Uncaught ReferenceError: require is not defined

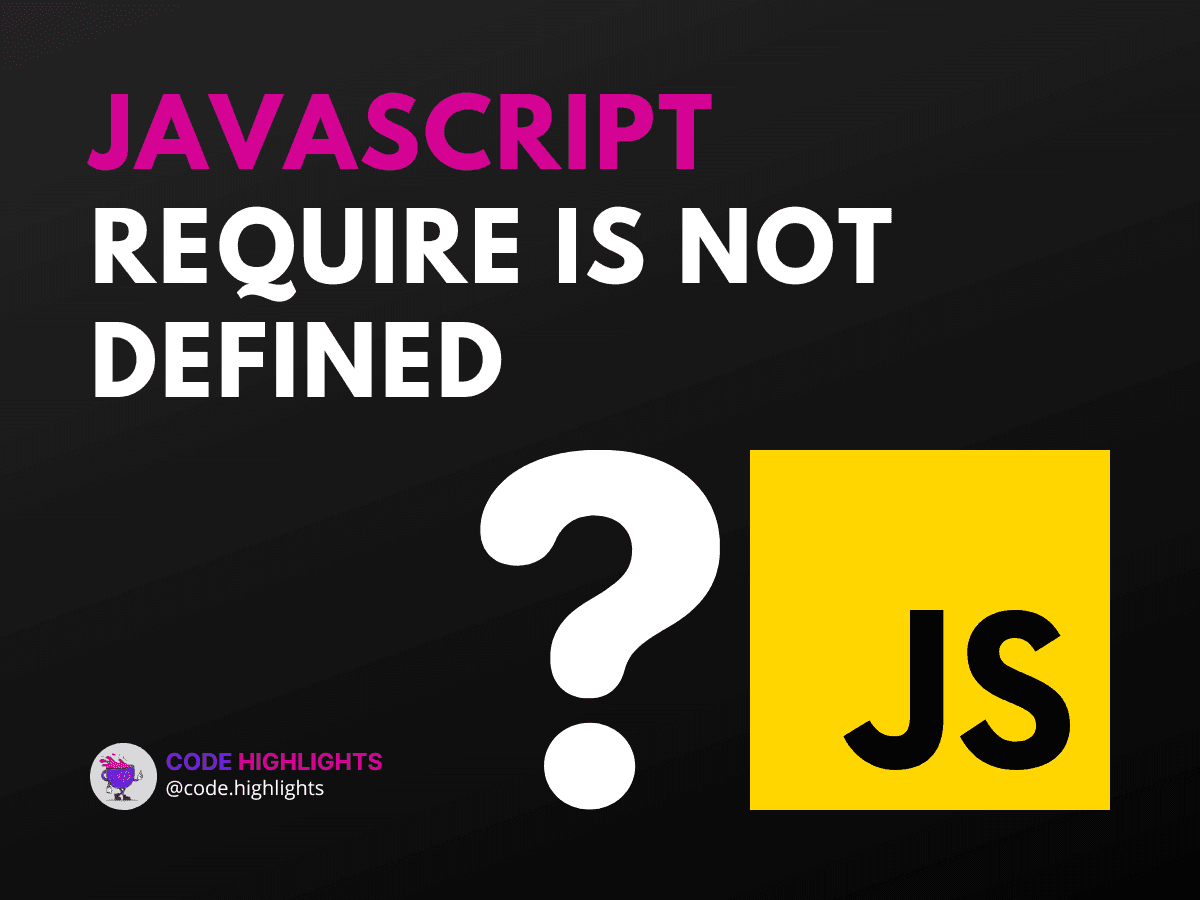
- Why is require() Not Defined?
- "require is not defined" in Production
- ES6 and the require Function
- What Does "Not Defined" Mean in Code?
- Resolving the Error
JavaScript is an incredibly versatile language, powering the dynamic aspects of websites and applications. However, like any programming language, it has its fair share of error messages that can stump developers. One such message is the "require is not defined" error. This tutorial will demystify this common issue, helping you to understand why it occurs and how to resolve it.
