5 Quick Ways to Remove First Character from String JavaScript

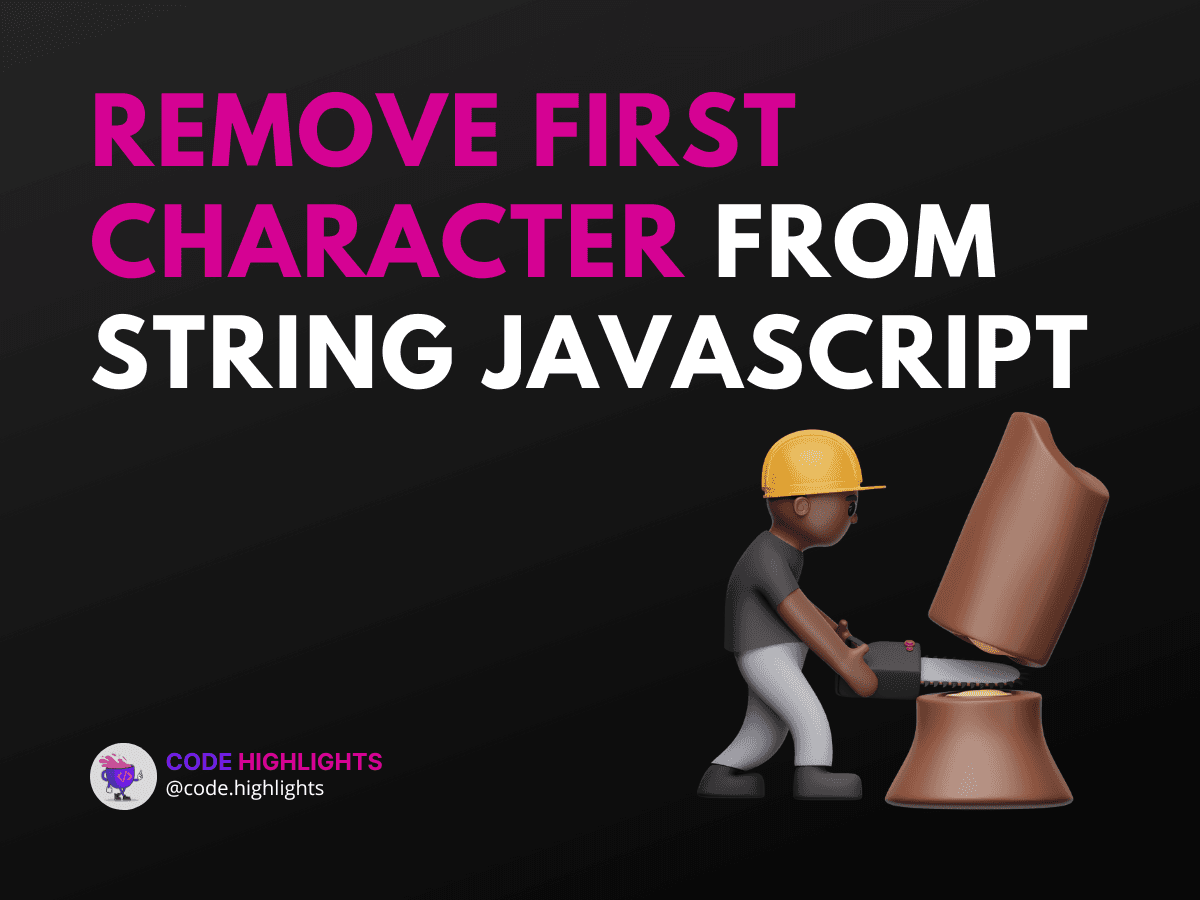
- Quick Code Example
- Method 1: Using the slice() Method
- Syntax
- Example
- Method 2: Using the substring() Method
- Syntax
- Example
- Method 3: Using the substr() Method
- Syntax
- Example
- Method 4: Using Array Methods
- Example
- Method 5: Using Regular Expressions
- Example
- Browser Compatibility
- Summary
When working with strings in JavaScript, you might find yourself needing to remove the first character for various reasons. Whether you're cleaning up user input or processing data, knowing how to manipulate strings is essential. In this tutorial, we’ll explore five quick methods to remove the first character from a string in JavaScript. Let's dive right in!
Quick Code Example
Here's a simple example to get you started. This code snippet demonstrates how to remove the first character using the slice
method:
1let str = "Hello, World!";
2let newStr = str.slice(1);
3console.log(newStr); // Output: "ello, World!"
Method 1: Using the slice()
Method
The slice()
method is a powerful way to extract a portion of a string. By specifying the starting index, you can easily remove the first character.
Syntax
- startIndex: The index to start extraction (0-based).
- endIndex: The index to stop extraction (optional).
Example
1let greeting = "Goodbye!";
2let result = greeting.slice(1);
3console.log(result); // Output: "oodbye!"
Method 2: Using the substring()
Method
Another option is the substring()
method. This method works similarly to slice()
, but it has different handling of negative indices.
Syntax
Example
1let message = "Welcome!";
2let modifiedMessage = message.substring(1);
3console.log(modifiedMessage); // Output: "elcome!"
Method 3: Using the substr()
Method
The substr()
method allows you to specify the starting position and the number of characters to return.
Syntax
Example
1let text = "JavaScript";
2let newText = text.substr(1);
3console.log(newText); // Output: "avaScript"
Method 4: Using Array Methods
You can also convert the string into an array, remove the first character, and then join it back into a string.
Example
1let strArray = "Programming".split('');
2strArray.shift();
3let finalString = strArray.join('');
4console.log(finalString); // Output: "rogramming"
Method 5: Using Regular Expressions
Regular expressions provide a flexible way to manipulate strings. You can use them to replace the first character with an empty string.
Example
1let regexString = "Hello, World!";
2let updatedString = regexString.replace(/^./, '');
3console.log(updatedString); // Output: "ello, World!"
Browser Compatibility
All methods discussed above are widely supported across major web browsers, including Chrome, Firefox, Safari, and Edge. This ensures that your code will run smoothly regardless of the user's browser choice.
Summary
In this tutorial, we've covered five quick ways to remove the first character from a string in JavaScript. Understanding these methods—slice()
, substring()
, substr()
, array manipulation, and regular expressions—can help you efficiently handle string operations in your projects. For more in-depth learning, consider checking out our courses on JavaScript and HTML Fundamentals.
By mastering these techniques, you'll be better equipped to handle string manipulation in your web development tasks. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
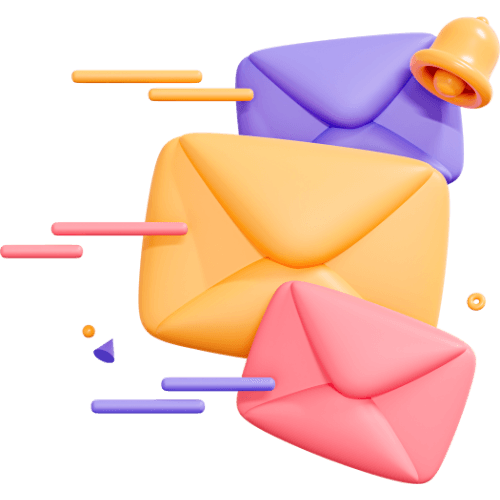
Related articles
9 Articles
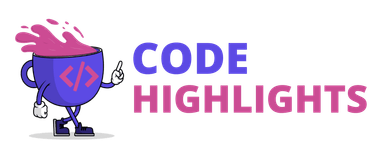
Copyright © Code Highlights 2025.