroughly to ensure it works across different scenarios.
For more advanced topics and tutorials, keep exploring our courses and don't hesitate to practice what you've learned. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
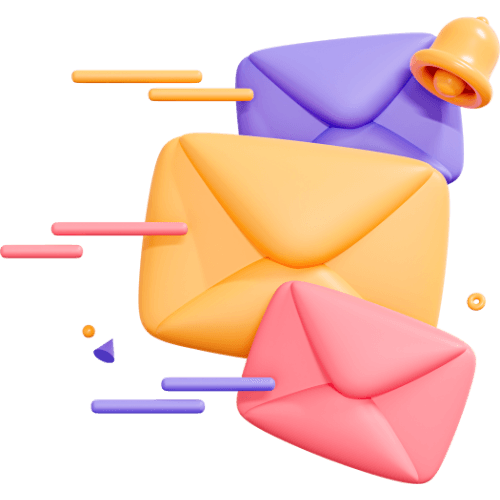
Related articles
9 Articles
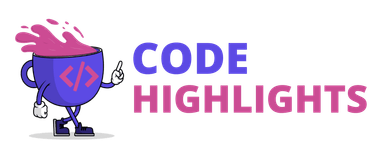
Copyright © Code Highlights 2025.