Let's start with a bit of code to illustrate:
This line might look correct, especially if you're coming from a Node.js background. However, if you've included it in a front-end JavaScript file, you're likely to encounter the "require is not defined" error. Why does this happen? Let's find out.
Why is require()
Not Defined?
The require()
function is a part of CommonJS module syntax, which is widely used in Node.js for including modules. However, when you're working within a browser environment, this function isn't available by default. Browsers use a different mechanism for loading modules, which leads to the "require is not defined" error when you try to use require()
.
If you're interested in furthering your JavaScript knowledge, consider exploring Learn JavaScript to deepen your understanding of the language and its environment-specific behaviors.
"require is not defined" in Production
Sometimes, you might encounter the "require is not defined" error only when your code goes into production. This typically happens when the build process for your application does not correctly transpile or bundle your modules. Tools like Webpack or Babel can transform your CommonJS modules into a format that's browser-friendly during development but may be misconfigured for production builds.
To ensure a smooth transition from development to production, it's essential to have a solid grasp of the build process. You can learn more about the underlying HTML structures in HTML Fundamentals Course, which can serve as a foundation for understanding how scripts are included in web pages.
ES6 and the require
Function
With the introduction of ECMAScript 6 (ES6), JavaScript received its own native module system using import
and export
statements. If you're working with ES6, you might wonder, "What is require is not defined in ES6?" The answer is straightforward: ES6 modules do not recognize the require()
function because they rely on the import
statement to load modules. Here's an example of how to use ES6 modules:
Learning ES6 can be a game-changer for your web development skills. Check out Introduction to Web Development to get up to speed with modern JavaScript practices, including ES6 modules.
What Does "Not Defined" Mean in Code?
When JavaScript throws a "not defined" error, it means that the script is trying to access a variable or function that has not been declared within the current scope. In the case of "require is not defined," the script is attempting to call the require()
function, which is not recognized in the browser's global scope.
Understanding the scope and declaration of variables is critical in JavaScript. To strengthen your CSS skills alongside JavaScript, take a look at Learn CSS Introduction to create visually compelling web pages.
Resolving the Error
To fix the "require is not defined" error, you have a few options:
-
Use a Module Bundler: Tools like Webpack or Browserify allow you to write CommonJS modules that will be bundled into a single script file, suitable for browser use.
-
Switch to ES6 Modules: If you can, refactor your code to use ES6
import
andexport
statements, which are becoming standard for modern web development. -
Include a Module Loader: Libraries like RequireJS provide a way to load CommonJS modules in the browser.
-
Use a CDN: Some modules are available via CDNs and can be included directly in your HTML with a
<script>
tag.
By understanding the context of the "require is not defined" error and knowing how to address it, you'll be better equipped to develop JavaScript applications that work seamlessly across different environments. Remember, the key to mastering JavaScript is continuous learning and practical application of concepts.
For further reading on JavaScript modules and their usage, reputable sources like Mozilla Developer Network (MDN) provide comprehensive guides and documentation.
With these insights and tools at your disposal, you're now ready to tackle the "require is not defined" error head-on. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
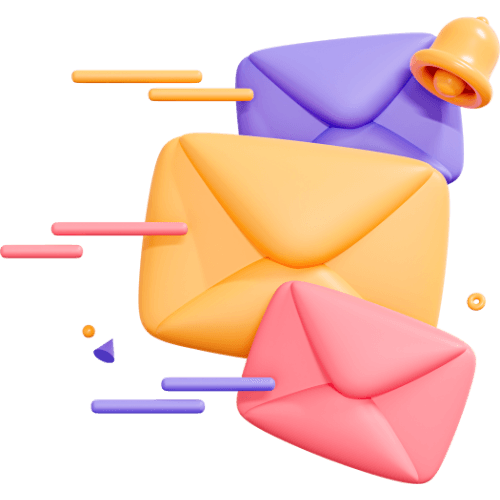
Related articles
9 Articles
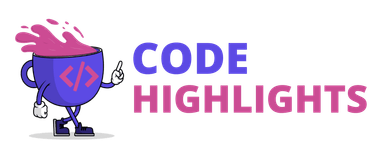
Copyright © Code Highlights 2024.