JavaScript return Statement

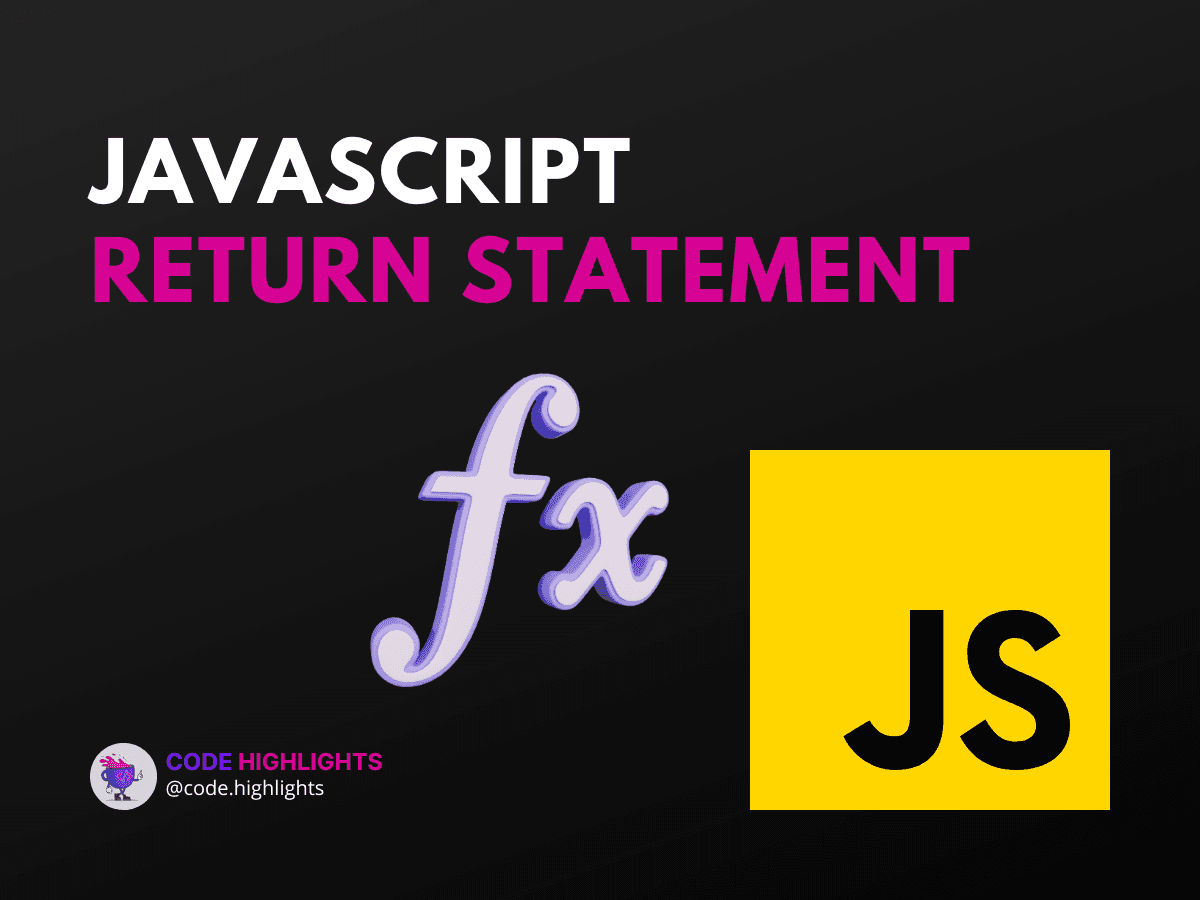
- Understanding the return Keyword in JavaScript
- When to Use return in JavaScript
- Crafting Functions with return
- Example: Calculating the Area of a Circle
- Best Practices and Common Pitfalls
- Conclusion
JavaScript is a powerful language that drives the interactive elements of modern websites. Understanding how to control the flow of information in your code is crucial, and that's where the return
statement comes into play. It's a fundamental concept that can be a bit tricky at first, but once mastered, it opens up a world of possibilities for your functions.
