How to Remove Numbers from String JavaScript for Cleaner Data

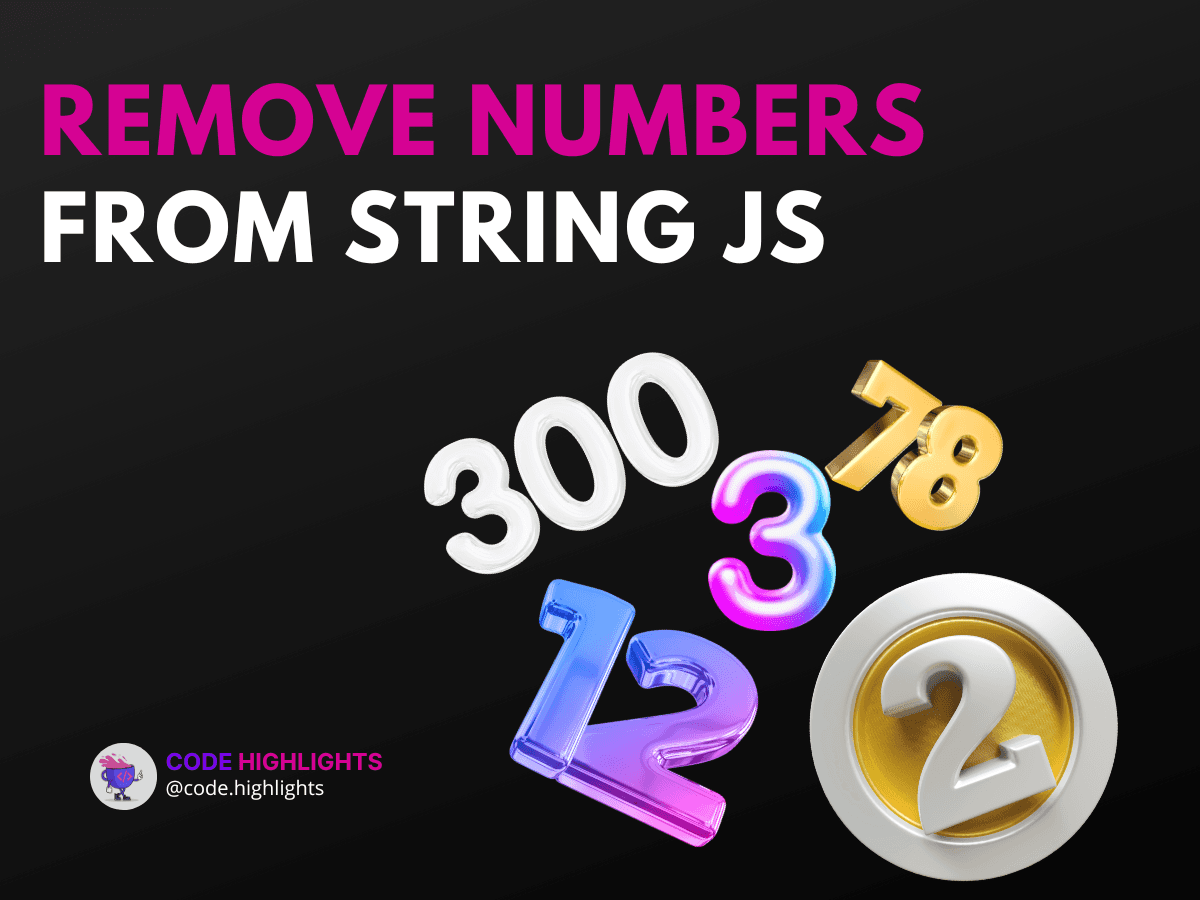
- Quick Code Example
- Understanding the Syntax
- Example 1: Basic Number Removal
- Removing All Digits
- Example 2: Removing Specific Digits
- Removing Only Certain Digits
- Example 3: Extracting Digits
- How to Extract Digits from a String
- Example 4: Using Function for Reusability
- Creating a Function to Remove Numbers
- Browser Compatibility
- Summary
When working with data in JavaScript, you might find yourself needing to clean up strings by removing unwanted numbers. This is especially useful when you're processing user input or handling data from various sources. In this tutorial, we'll explore different ways to remove numbers from string JavaScript and make your data cleaner and more manageable.
