7 Simple Ways to Remove Last Character from String JavaScript

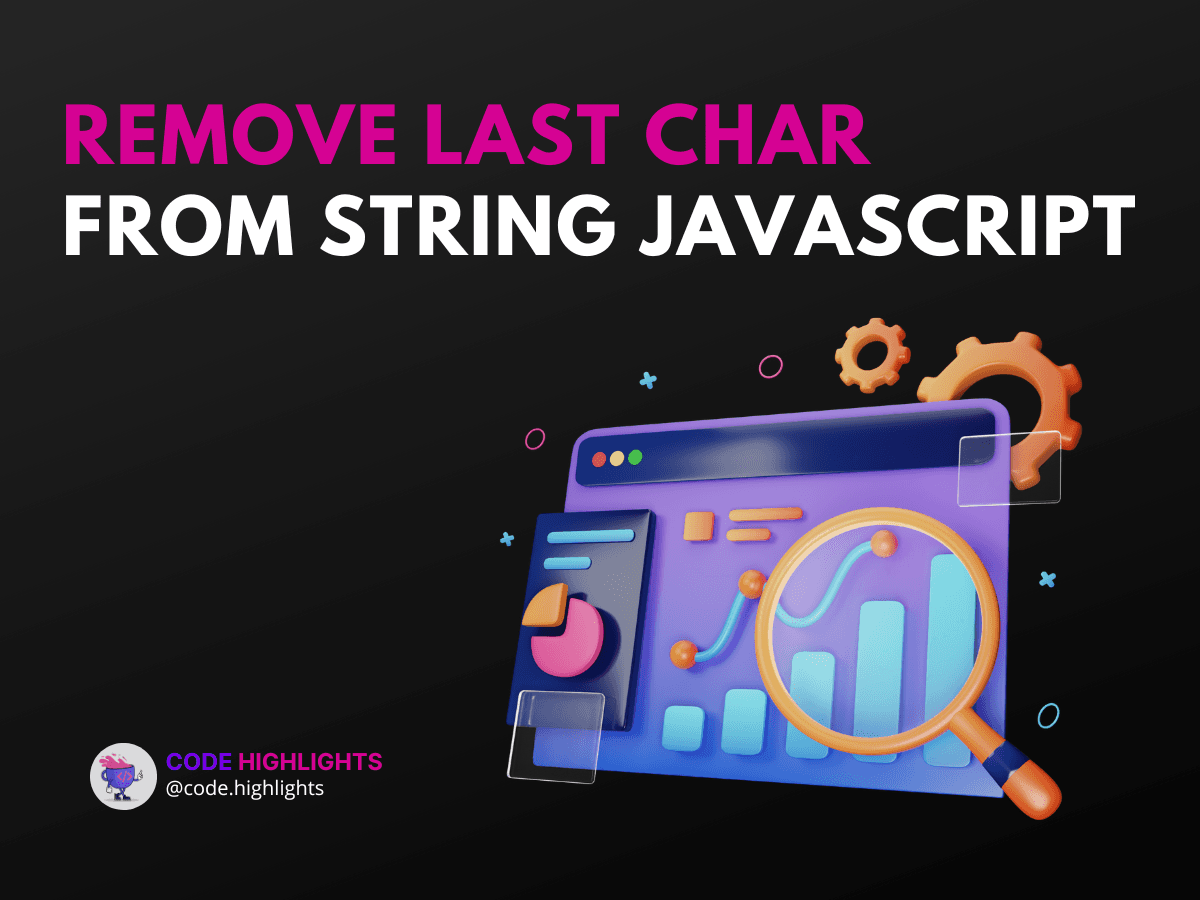
- Quick Code Example
- 1. Using the slice() Method
- Syntax
- Example
- 2. Using the substring() Method
- Syntax
- Example
- 3. Using the substr() Method
- Syntax
- Example
- 4. Using the slice() Method with a Variable
- Example
- 5. Using Regular Expressions
- Example
- 6. Using Array Methods
- Example
- 7. Using String Length in a Loop
- Example
- Browser Compatibility
- Summary
When working with strings in JavaScript, you might find yourself needing to remove the last character for various reasons. Whether you're cleaning up user input or manipulating data, knowing how to efficiently remove the last character from a string can be quite handy. In this tutorial, we will explore seven simple methods to achieve this goal, complete with code examples and explanations.