1function exampleFunction() {
2 let result = 'Hello, World!';
3 return result;
4}
5
6console.log(exampleFunction()); // Outputs: Hello, World!
This simple code snippet illustrates the syntax of a return
statement within a JavaScript function. The return
keyword is followed by the value or expression you wish to return from the function. Now, let's dive deeper into the mechanics and best practices of using return
in JavaScript.
Understanding the return
Keyword in JavaScript
When discussing functions in JavaScript, one question often arises: "Was ist Return in Javascript?" Simply put, the return
keyword is used to exit a function and hand back a value to the place where the function was invoked. This feature is not just a convenience but a powerful tool for controlling the flow of data through your code.
When to Use return
in JavaScript
"Wann benutzt man return?" The answer lies in the need for modularity and reusability in coding. You use return
when you want a function to produce a result that can be used elsewhere in your program. Whether you're calculating values, processing data, or simply need to stop the execution of a function under certain conditions, return
is your go-to mechanism.
For those who are just starting out, it might be helpful to check out Learn JavaScript for a more comprehensive understanding of the basics.
Crafting Functions with return
Creating functions that utilize the return
keyword effectively is a skill that will greatly enhance your coding. Here's a step-by-step guide to crafting a function with a return
statement:
- Define the Function: Start by declaring your function with a clear purpose.
- Process Information: Perform the necessary calculations or data processing.
- Return the Result: Use the
return
keyword followed by the value or result you want to pass back.
Example: Calculating the Area of a Circle
1function calculateArea(radius) {
2 const area = Math.PI * radius * radius;
3 return area;
4}
5
6let circleArea = calculateArea(5);
7console.log(circleArea); // Outputs: 78.53981633974483
In this example, we define a function calculateArea
that calculates the area of a circle given its radius and returns the result. This value can then be stored in a variable, displayed on the screen, or used in further calculations.
Best Practices and Common Pitfalls
- Single Exit Point: Aim to have a single
return
statement at the end of the function for better readability and maintainability. - Explicit Returns: Always specify what you're returning. Even if it's
undefined
, make it clear by statingreturn undefined;
. - Avoid Side Effects: A function should ideally not alter any external state unless that's its purpose.
To further expand your web development skills, consider exploring HTML Fundamentals Course and Learn CSS Introduction, which are essential alongside JavaScript for creating interactive and well-styled web pages. If you're new to all this, starting with an Introduction to Web Development might be the right move.
Conclusion
The return
keyword in JavaScript is a cornerstone of function definition, allowing you to control the output and flow of your code effectively. By understanding and implementing return
correctly, you can create more efficient and readable programs. Remember to keep practicing, as mastery comes with experience.
For further reading on JavaScript and programming best practices, check out these external resources:
- Mozilla Developer Network - Functions
- W3Schools JavaScript Functions
- JavaScript.info - Function Basics
With this knowledge in hand, you're well on your way to becoming proficient in JavaScript and writing functions that are both powerful and easy to understand. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
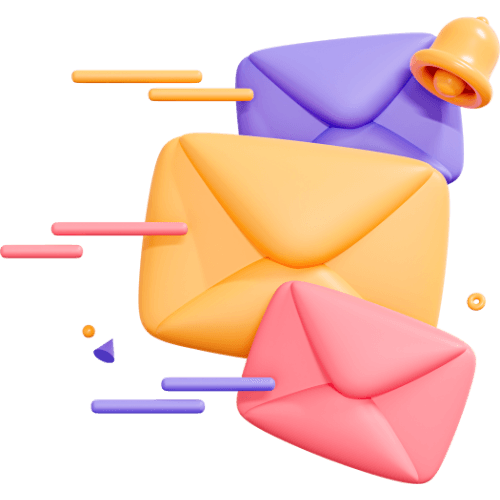
Related articles
9 Articles
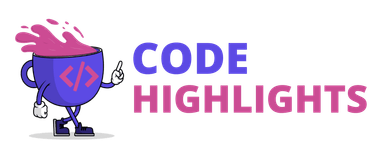
Copyright © Code Highlights 2025.