How to RemoveEventListener JavaScript for Clean Code

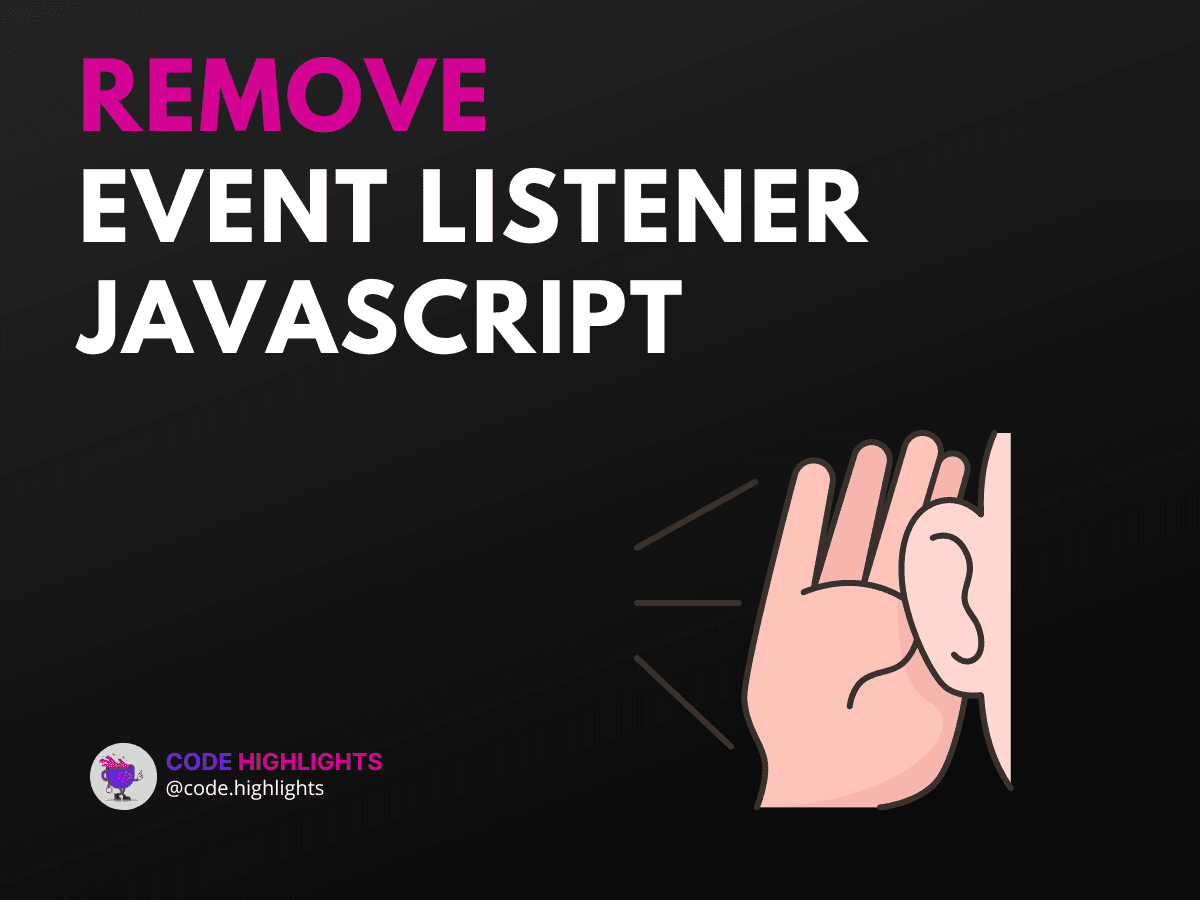
- Understanding the Syntax
- Example: Basic Usage of removeEventListener
- Example: Why You Should Remove Event Listeners
- Example: Using removeEventListener with Anonymous Functions
- Clearing the Event Queue
- Compatibility with Major Web Browsers
- Key Takeaways
When working with JavaScript, managing event listeners is essential for creating efficient and clean code. Event listeners help you respond to user actions, like clicks or keyboard inputs. However, if you don't remove these listeners when they're no longer needed, they can lead to memory leaks and performance issues. This tutorial will guide you through using removeEventListener
effectively.
