What is Returning Multiple Values JavaScript? A Quick Overview

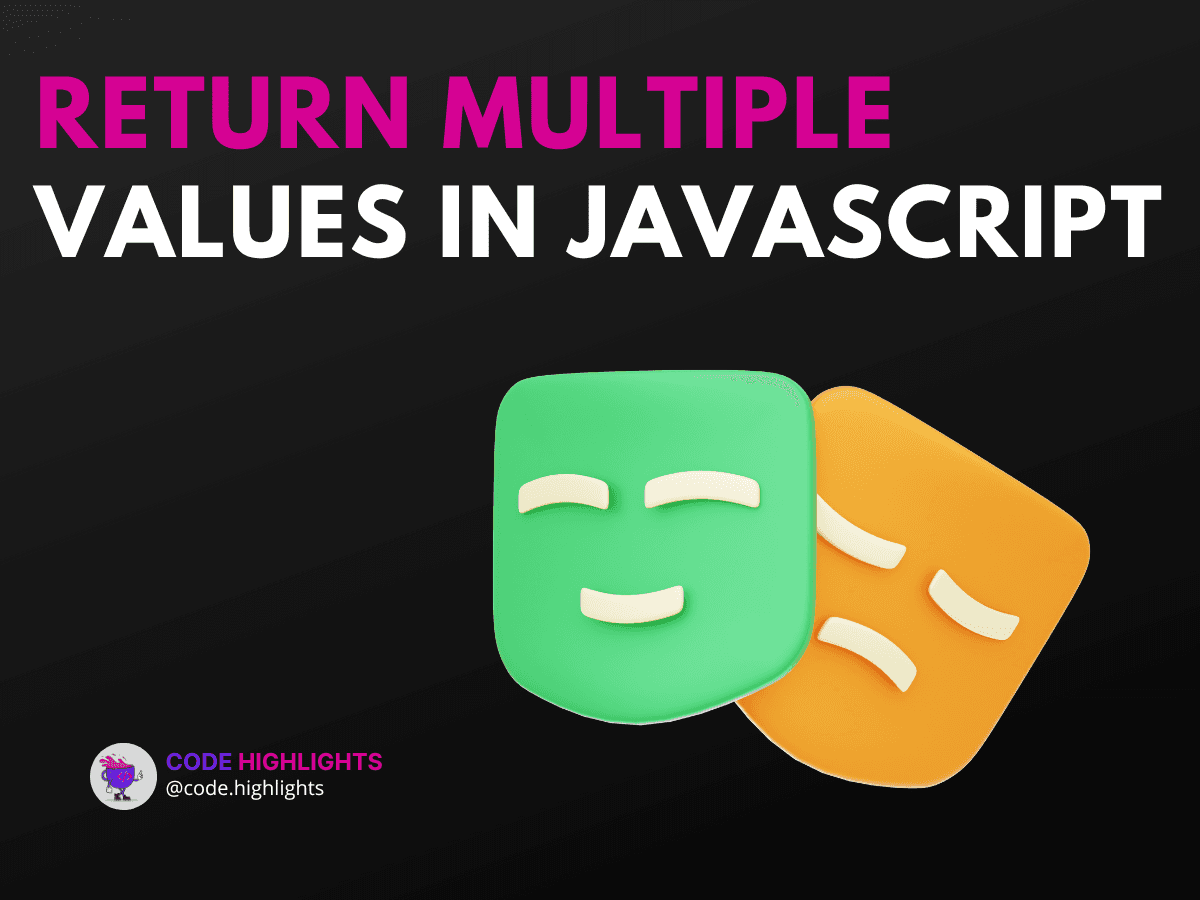
- Syntax for Returning Multiple Values
- Using Arrays
- Using Objects
- Example 1: Returning Coordinates as an Array
- Example 2: Returning User Info as an Object
- Example 3: Combining Functions
- Browser Compatibility
- Summary
Returning multiple values in JavaScript is a useful concept that allows functions to send back more than one piece of! information. While traditional functions return a single value using the return
statement, you can work around this limitation. This capability enhances code flexibility and makes it easier to handle complex data.
