5 Key Insights on Scope Chain in JavaScript for Better Coding

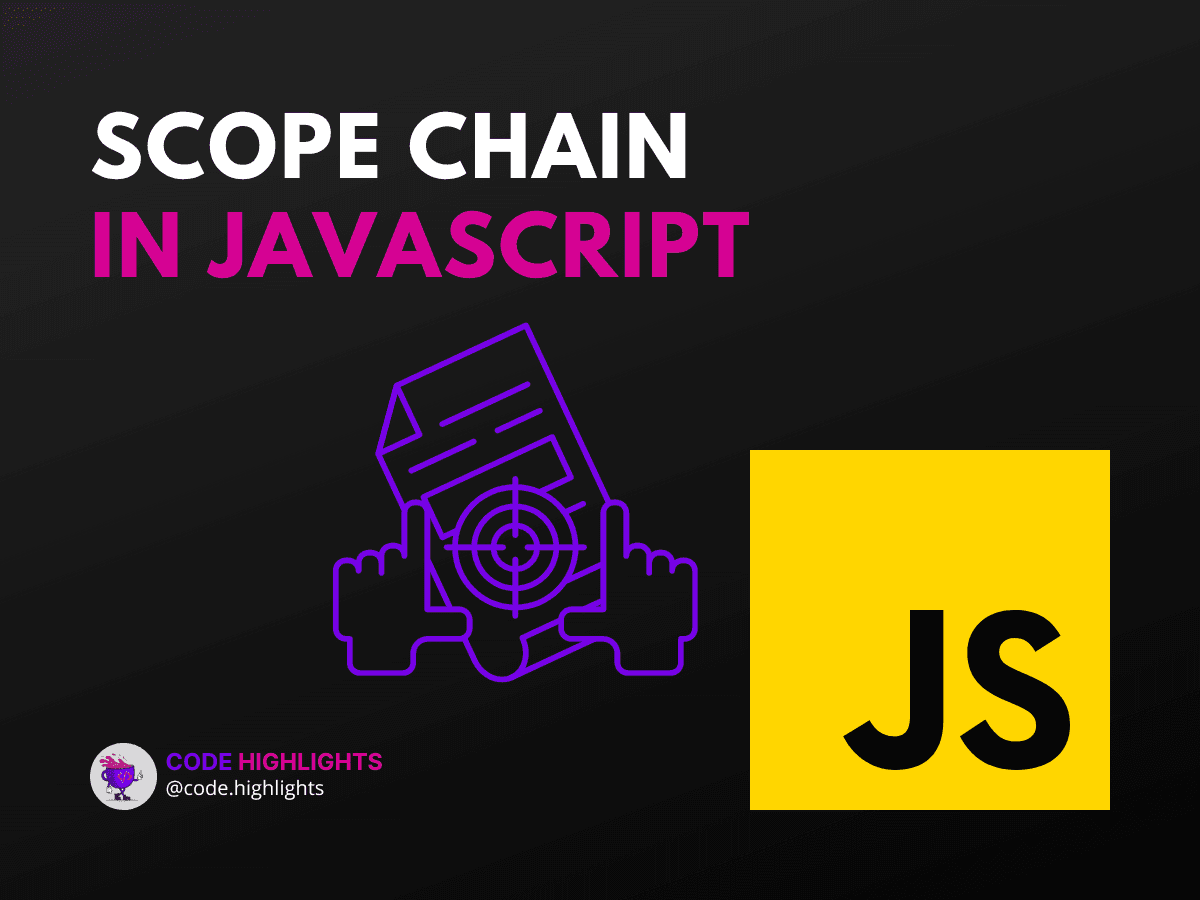
- What is Scope in JavaScript?
