How to Use Safe Navigation Operator JavaScript for Cleaner Code

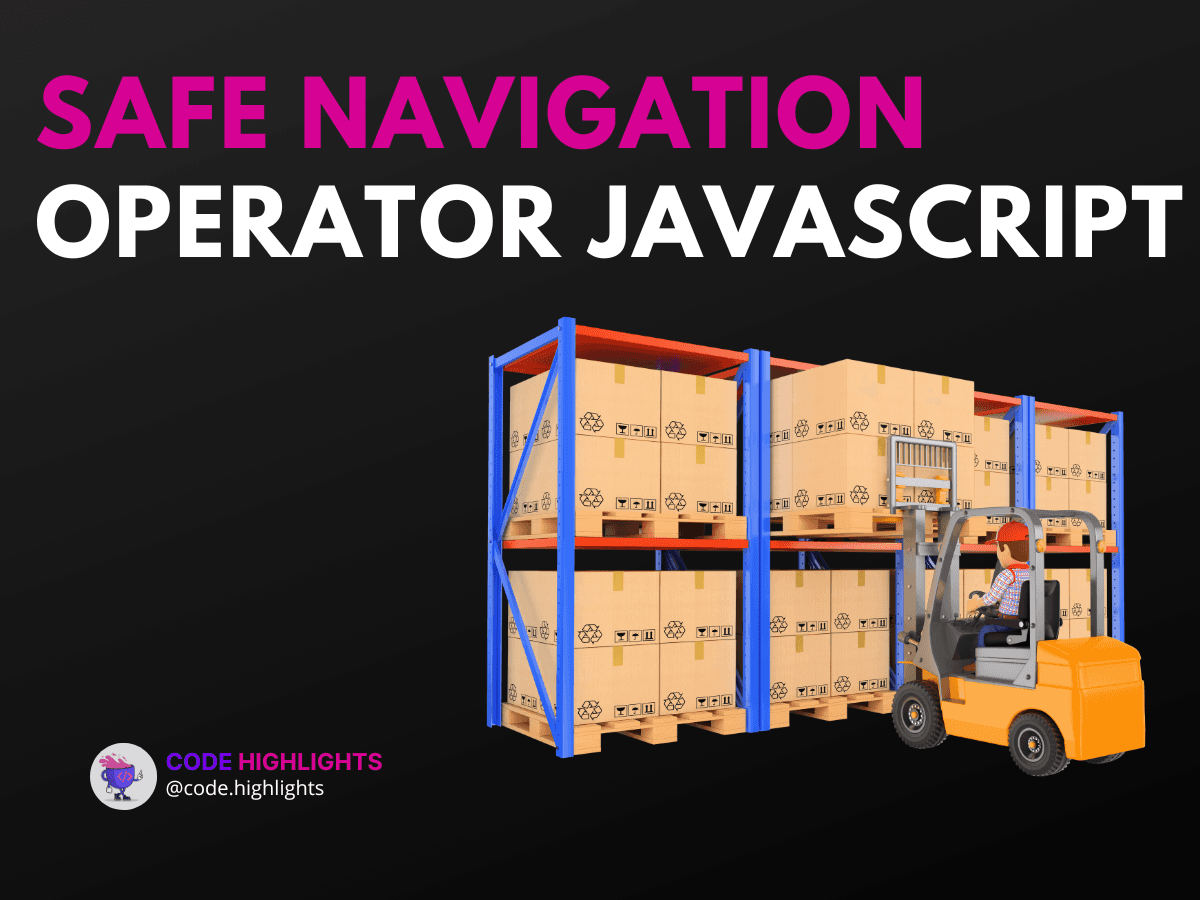
- Quick Introduction to the Safe Navigation Operator
- Understanding the Syntax
- Parameters
- Return Values
- Variations
- Example 1: Accessing Nested Properties
- Safely Accessing Deeply Nested Properties
- Example 2: Using Safe Navigation with Functions
- Calling Methods Safely
- Example 3: Safe Navigation in Angular
- Using Safe Navigation in Angular Templates
- Compatibility with Major Web Browsers
- Summary
The safe navigation operator is a handy feature in JavaScript that helps you avoid errors when accessing properties of objects. It allows you to safely access deeply nested properties without worrying about whether each level exists. This operator can make your code cleaner and reduce the chances of runtime errors. Let’s dive into how it works!
