How to Remove Non Alphanumeric Characters JavaScript for Cleaner Code

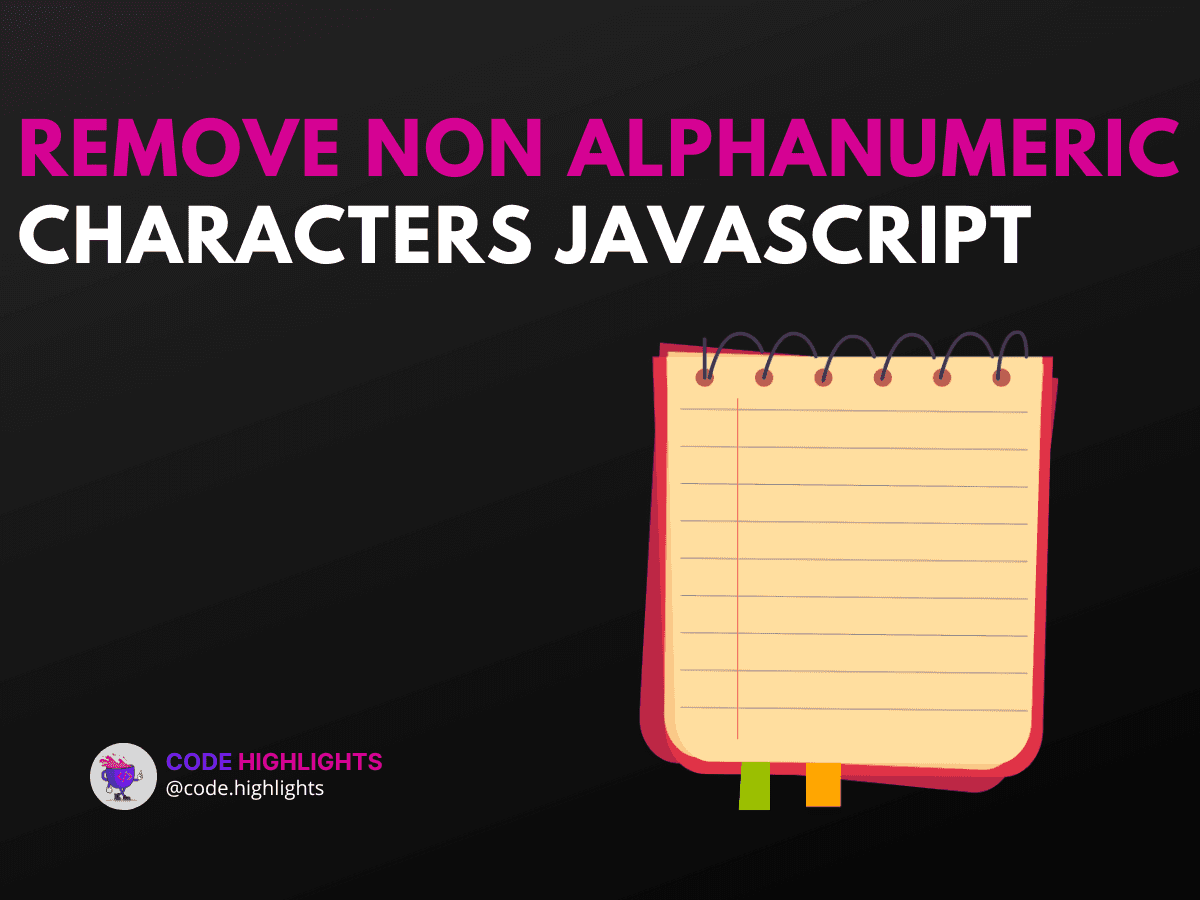
- Understanding the Syntax
- Parameters:
- Return Value:
- Variations:
- Example 1: Basic Removal of Non-Alphanumeric Characters
- Example 2: Removing Non-Numeric Characters
- Example 3: Advanced Use Case with Spaces
- Compatibility with Major Browsers
- Summary
When working with strings in JavaScript, you might encounter non-alphanumeric characters that can clutter your data. Removing these characters is crucial for cleaner code and better data processing. In this tutorial, we will explore how to remove non-alphanumeric characters in JavaScript, making your code cleaner and more efficient.
Here's a simple example to get you started:
1let inputStr = "Hello, World! 123.";
2let cleanStr = inputStr.replace(/[^a-zA-Z0-9]/g, '');
3console.log(cleanStr); // Output: HelloWorld123
Understanding the Syntax
To remove non-alphanumeric characters, we often use the String.prototype.replace()
method. The syntax looks like this:
Parameters:
- regexp: A regular expression used to find matches.
- substr: A string to be replaced.
- newSubStr: The string to replace the matched substring.
- function: A function to generate the replacement string.
Return Value:
The method returns a new string with the replacements made.
Variations:
You can use different regular expressions to target specific characters. For example, /[^a-zA-Z0-9]/g
removes anything that isn't a letter or number.
Example 1: Basic Removal of Non-Alphanumeric Characters
In this example, we'll remove all non-alphanumeric characters from a string:
1let exampleStr = "JavaScript is fun! @2023";
2let result = exampleStr.replace(/[^a-zA-Z0-9]/g, '');
3console.log(result); // Output: JavaScriptisfun2023
Example 2: Removing Non-Numeric Characters
Sometimes, you only want to keep numeric values. Here's how to do that:
1let numStr = "Phone: (123) 456-7890";
2let onlyNumbers = numStr.replace(/[^0-9]/g, '');
3console.log(onlyNumbers); // Output: 1234567890
Example 3: Advanced Use Case with Spaces
If you want to preserve spaces between words while removing other non-alphanumeric characters, try this:
1let mixedStr = "Hello! How are you? 123.";
2let cleanedWithSpaces = mixedStr.replace(/[^a-zA-Z0-9\s]/g, '');
3console.log(cleanedWithSpaces); // Output: Hello How are you 123
Compatibility with Major Browsers
The replace()
method and regular expressions are well-supported across all major browsers, including Chrome, Firefox, Safari, and Edge. This means you can confidently use these methods in your web development projects. For more on browser compatibility, check out MDN Web Docs.
Summary
In this tutorial, we explored how to remove non-alphanumeric characters in JavaScript. We learned about the replace()
method, its syntax, and how to use regular expressions to clean up strings effectively. By employing these techniques, you can ensure your data is clean and ready for further processing.
For more in-depth learning, consider checking out our courses on JavaScript, HTML fundamentals, and CSS introduction.
Remember, cleaning your data not only enhances readability but also improves performance in your applications. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
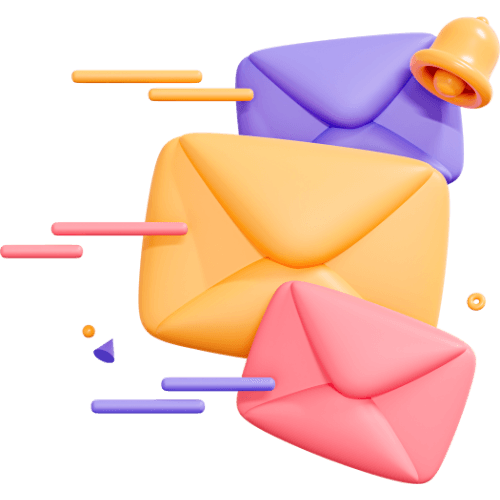
Related articles
9 Articles
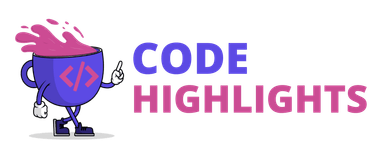
Copyright © Code Highlights 2025.