- Understanding Lexical Scoping and the Scope Chain
- Different Types of Scoping in JavaScript
- Scope in ES6
- Practical Examples and External Insights
JavaScript is a powerful language that runs in browsers and on servers, and understanding its inner workings can significantly improve your coding skills. One fundamental concept that often puzzles developers is the scope chain
. To kick things off, let's look at a snippet of JavaScript code:
1function outerFunction() {
2 var outerVar = 'I am from the outer scope!';
3
4 function innerFunction() {
5 console.log(outerVar);
6 }
7
8 return innerFunction;
9}
10
11var myInnerFunction = outerFunction();
12myInnerFunction(); // Outputs: I am from the outer scope!
This introductory code example illustrates how an inner function can access variables from an outer function, thanks to the scope chain in JavaScript.
What is Scope in JavaScript?
In JavaScript, scope refers to the current context of code, which determines the accessibility of variables to JavaScript entities like functions. Simply put, it answers the question: "Where are these variables available for use?" Scope is crucial because it ensures that variables are only accessible within the region they are defined, providing a structured and secure way to write programs.
Understanding Lexical Scoping and the Scope Chain
Lexical scoping, also known as static scoping, is a convention that sets the scope of a variable so that it is determined by its location within the source code. It's the main type of scoping in JavaScript, where blocks of code create scopes that are nested within each other. The scope chain is the system that the JavaScript interpreter uses to resolve variable references. When a variable is used, JavaScript looks up the scope chain, moving outward from the innermost scope until it finds the variable in question.
If you're looking to deepen your understanding of JavaScript's fundamentals, consider diving into this comprehensive course on JavaScript.
Different Types of Scoping in JavaScript
JavaScript primarily uses lexical scoping, but there are different types of scoping within this model:
- Global Scope: Variables declared outside all functions or curly braces
{}
belong to the global scope and are accessible from anywhere in the code. - Local (or Function) Scope: Variables declared within a function are local to that function and cannot be accessed from outside.
- Block Scope: Introduced with ES6,
let
andconst
provide block-level scoping, where variables are scoped to the nearest set of curly braces.
For those new to these concepts, taking an HTML fundamentals course can help you understand how JavaScript interacts with web page structure.
Scope in ES6
ES6, or ECMAScript 2015, brought significant improvements to scoping in JavaScript with the introduction of let
and const
. Unlike var
, which is function-scoped, both let
and const
are block-scoped. This means they are confined to the block (denoted by curly braces) in which they are defined, giving developers more control and reducing the risk of errors due to variable name collisions.
To get a well-rounded education in web development, including the latest ES6 features, consider enrolling in an introduction to web development course.
Practical Examples and External Insights
Now that we've covered the basics, let's look at some practical examples and insights from reputable sources:
- Mozilla Developer Network (MDN) provides an in-depth guide on JavaScript variables and scope.
- For a deeper dive into ES6 features, this article from the ECMA International outlines the specification.
- If you're interested in exploring more about function scope and block scope, this tutorial from freeCodeCamp is a great resource.
Remember, understanding the scope chain in JavaScript is essential for writing clean, efficient, and error-free code. By mastering these concepts, you'll be well on your way to becoming a more proficient JavaScript developer.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
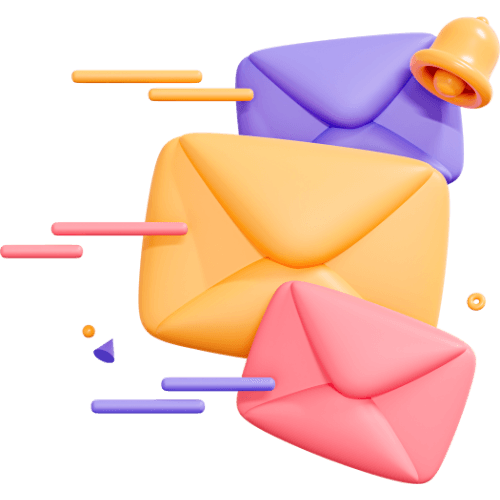
Related articles
9 Articles
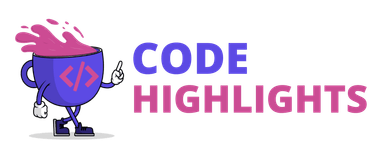
Copyright © Code Highlights 2025.