Quick Introduction to the Safe Navigation Operator
Here’s a simple example to get you started:
In this snippet, we use ?.
to safely access the name
property. If profile
doesn’t exist, userName
will be undefined
instead of throwing an error.
Understanding the Syntax
The syntax for the safe navigation operator is straightforward. You use ?.
before the property you want to access. Here’s how it looks:
Parameters
- object: The object you want to check for properties.
- property: The property you want to access.
Return Values
- Returns the value of the property if it exists.
- Returns
undefined
if the object or property does not exist.
Variations
You can also use it with methods:
If object
is null
or undefined
, result
will also be undefined
.
Example 1: Accessing Nested Properties
Safely Accessing Deeply Nested Properties
Imagine you have a user object:
1const user = {
2 profile: {
3 contact: {
4 email: "alice@example.com"
5 }
6 }
7};
8
9const email = user.profile?.contact?.email; // "alice@example.com"
If contact
were missing, email
would simply be undefined
, avoiding errors.
Example 2: Using Safe Navigation with Functions
Calling Methods Safely
You can also use the safe navigation operator when calling methods on objects:
1const car = {
2 engine: {
3 start: () => "Engine started!"
4 }
5};
6
7const startMessage = car.engine?.start(); // "Engine started!"
If engine
didn’t exist, startMessage
would be undefined
.
Example 3: Safe Navigation in Angular
Using Safe Navigation in Angular Templates
In Angular, you can use the safe navigation operator in your templates:
1<div>{{ user?.profile?.name }}</div>
This prevents errors if user
or profile
is not defined, displaying nothing instead.
Compatibility with Major Web Browsers
The safe navigation operator is supported in most modern browsers, including Chrome, Firefox, Safari, and Edge. However, it’s always good to check compatibility if you’re supporting older versions. You can refer to MDN Web Docs for more details.
Summary
In this tutorial, we explored the safe navigation operator in JavaScript. We learned how it helps us access nested properties safely, reducing errors in our code. By using ?.
, we can write cleaner and more reliable JavaScript. Whether you’re building complex applications or simple scripts, understanding this operator is essential.
For further learning, consider checking out these resources: Learn JavaScript, HTML Fundamentals Course, and Introduction to Web Development.
Now that you know how to use the safe navigation operator in JavaScript, you can apply it to your projects for improved code quality!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
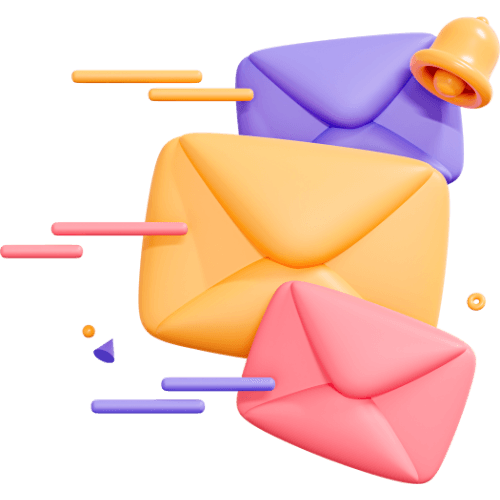
Related articles
9 Articles
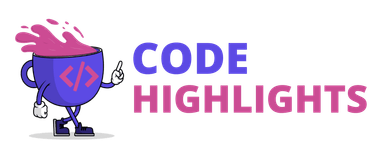
Copyright © Code Highlights 2025.