5 Quick Methods to Replace Space with Underscore JavaScript

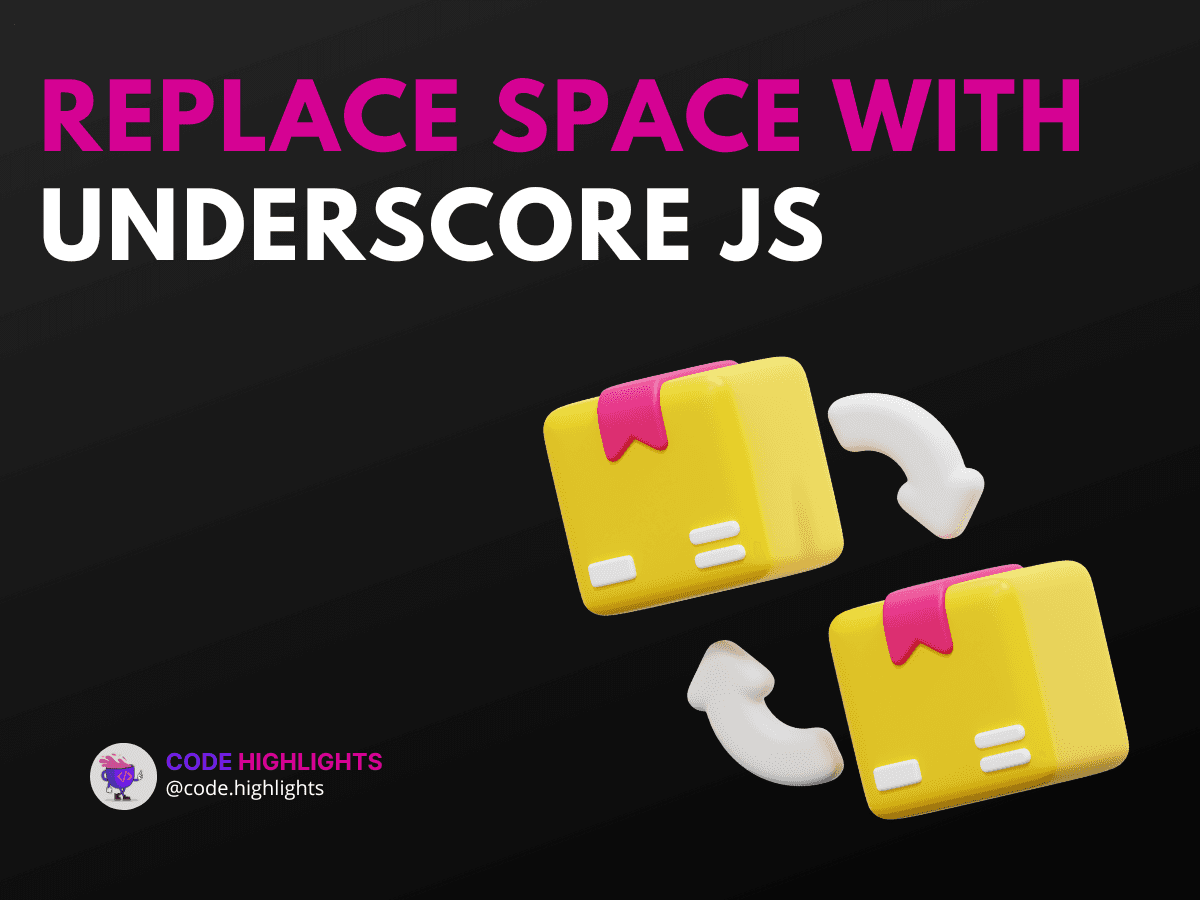
- Basic Example
- Method 1: Using String.replace()
- Syntax
- Example
- Method 2: Using split() and join()
- Syntax
- Example
- Method 3: Using Regular Expressions
- Example
- Method 4: Using Array.map()
- Example
- Method 5: Using a Custom Function
- Example
- Browser Compatibility
- Summary
Have you ever needed to replace spaces in a string with underscores? This is a common task in programming, especially when preparing data for URLs or file names. In this tutorial, we’ll explore five quick methods to replace space with underscore in JavaScript. Each method has its own syntax and use cases, so let’s dive right in!