Here’s a quick example to illustrate how you can return multiple values:
1function getCoordinates() {
2 return [10, 20];
3}
4
5const [x, y] = getCoordinates();
6console.log(`X: ${x}, Y: ${y}`); // Output: X: 10, Y: 20
In this example, the function getCoordinates
returns an array with two values. You can then use destructuring to assign these values to variables.
Syntax for Returning Multiple Values
To return multiple values in JavaScript, you can use arrays or objects.
Using Arrays
When you return an array, you can pack multiple values into it. Here's a simple breakdown:
-
Syntax:
-
Return Values: An array containing the specified values.
Using Objects
Alternatively, you can return an object to provide named properties, which can enhance clarity.
-
Syntax:
-
Return Values: An object with key-value pairs.
Here’s an example of returning an object:
1function getUserData() {
2 return { name: "Alice", age: 25 };
3}
4
5const user = getUserData();
6console.log(`Name: ${user.name}, Age: ${user.age}`); // Output: Name: Alice, Age: 25
Example 1: Returning Coordinates as an Array
In this example, we'll return coordinates using an array.
1function getPoint() {
2 return [5, 15];
3}
4
5const point = getPoint();
6console.log(`Point: (${point[0]}, ${point[1]})`); // Output: Point: (5, 15)
Example 2: Returning User Info as an Object
This example shows how to return user details in an object format.
1function createUser(name, age) {
2 return { name: name, age: age };
3}
4
5const userInfo = createUser("Bob", 30);
6console.log(`User: ${userInfo.name}, Age: ${userInfo.age}`); // Output: User: Bob, Age: 30
Example 3: Combining Functions
You can also combine functions to return multiple values.
1function calculate(a, b) {
2 return [a + b, a - b];
3}
4
5const [sum, difference] = calculate(10, 5);
6console.log(`Sum: ${sum}, Difference: ${difference}`); // Output: Sum: 15, Difference: 5
Browser Compatibility
The syntax for returning multiple values using arrays and objects is widely supported across modern web browsers such as Chrome, Firefox, Safari, and Edge. You should not encounter any compatibility issues when using these methods in your web applications.
Summary
In summary, returning multiple values in JavaScript can be achieved effortlessly by using arrays or objects. This technique simplifies data handling and enhances the readability of your code. Whether you are developing small scripts or large applications, understanding how to return multiple values is crucial. For further learning, consider exploring more about JavaScript through our JavaScript Course.
By mastering this concept, you can improve your coding skills and make your functions more versatile. If you're keen on web development, check out our courses on HTML Fundamentals and CSS Introduction to broaden your knowledge.
For more resources on web development, visit our Introduction to Web Development page. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
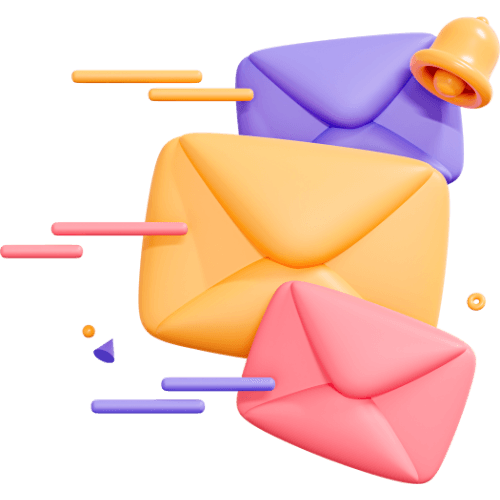
Related articles
9 Articles
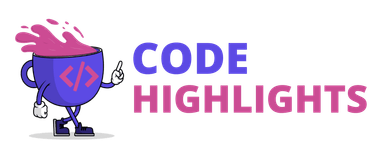
Copyright © Code Highlights 2025.