Quick Code Example
Here's a quick example to get started:
In this example, we use the slice
method to remove the last character from the string. Let’s dive deeper into different methods.
1. Using the slice()
Method
The slice()
method is one of the most common ways to remove the last character from a string.
Syntax
- startIndex: The index at which to start the slice.
- endIndex: The index at which to end the slice (not included).
Example
This method works by slicing the string from the start to the second-to-last character.
2. Using the substring()
Method
Another option is to use the substring()
method, which extracts characters between two specified indices.
Syntax
Example
1let str = "Programming";
2str = str.substring(0, str.length - 1);
3console.log(str); // Outputs: Programm
Here, we specify the starting index as 0
and the ending index as the length minus one.
3. Using the substr()
Method
The substr()
method can also be used to remove the last character.
Syntax
Example
1let str = "Hello World";
2str = str.substr(0, str.length - 1);
3console.log(str); // Outputs: Hello Worl
In this case, we take the substring from the start index to the length minus one.
4. Using the slice()
Method with a Variable
You can store the length of the string in a variable for better readability.
Example
1let str = "OpenAI";
2let len = str.length;
3str = str.slice(0, len - 1);
4console.log(str); // Outputs: OpenA
This approach makes it clear what you're doing with the length of the string.
5. Using Regular Expressions
Regular expressions can also be used to remove the last character.
Example
In this example, the replace()
method uses a regex pattern to match and remove the last character.
6. Using Array Methods
You can convert the string to an array, manipulate it, and then join it back to a string.
Example
1let str = "Array Method";
2str = str.split('').slice(0, -1).join('');
3console.log(str); // Outputs: Array Metho
This method splits the string into an array, removes the last element, and then joins it back together.
7. Using String Length in a Loop
Lastly, you can use a loop to remove the last character, though it's less efficient.
Example
1let str = "Loop Example";
2for (let i = 0; i < str.length - 1; i++) {
3 str = str[i];
4}
5console.log(str); // Outputs: L
This method iterates through the string but is not recommended for performance reasons.
Browser Compatibility
All the methods mentioned above are compatible with major web browsers, including Chrome, Firefox, Safari, and Edge. You can confidently use them in your web applications.
Summary
In this tutorial, we explored seven simple ways to remove the last character from a string in JavaScript. From using built-in methods like slice()
, substring()
, and substr()
, to employing regular expressions and array manipulation, you now have a variety of options to choose from. Understanding these techniques can help you handle string data more effectively in your projects. For more on JavaScript, check out our JavaScript course or learn about HTML fundamentals and CSS introduction.
If you're interested in diving deeper into web development, consider taking our introduction to web development course. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
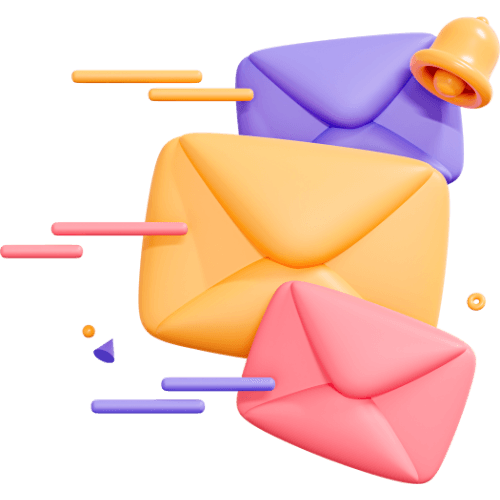
Related articles
9 Articles
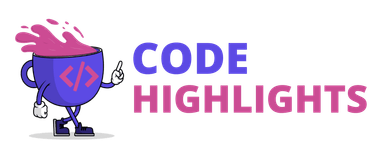
Copyright © Code Highlights 2025.