Basic Example
Before we start, here’s a simple code snippet demonstrating how to replace spaces with underscores:
1let str = "Hello World";
2let newStr = str.replace(/ /g, "_");
3console.log(newStr); // Output: Hello_World
This code uses the replace
method with a regular expression to find spaces and replace them with underscores.
Method 1: Using String.replace()
The most straightforward way to replace spaces is by using the replace()
method. Here’s how it works:
Syntax
- searchValue: The value to search for (can be a string or regex).
- newValue: The value to replace with.
Example
1let str = "JavaScript is fun";
2let result = str.replace(/ /g, "_");
3console.log(result); // Output: JavaScript_is_fun
This method is effective for single replacements, but using the global flag (g
) ensures all spaces are replaced.
Method 2: Using split() and join()
Another way to replace spaces is by using split()
and join()
. This method breaks the string into an array and then joins it back together.
Syntax
Example
1let str = "Learn JavaScript Easily";
2let result = str.split(" ").join("_");
3console.log(result); // Output: Learn_JavaScript_Easily
This approach can be helpful if you want to replace multiple characters at once.
Method 3: Using Regular Expressions
Regular expressions can be powerful for complex replacements. You can use them to target spaces specifically.
Example
1let str = "Replace all spaces!";
2let result = str.replace(/\s+/g, "_");
3console.log(result); // Output: Replace_all_spaces!
Here, \s+
matches all whitespace characters, making it versatile for any spaces.
Method 4: Using Array.map()
You can also use Array.map()
in combination with split()
to replace spaces. This method is useful for more complex transformations.
Example
1let str = "Spaces between words";
2let result = str.split(" ").map(word => word.replace(" ", "_")).join("_");
3console.log(result); // Output: Spaces_between_words
This method gives you more control over each word before joining them back together.
Method 5: Using a Custom Function
Creating a custom function allows for flexibility and reusability. You can define your logic for replacing spaces.
Example
1function replaceSpaceWithUnderscore(input) {
2 return input.replace(/ /g, "_");
3}
4
5let str = "Custom function example";
6let result = replaceSpaceWithUnderscore(str);
7console.log(result); // Output: Custom_function_example
This function can be reused across different parts of your application.
Browser Compatibility
All the methods mentioned above are widely supported in major web browsers, including Chrome, Firefox, Safari, and Edge. This means you can confidently use them in your web projects without compatibility issues.
Summary
In this tutorial, we explored five quick methods to replace space with underscore in JavaScript. From using the replace()
method to creating a custom function, each approach has its unique benefits. Whether you need a simple replacement or a more complex solution, these methods will help you handle spaces effectively. For more on JavaScript, check out our JavaScript course.
If you're interested in learning more about web development, consider looking into our HTML fundamentals course or CSS introduction. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
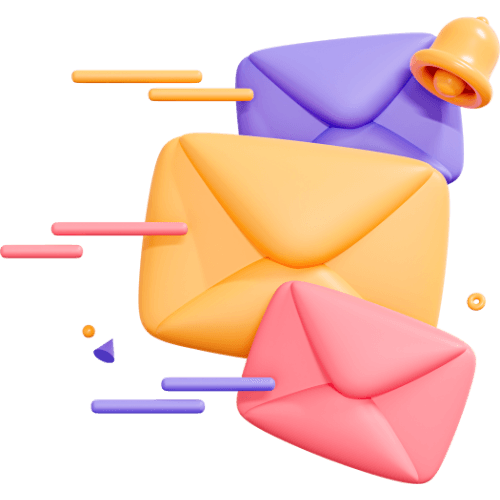
Related articles
9 Articles
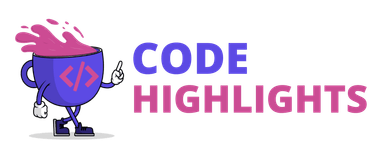
Copyright © Code Highlights 2025.