Here’s a simple code example to get started:
1function handleClick() {
2 console.log('Button clicked!');
3}
4
5const button = document.getElementById('myButton');
6button.addEventListener('click', handleClick);
7
8// Later, when you want to stop listening:
9button.removeEventListener('click', handleClick);
Understanding the Syntax
The syntax for removeEventListener
is straightforward. It looks like this:
- element: The DOM element from which you want to remove the listener.
- event: A string representing the event type (e.g., "click").
- function: The specific function that was added as an event listener.
- useCapture: A boolean indicating whether to remove the event listener in the capturing phase. This parameter is optional and defaults to
false
.
Example: Basic Usage of removeEventListener
Here's a simple example demonstrating how to use removeEventListener
:
1const button = document.getElementById('myButton');
2
3function logMessage() {
4 console.log('Button was clicked');
5}
6
7button.addEventListener('click', logMessage);
8
9// Now, let's remove the event listener
10button.removeEventListener('click', logMessage);
In this case, after calling removeEventListener
, clicking the button will not log any messages.
Example: Why You Should Remove Event Listeners
Should I remove event listeners in JavaScript? Absolutely! Not removing them can cause unwanted behavior, such as multiple triggers of the same event. Here’s an example:
1const checkbox = document.getElementById('myCheckbox');
2
3function toggleMessage() {
4 console.log('Checkbox toggled');
5}
6
7checkbox.addEventListener('change', toggleMessage);
8
9// If you don't remove it, each time you check/uncheck, it logs again.
10checkbox.removeEventListener('change', toggleMessage);
This ensures that the function only runs once per change.
Example: Using removeEventListener with Anonymous Functions
It’s important to note that if you add an event listener using an anonymous function, you cannot remove it later. For instance:
1const button = document.getElementById('myButton');
2
3button.addEventListener('click', function () {
4 console.log('Clicked!');
5});
6
7// This will NOT work because the function is anonymous
8button.removeEventListener('click', function () {
9 console.log('Clicked!');
10});
To remove an event listener, always define the function separately.
Clearing the Event Queue
How to clear the event queue in JavaScript? While you can't directly clear the event queue, you can control when events are processed by using methods like stopPropagation()
or preventDefault()
. These methods help manage how events are handled and can prevent unnecessary execution.
Compatibility with Major Web Browsers
The removeEventListener
method is widely supported across all major browsers, including Chrome, Firefox, Safari, and Edge. Always ensure your code is compatible by testing across different platforms.
Key Takeaways
In conclusion, using removeEventListener
in JavaScript is crucial for maintaining clean and efficient code. By properly removing event listeners, you can prevent memory leaks and ensure your application runs smoothly. Always remember to match your addEventListener
calls with corresponding removeEventListener
calls.
For more insights on JavaScript, check out our JavaScript course. If you're looking to strengthen your web development skills, consider exploring our HTML Fundamentals Course and CSS Introduction.
By following these practices, you’ll be well on your way to writing cleaner, more efficient JavaScript code. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
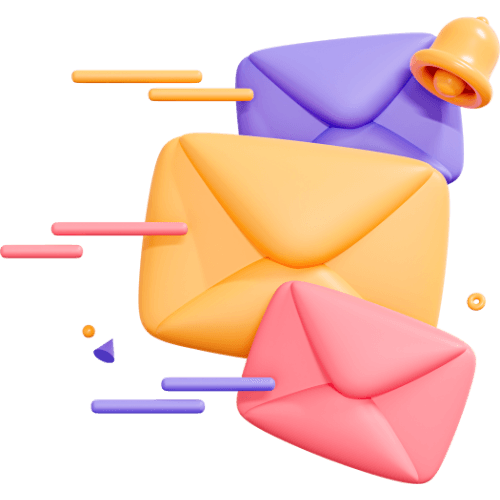
Related articles
9 Articles
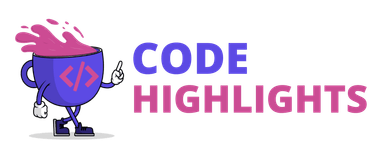
Copyright © Code Highlights 2024.