Quick Code Example
To kick things off, here’s a simple example that demonstrates how to remove numbers from a string:
1let originalString = "Hello123 World456!";
2let cleanedString = originalString.replace(/[0-9]/g, '');
3console.log(cleanedString); // Output: "Hello World!"
This code uses a regular expression to find and remove all digits from the string.
Understanding the Syntax
In the example above, we used the replace()
method combined with a regular expression. The syntax looks like this:
-
Parameters:
regexp
: This is the regular expression used to match the characters you want to replace. In our case,/[0-9]/g
matches all digits.newSubstr
: This is what we want to replace the matched characters with. Here, we use an empty string (''
) to remove the numbers.
-
Return Value: The method returns a new string with the specified replacements.
Example 1: Basic Number Removal
Removing All Digits
Here’s a straightforward example that removes all digits from a string:
1let text = "I have 2 apples and 3 oranges.";
2let result = text.replace(/[0-9]/g, '');
3console.log(result); // Output: "I have apples and oranges."
This code snippet shows how easy it is to clean up a string by removing numbers.
Example 2: Removing Specific Digits
Removing Only Certain Digits
If you only want to remove specific digits, you can modify the regular expression:
1let text = "My phone number is 123-456-7890.";
2let result = text.replace(/[1-3]/g, '');
3console.log(result); // Output: "My phone number is 24-56-7890."
In this case, we removed only the digits 1, 2, and 3 from the string.
Example 3: Extracting Digits
How to Extract Digits from a String
Sometimes, you may want to extract the digits instead of removing them. You can do this using the match()
method:
1let mixedString = "The year is 2023.";
2let digits = mixedString.match(/[0-9]+/g);
3console.log(digits); // Output: ["2023"]
This example shows how to extract numbers from a string using a regular expression.
Example 4: Using Function for Reusability
Creating a Function to Remove Numbers
You can create a reusable function to remove numbers from any string:
1function removeNumbers(str) {
2 return str.replace(/[0-9]/g, '');
3}
4
5let myString = "Remove 123 numbers from this string!";
6console.log(removeNumbers(myString)); // Output: "Remove numbers from this string!"
This function makes it easy to clean multiple strings without repeating code.
Browser Compatibility
The methods discussed in this tutorial are widely supported across major web browsers like Chrome, Firefox, Safari, and Edge. You can confidently use these techniques in your web applications.
Summary
In this tutorial, we covered how to remove numbers from string JavaScript effectively. We explored several methods, including using regular expressions with the replace()
method and creating reusable functions. By cleaning up your data, you can ensure better quality and usability in your applications.
For more in-depth learning, check out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. Additionally, if you're interested in broader web development topics, take a look at our Introduction to Web Development.
For further reading, you might find these resources helpful:
Now you're equipped to handle strings in JavaScript like a pro!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
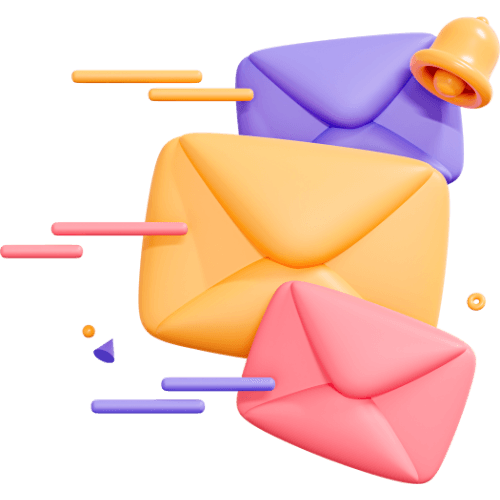
Related articles
9 Articles
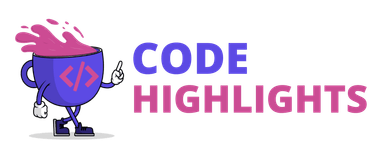
Copyright © Code Highlights 2025.